java中如何将Excel表格中的已有行和列的边框设置为所有框线
时间: 2024-01-09 12:05:34 浏览: 252
要在 Java 中将 Excel 表格中已有行和列的边框设置为所有框线,可以使用 Apache POI 库。以下是一个示例代码:
```java
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import java.io.FileInputStream;
import java.io.FileOutputStream;
public class ExcelBorderExample {
public static void main(String[] args) {
try {
// 读取现有的 Excel 文件
FileInputStream file = new FileInputStream("example.xlsx");
Workbook workbook = new XSSFWorkbook(file);
Sheet sheet = workbook.getSheetAt(0);
// 创建样式
CellStyle borderStyle = workbook.createCellStyle();
borderStyle.setBorderTop(BorderStyle.THIN);
borderStyle.setBorderBottom(BorderStyle.THIN);
borderStyle.setBorderLeft(BorderStyle.THIN);
borderStyle.setBorderRight(BorderStyle.THIN);
// 设置已有行的边框样式
for (Row row : sheet) {
for (Cell cell : row) {
cell.setCellStyle(borderStyle);
}
}
// 设置已有列的边框样式
for (int colIndex = 0; colIndex < sheet.getRow(0).getLastCellNum(); colIndex++) {
for (Row row : sheet) {
Cell cell = row.getCell(colIndex);
if (cell != null) {
cell.setCellStyle(borderStyle);
}
}
}
// 保存文件
FileOutputStream fileOut = new FileOutputStream("example.xlsx");
workbook.write(fileOut);
fileOut.close();
System.out.println("已成功设置边框样式!");
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
这段代码使用 Apache POI 库打开一个现有的 Excel 文件,然后遍历每个单元格并为其设置边框样式。首先,通过 `workbook.getSheetAt(0)` 获取第一个工作表。然后,使用嵌套的循环分别遍历所有的行和列,并为每个单元格设置样式。最后,将文件保存并关闭。这样就可以将已有行和列的边框设置为所有框线。
阅读全文
相关推荐
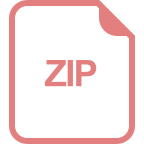
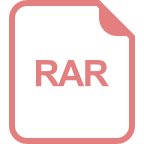
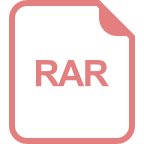



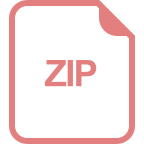
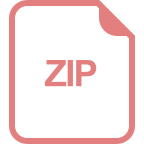
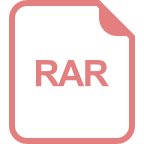
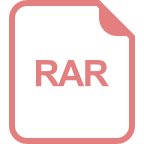
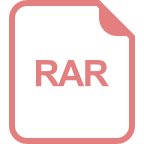
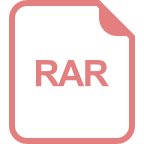
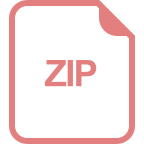
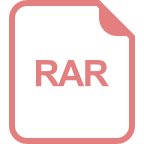
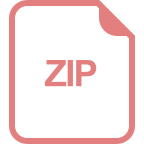
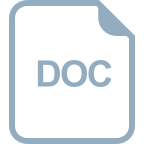
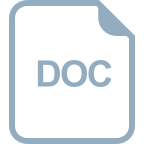
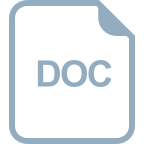
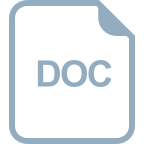