打开深度摄像头并部署yolov5的python代码
时间: 2023-09-21 09:01:35 浏览: 188
要打开深度摄像头并部署Yolov5的Python代码,可以按照以下步骤进行:
首先,安装所需的依赖库和软件。可以使用以下命令在终端中安装所需的依赖库和软件:
```
pip install numpy tensorflow opencv-python-headless torch torchvision pyyaml
```
接下来,导入所需的Python库:
```python
import cv2
import torch
import numpy as np
from PIL import Image
from models.experimental import attempt_load
from utils.datasets import letterbox
from utils.general import non_max_suppression, scale_coords
from utils.plots import plot_one_box
from utils.torch_utils import select_device
```
然后,定义一些全局变量和函数:
```python
device = select_device('')
def load_model(weights):
model = attempt_load(weights, map_location=device)
return model
def preprocess_image(img):
img = letterbox(img, new_shape=640)[0]
img = img[:, :, ::-1].transpose(2, 0, 1)
img = np.ascontiguousarray(img)
return img
def postprocess_bboxes(outputs, img, conf_thres=0.3, iou_thres=0.45):
detections = non_max_suppression(outputs, conf_thres, iou_thres)[0]
if len(detections):
detections[:, :4] = scale_coords(img.shape[2:], detections[:, :4], img.shape).round()
return detections
```
接下来,加载Yolov5的预训练权重文件:
```python
weights = 'path/to/weights.pt'
model = load_model(weights).to(device).eval()
```
然后,打开深度摄像头:
```python
cap = cv2.VideoCapture(0)
```
在循环中,逐帧读取摄像头图像并进行目标检测:
```python
while True:
ret, frame = cap.read()
if not ret:
break
img = preprocess_image(frame)
img = torch.from_numpy(img).unsqueeze(0).to(device)
outputs = model(img)[0]
detections = postprocess_bboxes(outputs, frame)
for x1, y1, x2, y2, conf, cls in detections:
plot_one_box((x1, y1, x2, y2), frame, label=cls, color=(0, 255, 0))
cv2.imshow("Yolov5 Object Detection", frame)
if cv2.waitKey(1) == ord('q'):
break
cap.release()
cv2.destroyAllWindows()
```
最后,释放资源并关闭窗口。
这就是使用Python代码打开深度摄像头并部署Yolov5的步骤。
阅读全文
相关推荐
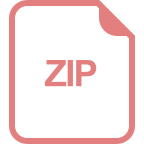
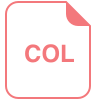
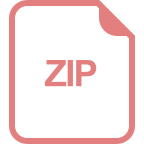
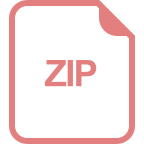
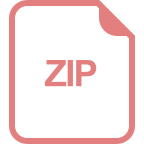
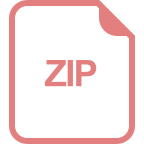
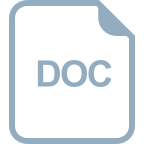
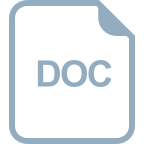
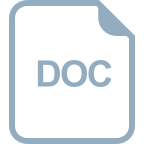
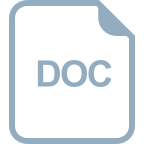
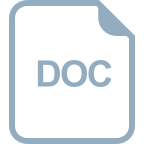
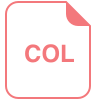



