Java代码实现根据经纬度差值1000m算出最靠近的四条数据
时间: 2024-09-17 18:06:27 浏览: 44
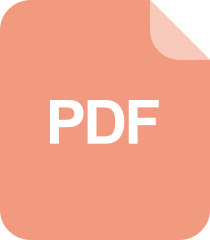
java 根据经纬度获取地址实现代码
在Java中,根据经纬度差值1000米找出最靠近的数据通常需要地理编码和距离计算。首先,你需要将经纬度转换成点(Point)或者地理位置对象,然后利用Haversine公式(大圆距离公式)计算两点之间的精确距离。下面是一个简单的示例,假设你有一个包含经纬度的List<GeoLocation>,GeoLocation是一个自定义类包含经纬度字段:
```java
import java.util.ArrayList;
import java.util.List;
public class Main {
private static final int R = 6371; // 地球平均半径,单位为公里
public static List<GeoLocation> findNearest(List<GeoLocation> locations, double latitude, double longitude, double distanceInMeter) {
List<GeoLocation> nearest = new ArrayList<>();
for (GeoLocation location : locations) {
double latDiff = Math.toRadians(location.getLatitude() - latitude);
double lonDiff = Math.toRadians(location.getLongitude() - longitude);
double a = Math.sin(latDiff / 2) * Math.sin(latDiff / 2)
+ Math.cos(Math.toRadians(latitude)) * Math.cos(Math.toRadians(location.getLatitude()))
* Math.sin(lonDiff / 2) * Math.sin(lonDiff / 2);
double c = 2 * Math.atan2(Math.sqrt(a), Math.sqrt(1 - a));
double distance = R * c; // 计算距离,单位为公里
distance *= 1000; // 转换为米
if (distance <= distanceInMeter && !nearest.contains(location)) {
nearest.add(location); // 当距离小于阈值且地点未添加到结果中时,加入
if (nearest.size() == 4) { // 如果找到四个符合条件的地点就停止搜索
break;
}
}
}
return nearest;
}
public static void main(String[] args) {
GeoLocation location1 = new GeoLocation(39.9042, 116.4074); // 北京坐标
List<GeoLocation> locations = ... // 你的地点列表
List<GeoLocation> result = findNearest(locations, 39.9189, 116.4318, 1000);
System.out.println(result);
}
}
class GeoLocation {
private double latitude;
private double longitude;
// 构造函数、getter和setter省略
}
```
阅读全文
相关推荐

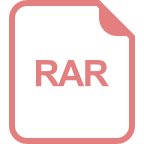
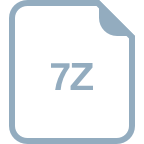
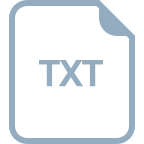
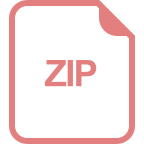
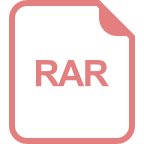
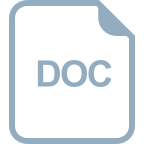
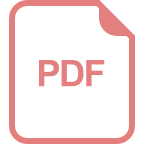
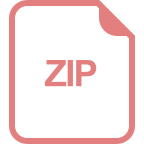
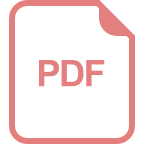