C# 编码实现用户界面点击按钮执行C源码编译的功能
时间: 2024-10-27 18:09:07 浏览: 22
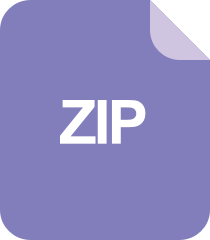
C#的http发送post和get请求源码
在C#中,为了实现在用户界面上点击按钮后执行C源码编译,通常你会结合WPF、WinForms或其他UI框架以及系统命令执行。下面是一个简单的例子,使用Windows Forms和System.Diagnostics.Process类来模拟这个功能:
1. 首先,在Form上添加一个Button控件:
```csharp
private Button btnCompileCode;
public Form1()
{
InitializeComponent();
btnCompileCode = new Button();
btnCompileCode.Text = "编译C源码";
btnCompileCode.Click += BtnCompileCode_Click;
Controls.Add(btnCompileCode);
}
```
2. 定义点击按钮的事件处理函数:
```csharp
private void BtnCompileCode_Click(object sender, EventArgs e)
{
string sourceFile = @"C:\path\to\your\source.c"; // 源代码文件路径
string compileCommand = $"cl /nologo {sourceFile}"; // 使用Clang编译,根据实际情况替换为合适的编译命令
ProcessStartInfo psi = new ProcessStartInfo("cmd.exe", $"/c {compileCommand}");
psi.UseShellExecute = false;
psi.RedirectStandardOutput = true;
psi.RedirectStandardError = true;
using (Process process = new Process())
{
process.StartInfo = psi;
process.Start();
// 监听编译输出
process.StandardOutput.ReadToEnd().WriteToConsole();
process.StandardError.ReadToEnd().WriteToConsole();
if (process.WaitForExit(5000) != true)
throw new TimeoutException("编译超时");
int exitCode = process.ExitCode;
if (exitCode != 0)
MessageBox.Show($"编译失败,退出代码: {exitCode}", "错误", MessageBoxButtons.OK, MessageBoxIcon.Error);
}
}
```
在这个例子中,我们创建了一个`Process`对象,并设置其执行命令为编译器(如`cl`),然后通过`RedirectStandardOutput`和`RedirectStandardError`属性来捕获编译过程的输出和错误。如果编译成功,程序会显示编译结果;如果编译失败,会显示错误消息。
阅读全文
相关推荐
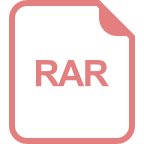
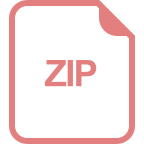
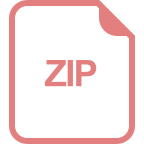
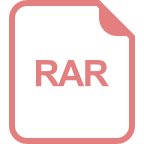
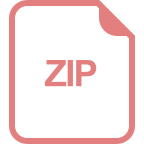
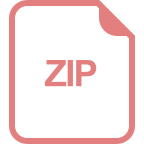
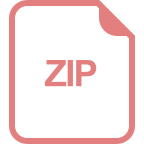
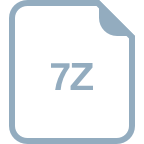
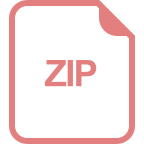
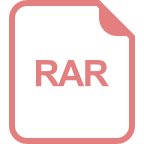
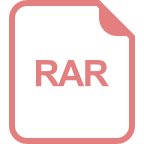
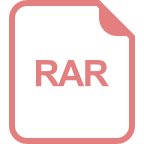
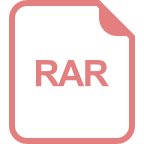
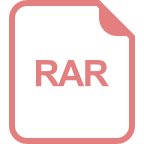
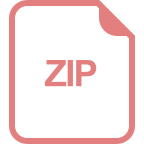
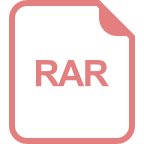
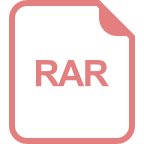
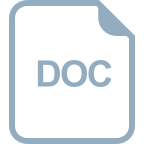