inotify监控文件,并在收到文件变化通知后调用回调,C代码示例
时间: 2024-09-19 11:07:55 浏览: 57
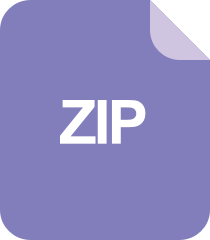
nimwatch:Nim的文件系统监视库
Inotify是一个Linux内核功能,用于监控文件系统活动,当文件、目录或元数据发生改变时会发送相应的事件通知。在C语言中,你可以使用inotify库(如libinotify)来实现在文件变化时调用预定义的回调函数。
下面是一个简单的C代码示例,展示了如何使用libinotify创建一个监控目录并处理事件的基本流程:
```c
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <sys/inotify.h>
#define MAX_EVENTS 1024
void handle_event(int fd, uint32_t mask, const char* path) {
printf("Event received for %s: %s\n", path, event_to_string(mask));
// 在这里添加具体的业务逻辑,例如处理文件变动、更新数据库等
}
int main() {
int wd = -1;
int fd = inotify_init();
if (fd == -1) {
perror("Error initializing inotify");
return 1;
}
struct inotify_add_watch add_watch_request = {fd, ".", IN_CLOSE_WRITE | IN_MOVED_TO};
if (inotify_add_watch(fd, &add_watch_request) == -1) {
perror("Error adding watch");
close(fd);
return 1;
}
while (true) {
char buffer[MAX_EVENTS * sizeof(struct inotify_event)];
ssize_t n = read(fd, buffer, sizeof(buffer));
if (n <= 0) {
perror("Error reading from inotify");
break;
}
for (size_t i = 0; i < n / sizeof(struct inotify_event); ++i) {
struct inotify_event* event = (struct inotify_event*)&buffer[i];
if (event->mask & ADD watches) {
wd = event->wd;
handle_event(fd, event->mask, event->name);
} else {
handle_event(fd, event->mask, event->name);
}
}
}
close(fd);
return 0;
}
```
在这个例子中,我们首先初始化inotify,然后添加对当前目录的监控,只关心IN_CLOSE_WRITE和IN_MOVED_TO事件。`handle_event`函数会在接收到事件时被调用,并根据事件类型处理文件的变化。
阅读全文
相关推荐
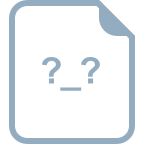
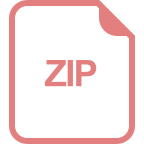

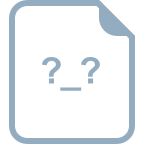
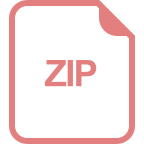
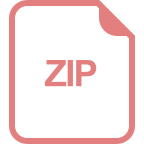
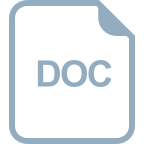
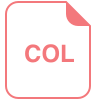
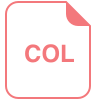
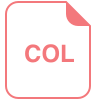
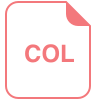
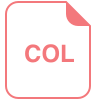
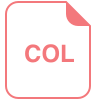
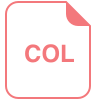
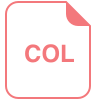
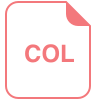
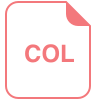
