使用java,mybatis,springroot查询报表中的controller,service文件中的代码
时间: 2024-09-10 17:19:44 浏览: 47
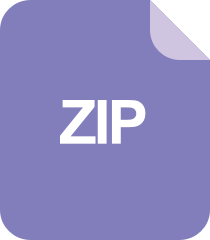
mybatis-spring-2.0.3-API文档-中文版.zip
在使用Java结合MyBatis和Spring框架开发一个报表查询功能时,Controller层通常负责接收用户的请求并返回响应,而Service层则是业务逻辑的处理中心。下面是一个简化的例子来说明这两个层中可能会用到的代码。
Controller层代码示例:
```java
@RestController
@RequestMapping("/report")
public class ReportController {
@Autowired
private ReportService reportService;
@GetMapping("/query")
public ResponseEntity<?> queryReportData(@RequestParam Map<String, Object> params) {
try {
List<Map<String, Object>> reportData = reportService.getReportData(params);
return ResponseEntity.ok(reportData);
} catch (Exception e) {
// 处理异常,返回错误信息
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).body("查询报表数据失败");
}
}
}
```
在这个例子中,`ReportController`类处理对报表数据的查询请求。它依赖于`ReportService`来获取报表数据,并将结果返回给前端。
Service层代码示例:
```java
@Service
public class ReportService {
@Autowired
private ReportMapper reportMapper;
public List<Map<String, Object>> getReportData(Map<String, Object> params) {
// 可能会进行参数校验和处理
return reportMapper.selectReportData(params);
}
}
```
在`ReportService`类中,它依赖于`ReportMapper`接口来实际执行数据库查询操作。`ReportMapper`是一个MyBatis的Mapper接口,它定义了与报表数据表相关的数据库操作方法。
Mapper接口示例(ReportMapper.java):
```java
@Mapper
public interface ReportMapper {
List<Map<String, Object>> selectReportData(@Param("params") Map<String, Object> params);
}
```
`ReportMapper`接口定义了一个查询方法,它会根据传入的参数`params`来执行SQL查询,并返回报表数据。
Mapper XML示例(ReportMapper.xml):
```xml
<mapper namespace="com.yourpackage.ReportMapper">
<select id="selectReportData" resultType="map">
SELECT * FROM report_table WHERE 1 = 1
<if test="params != null">
<foreach collection="params" item="value" index="key" separator=" AND ">
${key} = #{value}
</foreach>
</if>
</select>
</mapper>
```
MyBatis的Mapper XML文件定义了具体的SQL语句,这些SQL语句会根据方法调用时传入的参数动态构建查询条件。
阅读全文
相关推荐
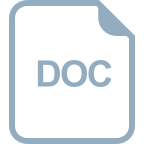
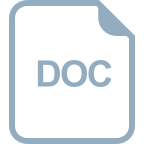
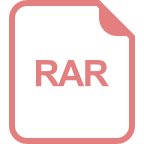
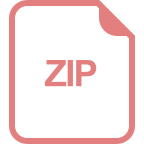
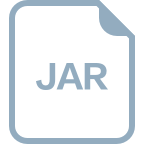
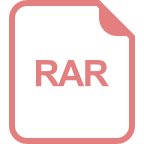
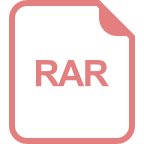
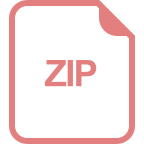
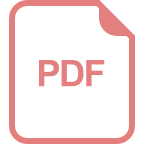
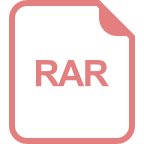
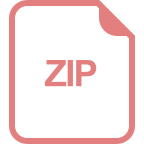
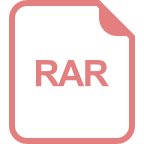
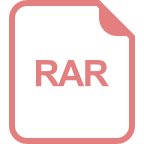
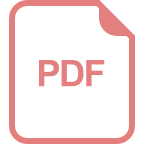
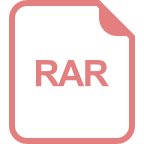
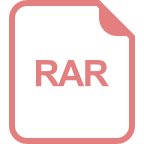
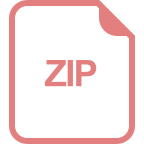