java ymodem
时间: 2023-10-17 09:05:48 浏览: 99
Ymodem 是一种用于在串行通信中进行文件传输的协议。在 Java 中实现 Ymodem 文件传输可以使用以下步骤:
1. 建立串行通信连接:使用 Java 的串行通信库(如 RXTX)来建立与目标设备的串行通信连接。
2. 实现 Ymodem 协议:在 Java 中实现 Ymodem 协议的核心功能,包括发送文件和接收文件。这涉及到处理 Ymodem 协议的数据包格式、校验和和重传机制等。
3. 发送文件:通过串行连接发送文件,将文件分成多个数据包并使用 Ymodem 协议进行传输。发送过程中需要处理接收方的确认和重传请求。
4. 接收文件:通过串行连接接收文件,解析接收到的数据包并按照 Ymodem 协议进行处理。在接收过程中需要发送确认和重传请求。
需要注意的是,实现 Ymodem 文件传输可能涉及到一些底层的串行通信和字节处理的知识。你可以参考相关的文档和示例代码来帮助你完成这个任务。
相关问题
Java使用ymodem传输
Java可以使用YModem协议进行文件传输,以下是一个基本的示例代码:
```java
import java.io.*;
import java.net.*;
public class YModem {
private static final int PACKET_SIZE = 1024;
private static final int FILE_SIZE = 1024 * 1024;
private static final byte SOH = 0x01;
private static final byte STX = 0x02;
private static final byte EOT = 0x04;
private static final byte ACK = 0x06;
private static final byte NAK = 0x15;
private static final byte CAN = 0x18;
public static void main(String[] args) throws Exception {
if (args.length != 2) {
System.err.println("Usage: java YModem <filename> <port>");
System.exit(1);
}
String filename = args[0];
String portName = args[1];
try (RandomAccessFile file = new RandomAccessFile(filename, "r");
SerialPort port = new SerialPort(portName)) {
// send file name and size
sendFileNameAndSize(port, filename, (int) file.length());
// send file data
sendFileData(port, file);
// end of transmission
sendEOT(port);
}
}
private static void sendFileNameAndSize(SerialPort port, String filename, int fileSize) throws Exception {
// send file name
byte[] nameBytes = filename.getBytes();
port.write(nameBytes);
port.write(0);
// send file size
String fileSizeStr = String.format("%d", fileSize);
byte[] sizeBytes = fileSizeStr.getBytes();
port.write(sizeBytes);
port.write(0);
}
private static void sendFileData(SerialPort port, RandomAccessFile file) throws Exception {
byte[] buffer = new byte[PACKET_SIZE];
int bytesRead;
int blockNumber = 1;
while ((bytesRead = file.read(buffer)) != -1) {
sendPacket(port, blockNumber, buffer, bytesRead);
blockNumber++;
}
}
private static void sendPacket(SerialPort port, int blockNumber, byte[] data, int length) throws Exception {
byte packetNumber = (byte) blockNumber;
byte packetNumberComplement = (byte) ~blockNumber;
// send packet header
if (length == PACKET_SIZE) {
port.write(STX);
} else {
port.write(SOH);
}
port.write(packetNumber);
port.write(packetNumberComplement);
// send packet data
port.write(data, 0, length);
int paddingLength = PACKET_SIZE - length;
for (int i = 0; i < paddingLength; i++) {
port.write(0);
}
// wait for ACK or NAK
byte response = port.read();
if (response == ACK) {
// packet successfully received
return;
} else if (response == NAK) {
// packet not received, resend
sendPacket(port, blockNumber, data, length);
} else if (response == CAN) {
// transmission cancelled
throw new Exception("Transmission cancelled");
} else {
// unknown response
throw new Exception("Unknown response: " + response);
}
}
private static void sendEOT(SerialPort port) throws Exception {
// send EOT
port.write(EOT);
// wait for ACK
byte response = port.read();
if (response != ACK) {
throw new Exception("Unknown response: " + response);
}
}
}
```
注意,此示例代码仅用于演示目的,实际使用中需要根据具体需求进行修改和完善。同时,还需要使用相应的串口库(如 RXTX)来连接串口设备。
java 采用 Ymodem传输文件
Java可以使用串口通讯库来实现Ymodem传输文件。以下是一个简单的示例代码:
```java
import java.io.*;
import java.util.*;
import purejavacomm.*;
public class YModem {
private static final byte SOH = 0x01;
private static final byte STX = 0x02;
private static final byte EOT = 0x04;
private static final byte ACK = 0x06;
private static final byte NAK = 0x15;
private static final byte CAN = 0x18;
private static final byte CRC16 = 0x43;
private static final int BLOCK_SIZE = 1024;
private static final int MAX_RETRIES = 10;
private static final int FILE_SIZE_OFFSET = 132;
private static final int FILE_SIZE_LENGTH = 10;
private static final int FILE_NAME_OFFSET = 0;
private static final int FILE_NAME_LENGTH = 128;
public static void send(String fileName, OutputStream outputStream) throws Exception {
File file = new File(fileName);
FileInputStream fileInputStream = new FileInputStream(file);
long fileSize = file.length();
byte[] fileNameBytes = Arrays.copyOf(file.getName().getBytes("ASCII"), FILE_NAME_LENGTH);
byte[] fileSizeBytes = Arrays.copyOf(Long.toString(fileSize).getBytes("ASCII"), FILE_SIZE_LENGTH);
int blockNumber = 1;
int retries = 0;
while (true) {
outputStream.write(NAK);
int bytesRead = fileInputStream.read();
if (bytesRead != SOH) {
throw new Exception("Invalid start of header");
}
int blockIndex = 0;
byte[] block = new byte[BLOCK_SIZE + 4];
block[blockIndex++] = SOH;
block[blockIndex++] = (byte) blockNumber;
block[blockIndex++] = (byte) ~blockNumber;
bytesRead = fileInputStream.read(block, blockIndex, BLOCK_SIZE);
if (bytesRead < BLOCK_SIZE) {
Arrays.fill(block, blockIndex + bytesRead, blockIndex + BLOCK_SIZE, (byte) 0x1A);
}
int crc = calculateCRC16(block, 3, BLOCK_SIZE);
block[blockIndex + BLOCK_SIZE] = (byte) ((crc >> 8) & 0xFF);
block[blockIndex + BLOCK_SIZE + 1] = (byte) (crc & 0xFF);
outputStream.write(block);
int response = readByte(inputStream);
if (response == ACK) {
blockNumber++;
if (blockNumber == 256) {
blockNumber = 0;
}
retries = 0;
if (bytesRead < BLOCK_SIZE) {
break;
}
} else if (response == NAK) {
retries++;
if (retries >= MAX_RETRIES) {
throw new Exception("Transfer failed");
}
} else {
throw new Exception("Invalid response: " + response);
}
}
fileInputStream.close();
while (true) {
outputStream.write(EOT);
int response = readByte(inputStream);
if (response == NAK) {
continue;
} else if (response == ACK) {
break;
} else {
throw new Exception("Invalid response: " + response);
}
}
}
public static void receive(String fileName, InputStream inputStream) throws Exception {
FileOutputStream fileOutputStream = new FileOutputStream(fileName);
byte[] block = new byte[BLOCK_SIZE + 4];
int blockNumber = 1;
int retries = 0;
boolean endOfFile = false;
while (!endOfFile) {
block[0] = (byte) inputStream.read();
if (block[0] == EOT) {
outputStream.write(ACK);
break;
} else if (block[0] != SOH && block[0] != STX) {
throw new Exception("Invalid start of header");
}
int bytesRead = inputStream.read(block, 1, BLOCK_SIZE + 3);
if (bytesRead < BLOCK_SIZE + 3) {
throw new Exception("Invalid block size");
}
int crc = calculateCRC16(block, 3, BLOCK_SIZE);
if (crc != ((block[BLOCK_SIZE + 3] << 8) | (block[BLOCK_SIZE + 2] & 0xFF))) {
throw new Exception("Invalid CRC");
}
if (blockNumber == block[1] && ~blockNumber == block[2]) {
retries = 0;
fileOutputStream.write(block, 3, BLOCK_SIZE);
outputStream.write(ACK);
blockNumber++;
if (blockNumber == 256) {
blockNumber = 0;
}
} else if (blockNumber == ((block[1] + 1) & 0xFF) && ~(blockNumber) == block[2]) {
retries = 0;
endOfFile = true;
int bytesToWrite = 0;
for (int i = 0; i < FILE_SIZE_LENGTH; i++) {
if (fileSizeBytes[i] == 0) {
break;
}
bytesToWrite = bytesToWrite * 10 + (fileSizeBytes[i] - '0');
}
fileOutputStream.write(block, 3, bytesToWrite);
outputStream.write(ACK);
} else {
retries++;
if (retries >= MAX_RETRIES) {
throw new Exception("Transfer failed");
}
outputStream.write(NAK);
}
}
fileOutputStream.close();
}
private static int calculateCRC16(byte[] data, int offset, int length) {
int crc = 0;
for (int i = offset; i < offset + length; i++) {
crc ^= (data[i] & 0xFF) << 8;
for (int j = 0; j < 8; j++) {
if ((crc & 0x8000) != 0) {
crc = (crc << 1) ^ 0x1021;
} else {
crc <<= 1;
}
}
}
return crc & 0xFFFF;
}
private static int readByte(InputStream inputStream) throws Exception {
long startTime = System.currentTimeMillis();
while (inputStream.available() == 0) {
if (System.currentTimeMillis() - startTime > 10000) {
throw new Exception("Timeout waiting for response");
}
}
return inputStream.read();
}
}
```
在这个示例代码中,send()方法用于发送文件,而receive()方法用于接收文件。如果您使用的是Windows系统,则需要安装Java Communications API(JavaComm)来使用串口通讯库。
阅读全文
相关推荐
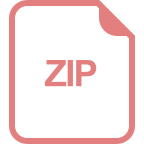
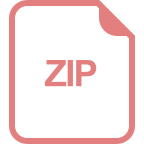
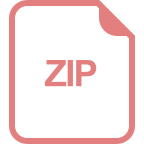
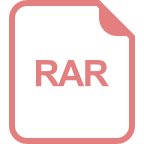
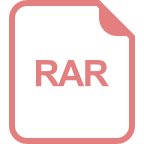
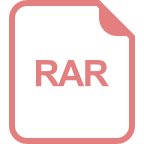
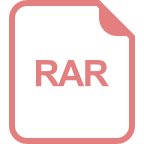
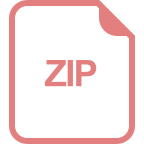
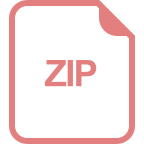
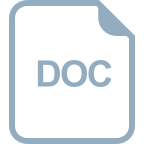
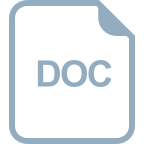
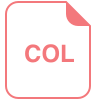
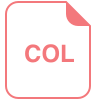
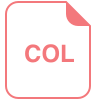