java创建一个OAuth1.0的post请求
时间: 2024-11-06 20:18:33 浏览: 7
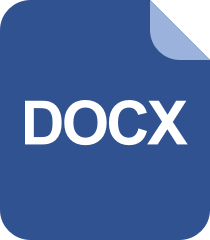
OAuth1.0中文文档

在Java中,创建一个OAuth 1.0的POST请求通常涉及到几个步骤,包括获取授权、构造URL、设置HTTP头部和发送请求。这里是一个简单的示例,假设你已经拥有客户端ID、客户端密钥、访问令牌和秘密:
```java
import org.apache.http.HttpResponse;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
public class OAuthRequest {
private static final String CONSUMER_KEY = "your_consumer_key";
private static final String CONSUMER_SECRET = "your_consumer_secret";
private static final String TOKEN = "your_access_token";
private static final String TOKEN_SECRET = "your_access_token_secret";
public void makeOAuthPostRequest(String url, String data) throws Exception {
// 创建HttpClient实例
CloseableHttpClient httpClient = HttpClients.createDefault();
try {
// 构造OAuth URL
String oAuthUrl = constructOAuthUrl(url);
// 创建HttpPost
HttpPost httpPost = new HttpPost(oAuthUrl);
// 设置Authorization header
httpPost.setHeader("Authorization", createOAuthHeader());
// 将数据转换为StringEntity并添加到请求中
httpPost.setEntity(new StringEntity(data, "application/x-www-form-urlencoded"));
// 发送POST请求
CloseableHttpResponse response = httpClient.execute(httpPost);
// 检查响应状态码并处理结果
int statusCode = response.getStatusLine().getStatusCode();
if (statusCode == HttpStatus.SC_OK) {
System.out.println("Request successful. Response: " + EntityUtils.toString(response.getEntity()));
} else {
throw new RuntimeException("Failed to post request with status code: " + statusCode);
}
} finally {
// 关闭连接
httpClient.close();
}
}
private String constructOAuthUrl(String base) {
// 根据实际需求拼接完整的OAuth URL,例如:
return "https://api.example.com/oauth/access_token?oauth_consumer_key=" + CONSUMER_KEY +
"&oauth_nonce=" + generateNonce() +
"&oauth_signature_method=HMAC-SHA1" +
"&oauth_timestamp=" + System.currentTimeMillis() / 1000 +
"&oauth_token=" + TOKEN +
"&oauth_version=1.0";
}
private String createOAuthHeader() {
String oauthSignatureBaseString = "POST&" + Uri.encode(oAuthUrl) + "&" + Uri.encode(data);
return "OAuth realm=\"\"",
"oauth_consumer_key=\"" + CONSUMER_KEY + "\",",
"oauth_token=\"" + TOKEN + "\",",
"oauth_signature_method=\"HMAC-SHA1\",",
"oauth_timestamp=\"" + Long.toString(System.currentTimeMillis() / 1000) + "\",",
"oauth_nonce=\"" + generateNonce() + "\",",
"oauth_signature=" + getSignature(oauthSignatureBaseString);
}
// 假设getSignature方法可以根据给定的字符串生成签名,此处略去具体实现
private String getSignature(String oauthSignatureBaseString) {
// 实现具体的签名计算逻辑,通常涉及HMAC-SHA1算法和密钥
// ...
}
// 生成随机nonce值
private String generateNonce() {
// 生成随机字符串作为nonce
return UUID.randomUUID().toString();
}
// 其他相关问题:
阅读全文
相关推荐
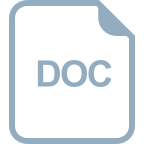
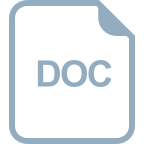

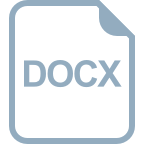
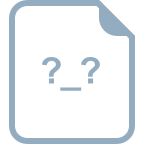
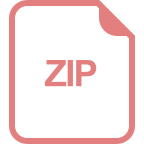
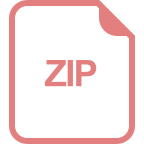
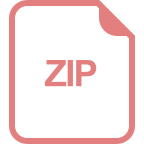
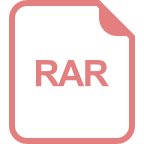
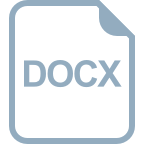
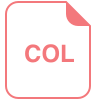
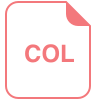
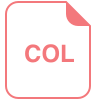
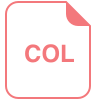
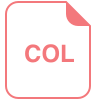
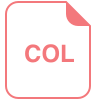
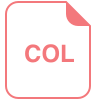
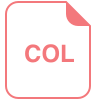