opencvsharp16位图转8位
时间: 2024-01-19 13:16:02 浏览: 28
在OpenCvSharp中,可以使用ConvertScaleAbs方法将16位图像转换为8位图像。该方法将每个像素的值缩放到0到255之间,并将结果转换为8位无符号整数类型。以下是一个示例代码:
```csharp
using OpenCvSharp;
// 读取16位图像
Mat img16 = Cv2.ImRead("path/to/16bit/image", ImreadModes.AnyDepth);
// 将16位图像转换为8位图像
Mat img8 = new Mat();
Cv2.ConvertScaleAbs(img16, img8, 1.0 / 256.0, 0);
// 保存8位图像
Cv2.ImWrite("path/to/8bit/image", img8);
```
在上面的代码中,我们首先使用ImRead方法读取16位图像,然后使用ConvertScaleAbs方法将其转换为8位图像。最后,我们使用ImWrite方法将8位图像保存到磁盘上。
相关问题
opencv,8位图转16位图
### 回答1:
在 OpenCV 中,可以通过 `convertScaleAbs` 函数将 8 位图像转换为 16 位图像。具体步骤如下:
1. 加载 8 位图像:
```python
import cv2
img_8 = cv2.imread('path/to/8-bit/image.png', cv2.IMREAD_GRAYSCALE)
```
2. 转换为 16 位图像:
```python
img_16 = cv2.convertScaleAbs(img_8, alpha=(65535.0/255.0), beta=0)
```
其中,`alpha` 参数为缩放系数,表示将 8 位图像的像素值缩放至 16 位图像的像素值范围(0~65535)。`beta` 参数为偏移量,表示在缩放后的像素值上加上一个常数。
转换后的 16 位图像 `img_16` 可以使用 OpenCV 的其他函数进行处理和显示。
### 回答2:
将8位图转换为16位图可以使用OpenCV库中的函数cv::cvtcolor()。首先,我们需要加载8位图像,并创建一个16位图像的空白画布。然后使用cv::cvtColor()函数将8位图像转换为16位图像。
以下是一个示例代码:
```python
import cv2
def convert_8bit_to_16bit_image(image_path):
# 加载8位图像
img_8bit = cv2.imread(image_path, cv2.IMREAD_GRAYSCALE)
# 创建一个16位图像的空白画布
img_16bit = np.zeros_like(img_8bit, dtype=np.uint16)
# 将8位图像转换为16位图像
cv2.cvtColor(img_8bit, cv2.COLOR_GRAY2BGR, img_16bit)
return img_16bit
image_path = "8bit_image.png"
converted_image = convert_8bit_to_16bit_image(image_path)
cv2.imwrite("16bit_image.png", converted_image)
cv2.imshow("8-bit Image", cv2.imread(image_path))
cv2.imshow("16-bit Image", converted_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在这个例子中,我们首先加载一个名为"8bit_image.png"的8位图像。然后,我们创建一个与8位图像相同大小的空白16位图像画布。使用cv2.cvtColor()函数,我们将8位图像转换为16位图像。最后,将16位图像保存为"16bit_image.png"并显示在窗口中。
需要注意的是,在转换过程中,我们使用参数cv2.COLOR_GRAY2BGR来指定从灰度图像转换为16位BGR图像。你可以根据需要选择其他颜色空间。
### 回答3:
要将8位图转换为16位图,可以使用OpenCV库中的函数cv2.convertScaleAbs()。
下面是转换过程的步骤:
1. 首先,使用cv2.imread()函数读取8位图像。请确保图像的路径正确,并且图像文件是8位灰度图。
2. 使用cv2.cvtColor()函数将8位图像转换为16位灰度图像。参数为输入图像和转换类型,这里是cv2.COLOR_GRAY2GRAY。
3. 然后,使用cv2.convertScaleAbs()函数将转换后的图像从8位转换为16位。该函数的参数为输入图像、输出图像和比例。
4. 最后,使用cv2.imshow()函数显示转换后的16位图像,并使用cv2.imwrite()函数将图像保存到指定路径上。
下面是一个示例代码:
```python
import cv2
# 读取8位图像
img = cv2.imread("path_to_image.jpg", 0)
# 将8位图像转换为16位
img_16bit = cv2.cvtColor(img, cv2.COLOR_GRAY2GRAY)
# 将转换后的图像从8位转换为16位
img_16bit = cv2.convertScaleAbs(img_16bit, alpha=(65535 / 255))
# 显示16位图像
cv2.imshow("16-bit Image", img_16bit)
cv2.waitKey(0)
# 保存16位图像
cv2.imwrite("path_to_save_image.png", img_16bit)
```
请注意,这只是一个示例,你需要替换"path_to_image.jpg"和"path_to_save_image.png"为你自己的图像路径和保存路径。
此外,你还可以根据自己的需求调整转换比例和其他参数。
python 24位图转 8位_python,opencv,8位图转24位图
将24位图转换为8位图:
```python
import cv2
# 读取24位图
img = cv2.imread('24bit_image.png')
# 将24位图转换为灰度图
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 将灰度图转换为8位图
gray_8bit = cv2.normalize(gray, None, 0, 255, cv2.NORM_MINMAX).astype('uint8')
# 保存8位图
cv2.imwrite('8bit_image.png', gray_8bit)
```
将8位图转换为24位图:
```python
import cv2
# 读取8位图
img = cv2.imread('8bit_image.png', cv2.IMREAD_GRAYSCALE)
# 将8位图转换为24位图
color_map = cv2.applyColorMap(img, cv2.COLORMAP_JET)
# 保存24位图
cv2.imwrite('24bit_image.png', color_map)
```
注意:将8位图转换为24位图时,需要使用`applyColorMap`函数进行转换,同时需要选择一个合适的`COLORMAP`,例如上述代码中使用的是`COLORMAP_JET`。
相关推荐
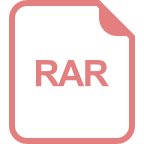












