c语言实现sha1算法
时间: 2024-11-07 22:10:07 浏览: 21
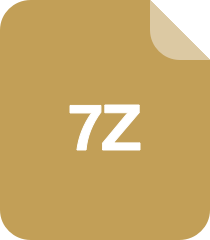
Sha1算法c语言实现
SHA-1(Secure Hash Algorithm 1)是一种广泛使用的哈希函数,用于创建消息摘要或数字签名。在C语言中实现SHA-1算法通常涉及到一些复杂的位操作和循环,因为这个算法涉及大量的异或(XOR)、移位(rotate)和逻辑运算。这里提供一个简化的伪代码版本,实际应用时可能需要使用第三方库如OpenSSL,因为它们已经提供了优化过的实现。
```c
#include <stdio.h>
#include <string.h>
// 假设我们从OpenSSL引入了以下结构体和函数
typedef struct SHA1Context {
// SHA1内部状态变量
} SHA1_CTX;
void SHA1_Init(SHA1_CTX *ctx);
void SHA1_Update(SHA1_CTX *ctx, const unsigned char* data, size_t len);
unsigned char SHA1_Final(unsigned char hash[20], SHA1_CTX *ctx);
// 示例用法
int main() {
SHA1_CTX ctx;
unsigned char input[] = "Hello, world!";
unsigned char hash[20];
SHA1_Init(&ctx);
SHA1_Update(&ctx, input, strlen(input));
SHA1_Final(hash, &ctx);
printf("SHA-1 hash: ");
for (size_t i = 0; i < 20; i++) {
printf("%02x", hash[i]);
}
printf("\n");
return 0;
}
// 注意:这些函数并非真正的C语言代码,而是描述性的说明。在实际项目中,请使用OpenSSL或者其他经过验证的安全库进行SHA-1计算。
```
阅读全文
相关推荐
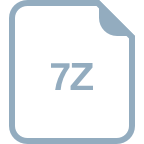
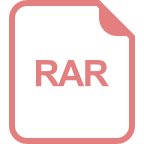















