随便给一段python代码
时间: 2024-09-15 20:01:39 浏览: 58
当然可以,这里是一个简单的 Python 代码示例,它定义了一个函数并实现了函数的多态性:
```python
# 定义一个基类 Animal
class Animal:
def __init__(self, name):
self.name = name
# 定义通用的行为
def make_sound(self):
pass
# 定义两个派生类 Dog 和 Cat
class Dog(Animal):
def make_sound(self):
return "Woof!"
class Cat(Animal):
def make_sound(self):
return "Meow!"
# 动物集合,包含多种类型的动物
animals = [Dog("Rex"), Cat("Whiskers")]
# 显示每个动物的声音,体现多态性
for animal in animals:
print(animal.make_sound())
```
在这个例子中,`make_sound()` 方法在基类 `Animal` 中定义,但在派生类 `Dog` 和 `Cat` 中进行了重写,体现了多态性。运行这段代码会根据不同动物实例调用不同的 `make_sound()` 实现。
相关问题
随便给我一段python代码
当然可以。这是一个简单的Python代码片段,它定义了一个函数并使用了输入参数的多态性:
```python
def print_length(item):
if isinstance(item, str): # 判断是否为字符串
length = len(item)
print(f"The string's length is: {length}")
elif isinstance(item, list): # 判断是否为列表
length = len(item)
print(f"The list's length is: {length}")
else:
print("The input type is not supported.")
# 测试示例
print_length("Hello") # 输出:The string's length is: 5
print_length([1, 2, 3, 4, 5]) # 输出:The list's length is: 5
print_length(123) # 输出:The input type is not supported.
请帮我随便写一段python代码
当然可以,以下是一个简单的Python程序,它会输出“Hello, World!”在屏幕上:
```python
print("Hello, World!")
```
如果你想让程序接受用户输入,并将输入的字符串显示在屏幕上,可以使用以下代码:
```python
name = input("What's your name? ")
print("Hello, " + name + "!")
```
这将提示用户输入他们的名字,并将该名称与“Hello, ”连接起来,最终将结果打印到屏幕上。
阅读全文
相关推荐
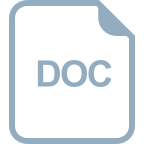
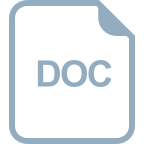
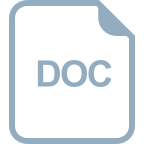













