编写一个 C++ 程序来模拟两台计算机(我们称之为计算机 A 和计算机 B)进行石头剪刀布的游戏
时间: 2024-09-20 16:08:47 浏览: 16
要编写一个简单的 C++ 程序来模拟两个玩家(计算机A和计算机B)玩石头、剪刀、布游戏,首先需要定义一个包含三种选择的枚举类型,以及随机生成计算机选择的函数。以下是简化的示例代码:
```cpp
#include <iostream>
#include <ctime>
#include <cstdlib> // 包含 rand() 和 srand()
// 定义石头、剪刀和布
enum class Move {
Rock,
Paper,
Scissors
};
// 计算机随机选择一个动作
Move computerChoice() {
std::srand(std::time(0)); // 设置随机种子
return (Move)(std::rand() % 3); // 0, 1 或 2 对应于 Rock, Paper, Scissors
}
// 判断胜负
bool compareMoves(Move moveA, Move moveB) {
if (moveA == moveB)
return false; // 平局
else if ((moveA == Move::Rock && moveB == Move::Scissors) ||
(moveA == Move::Paper && moveB == Move::Rock) ||
(moveA == Move::Scissors && moveB == Move::Paper))
return true; // 计算机赢
else
return false; // 用户赢
}
int main() {
const char* playerNames[] = {"计算机A", "计算机B"};
for (size_t i = 0; i < sizeof(playerNames) / sizeof(playerNames[0]); ++i) {
std::cout << "轮到 " << playerNames[i] << ", 输入你的选择(R - 石头,P - 剪刀,S - 布): ";
char userChoice;
std::cin >> userChoice;
switch (userChoice) {
case 'R':
userChoice = Move::Rock;
break;
case 'P':
userChoice = Move::Paper;
break;
case 'S':
userChoice = Move::Scissors;
break;
default:
std::cerr << "无效的选择!" << std::endl;
continue;
}
Move compChoice = computerChoice();
bool isWin = compareMoves(userChoice, compChoice);
if (isWin)
std::cout << playerNames[i] << " 赢了!" << std::endl;
else
std::cout << playerNames[i] << " 输了!" << std::endl;
}
return 0;
}
```
相关推荐
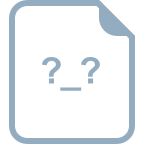
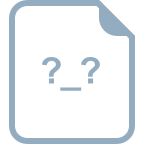














