kotlin图片旋转
时间: 2023-12-22 19:00:08 浏览: 34
当在Kotlin中要实现图片全屏时,可以使用以下步骤:
1. 首先,确保你的图片资源是高分辨率的,并且具有足够的大小以适应各种屏幕尺寸。
2. 在布局文件中,使用ImageView来显示图片。设置ImageView的宽度和高度为match_parent,以充满整个屏幕。
3. 如果你想要适应屏幕的刘海区域(如果有的话),可以使用属性android:layoutInDisplayCutoutMode="shortEdges"。这样,在竖屏模式和横屏模式下,内容都会呈现到刘海区域中。
4. 在代码中,可以使用BitmapFactory类来加载位图。确保位图的质量和大小适合全屏显示。
5. 如果您在改变尺寸的视图中使用位图作为背景,您可能会注意到Android会缩放您的图片,导致模糊或缩放失真。为了解决这个问题,您可以使用九宫格位图,它是一种特殊格式的PNG文件,可以指示哪些区域可以拉伸,哪些区域不可以拉伸。这样可以确保图片在不同屏幕尺寸上正确缩放而不失真。
通过遵循以上步骤,您可以在Kotlin中实现图片全屏显示,并确保在不同屏幕尺寸上正确缩放。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
相关问题
kotlin 图片 sqlite
要在 Kotlin 中将图像插入 SQLite 数据库,可以将图像转换为字节数组,然后将其存储在 SQLite 数据库中的 BLOB 列中。以下是一个示例:
```kotlin
// 将图像转换为字节数组
val bitmap = BitmapFactory.decodeResource(resources, R.drawable.my_image)
val stream = ByteArrayOutputStream()
bitmap.compress(Bitmap.CompressFormat.PNG, 100, stream)
val byteArray = stream.toByteArray()
// 将字节数组插入 SQLite 数据库
val database = MyDatabaseHelper(context).writableDatabase
val contentValues = ContentValues()
contentValues.put("image_column", byteArray)
database.insert("my_table", null, contentValues)
```
要从 SQLite 数据库中检索图像,可以使用以下代码:
```kotlin
// 从 SQLite 数据库中检索图像
val cursor = database.query("my_table", arrayOf("image_column"), null, null, null, null, null)
if (cursor.moveToFirst()) {
val byteArray = cursor.getBlob(cursor.getColumnIndex("image_column"))
val bitmap = BitmapFactory.decodeByteArray(byteArray, 0, byteArray.size)
imageView.setImageBitmap(bitmap)
}
cursor.close()
```
这将检索数据库中名为 "my_table" 的表中的第一行,并将其图像字节数组转换为位图,并在 ImageView 中显示它。
kotlin 图片预览
您可以使用 Android 系统自带的 ImageView 控件来实现图片预览,具体步骤如下:
1. 在布局文件中添加 ImageView 控件:
```
<ImageView
android:id="@+id/imageView"
android:layout_width="match_parent"
android:layout_height="match_parent" />
```
2. 在代码中获取 ImageView 控件并设置图片:
```
val imageView = findViewById<ImageView>(R.id.imageView)
val bitmap = BitmapFactory.decodeFile("图片路径")
imageView.setImageBitmap(bitmap)
```
其中,"图片路径" 是您要预览的图片的路径。
相关推荐
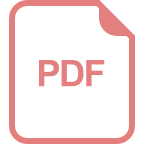
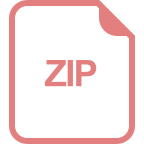
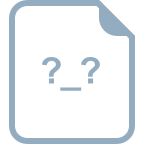












