def next_letter(letter): if letter == 'Z' or letter == 'z': return 'a' return chr(ord(letter) + 1).lower() def previous_letter(letter): if letter == 'A' or letter == 'a': return 'z' return chr(ord(letter) - 1).lower() # 加密 def encrypt(): encrypt_letter = '' for i in need_encrypt: if i.isalpha(): ii = next_letter(i) if i.upper() == i: encrypt_letter += ii else: encrypt_letter += ii.upper() elif i.isdigit(): if i == '9': encrypt_letter += '0' else: encrypt_letter += str(int(i)+1) else: encrypt_letter += i print(encrypt_letter) # 解密 def decode(): decode_letter = '' for i in need_decode: if i.isalpha(): ii = previous_letter(i) if i.upper() == i: decode_letter += ii else: decode_letter += ii.upper() elif i.isdigit(): if i == '0': decode_letter += '9' else: decode_letter += str(int(i)-1) else: decode_letter += i print(decode_letter) while 1: try: need_encrypt = input() need_decode = input() encrypt() decode() except: break
时间: 2024-02-14 10:29:26 浏览: 31
这段代码是一个简单的加密和解密程序。它包含两个函数:`next_letter`和`previous_letter`,用于获取字母的下一个和上一个字母。然后有两个主要的功能函数:`encrypt`和`decode`,用于加密和解密给定的字符串。
在加密过程中,程序会遍历输入字符串中的每个字符。如果字符是字母,则使用`next_letter`函数获取它的下一个字母,并根据原字符的大小写情况决定是否转为大写。如果字符是数字,则将其加1,如果是9则变为0。其他非字母和数字的字符将保持不变。
在解密过程中,程序会遍历输入字符串中的每个字符。如果字符是字母,则使用`previous_letter`函数获取它的上一个字母,并根据原字符的大小写情况决定是否转为大写。如果字符是数字,则将其减1,如果是0则变为9。其他非字母和数字的字符将保持不变。
代码最后使用一个无限循环来获取用户输入,并对输入进行加密和解密操作。当用户输入异常时,循环终止。
请问你有什么问题?
相关问题
def encrypt(plain_text, shift): encrypted_text = "" for char in plain_text: if char.isalpha(): if char.isupper(): encrypted_text += chr((ord(char) - 65 + shift) % 26 + 65) else: encrypted_text += chr((ord(char) - 97 + shift) % 26 + 97) else: encrypted_text += char return encrypted_text def decrypt(encrypted_text, shift): decrypted_text = "" for char in encrypted_text: if char.isalpha(): if char.isupper(): decrypted_text += chr((ord(char) - 65 - shift) % 26 + 65) else: decrypted_text += chr((ord(char) - 97 - shift) % 26 + 97) else: decrypted_text += char return decrypted_text # 代码输入和输出部分 text = input("输入想要加密或解密的文本:") shift = int(input("输入加密的数位:")) encrypted = encrypt(text, shift) print("加密后的文本:", encrypted) decrypted = decrypt(encrypted, shift) print("解密后的文本:", decrypted)
这是一个改进后的程序,它允许用户输入要加密或解密的文本,并指定加密的位移。
```python
def encrypt(plain_text, shift):
encrypted_text = ""
for char in plain_text:
if char.isalpha():
if char.isupper():
encrypted_text += chr((ord(char) - 65 + shift) % 26 + 65)
else:
encrypted_text += chr((ord(char) - 97 + shift) % 26 + 97)
else:
encrypted_text += char
return encrypted_text
def decrypt(encrypted_text, shift):
decrypted_text = ""
for char in encrypted_text:
if char.isalpha():
if char.isupper():
decrypted_text += chr((ord(char) - 65 - shift) % 26 + 65)
else:
decrypted_text += chr((ord(char) - 97 - shift) % 26 + 97)
else:
decrypted_text += char
return decrypted_text
# 代码输入和输出部分
text = input("输入想要加密或解密的文本:")
shift = int(input("输入加密的数位:"))
encrypted = encrypt(text, shift)
print("加密后的文本:", encrypted)
decrypted = decrypt(encrypted, shift)
print("解密后的文本:", decrypted)
```
现在,您可以输入要加密或解密的文本,并指定一个数字作为加密的位移。程序将输出加密后的文本以及解密后的文本。
def strQ2B(ustring): rstring = "" for uchar in ustring: inside_code = ord(uchar) if inside_code == 12288: inside_code = 32 elif 65281 <= inside_code <= 65374: inside_code -= 65248 rstring += chr(inside_code) return rstring
这段代码的功能是将字符串中的全角字符转换为半角字符。全角字符是指Unicode编码值大于等于65281的字符,半角字符是指Unicode编码值小于等于126的字符。在转换过程中,将全角空格(Unicode编码值为12288)转换为半角空格(Unicode编码值为32),将其他全角字符的Unicode编码值减去65248,得到对应的半角字符的Unicode编码值。最后将转换后的字符拼接成新的字符串并返回。
相关推荐
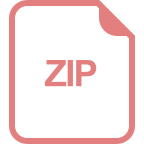
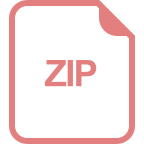
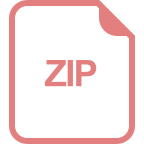













