python if else finally
时间: 2024-01-24 10:15:28 浏览: 29
在Python中,if-else语句用于根据条件执行不同的代码块。finally子句用于定义无论是否发生异常都会执行的代码块。下面是一个示例:
```python
try:
# 代码块
age = int(input('请输入你的年龄:'))
if age >= 18:
print('你已经成年了')
else:
print('你还没有成年')
except:
# 异常处理代码块
print('发生了异常')
finally:
# 无论是否发生异常都会执行的代码块
print('程序执行完毕')
```
在上面的示例中,我们使用try-except语句来捕获可能发生的异常。如果用户输入的不是一个整数,会触发异常并执行except代码块中的代码。无论是否发生异常,finally代码块中的代码都会执行。
相关问题
python 中的if else finally语法结构
在 Python 中,if-else-finally 是一种条件语句结构,用于根据条件执行不同的代码块。if-else-finally 语法结构如下:
```
if condition:
# 如果条件为真,执行这个代码块
else:
# 如果条件为假,执行这个代码块
finally:
# 不管条件是否为真,都会执行这个代码块
```
其中 `condition` 是待测试的条件,可以是任何能够返回 True 或 False 的表达式。如果条件为真,将执行 `if` 代码块;否则,将执行 `else` 代码块。无论条件是否为真,都会执行 `finally` 代码块。
需要注意的是,如果 `if` 代码块只有一行语句,可以写成一行,如下所示:
```
if condition: statement
```
如果 `else` 或 `finally` 代码块只有一行语句,也可以写成一行。
python if
In Python, `if` is a conditional statement that allows you to execute a block of code only if a certain condition is met. Here's the basic syntax of an `if` statement:
```python
if condition:
# code to execute if condition is True
```
The `condition` can be any expression that evaluates to a Boolean value (`True` or `False`). If the `condition` is `True`, the code block indented under the `if` statement will be executed. If the `condition` is `False`, the code block will be skipped and execution will continue with the next statement after the `if` block.
You can also use an `else` statement to specify a block of code to execute if the `condition` is `False`:
```python
if condition:
# code to execute if condition is True
else:
# code to execute if condition is False
```
Finally, you can use an `elif` statement (short for "else if") to specify additional conditions to check:
```python
if condition1:
# code to execute if condition1 is True
elif condition2:
# code to execute if condition2 is True and condition1 is False
else:
# code to execute if both condition1 and condition2 are False
```
Here's an example to help illustrate how `if` statements work:
```python
x = 5
if x > 0:
print("x is positive")
else:
print("x is non-positive")
```
In this example, the `condition` is `x > 0`. Since `x` is equal to `5`, which is greater than `0`, the code block under the `if` statement will be executed, and the output will be:
```
x is positive
```
I hope this helps! Let me know if you have any other questions.
相关推荐
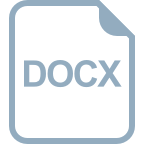
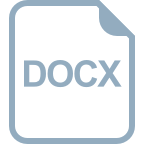
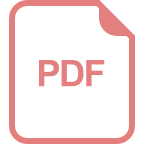












