试写一个算法判别读入的一个以‘@’为结束符的字符序列是否为“回文”。 例如: abcdedcba 或 abccba 是回文。 算法思想: 将依次读入的字符分别插入栈和队列,然后依次比较“栈顶”和“队头”的字符。用C语言循环队列解决,并用Visual C++运行
时间: 2024-10-21 11:12:38 浏览: 60
你可以使用以下C语言实现一个简单的循环队列和栈来判断输入字符序列是否为回文。这里假设我们已经有一个自定义的循环队列结构`CircularQueue`。
```c
#include <stdio.h>
#include <stdbool.h>
// 定义循环队列结构
typedef struct {
char *data;
int front; // 队头元素索引
int rear; // 队尾元素索引
int capacity; // 队列容量
} CircularQueue;
// 初始化循环队列
void init_queue(CircularQueue *queue, int size) {
queue->data = (char*)malloc(size * sizeof(char));
queue->front = -1;
queue->rear = -1;
queue->capacity = size;
}
// 入队操作
bool enqueue(CircularQueue *queue, char c) {
if ((queue->rear + 1) % queue->capacity == queue->front)
return false; // 队满,无法再入队
queue->data[(queue->rear + 1) % queue->capacity] = c;
queue->rear = (queue->rear + 1) % queue->capacity;
return true;
}
// 出队操作
char dequeue(CircularQueue *queue) {
if (queue->front == -1)
return '\0'; // 空队列,无法出队
char temp = queue->data[queue->front];
queue->data[queue->front] = '\0';
queue->front = (queue->front + 1) % queue->capacity;
return temp;
}
// 判断字符串是否为回文
bool is_palindrome(const char *str, int len) {
CircularQueue stack, queue;
init_queue(&stack, len / 2);
init_queue(&queue, len);
for (int i = 0; str[i]; i++) {
enqueue(&stack, str[i]);
enqueue(&queue, str[i]);
}
while (!queue.isEmpty()) {
if (dequeue(&stack) != dequeue(&queue)) {
return false;
}
}
return true;
}
int main() {
char input[100];
printf("请输入以 '@' 结束的字符序列: ");
fgets(input, sizeof(input), stdin);
input[strlen(input) - 1] = '\0'; // 去除换行符
bool isPalindrome = is_palindrome(input, strlen(input));
if (isPalindrome)
printf("%s 是回文。\n", input);
else
printf("%s 不是回文。\n", input);
return 0;
}
```
阅读全文
相关推荐
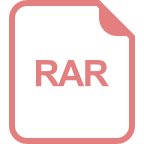
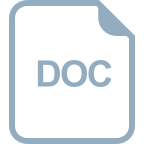
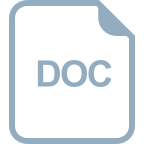















