编程3:设计一个汽车类Car。成员变量有:品牌,颜色,车门,车灯,行驶速度。成员函数有:打开车门、打开车灯、加速、减速等。最后,编写一个主程序来测试该类及功能。(提示:在成员函数中用输出成员函数名来表示该成员函数被调用 用C++语言
时间: 2024-11-03 19:17:23 浏览: 19
在C++中,你可以按照下面的方式设计一个简单的`Car`类来满足这个需求:
```cpp
#include <iostream>
using namespace std;
class Car {
public:
// 成员变量
string brand;
string color;
int doors;
bool lights; // 假设车灯是一个布尔值
float speed;
// 构造函数初始化车辆属性
Car(string b, string c, int d) : brand(b), color(c), doors(d), lights(false), speed(0) {}
// 成员函数
void openDoor() {
cout << "打开车门..." << endl;
}
void toggleLights() {
if (lights) {
cout << "关闭车灯..." << endl;
} else {
cout << "打开车灯..." << endl;
}
lights = !lights;
}
void accelerate() {
if (speed < 100) {
speed += 10;
cout << "加速..." << endl;
} else {
cout << "车速已满,无法再加速..." << endl;
}
}
void decelerate() {
if (speed > 0) {
speed -= 10;
cout << "减速..." << endl;
} else {
cout << "车速已经为零,无法再减速..." << endl;
}
}
// 主程序测试
void testFunctions() {
openDoor();
toggleLights();
accelerate();
decelerate();
cout << "当前车速:" << speed << " km/h" << endl;
}
};
int main() {
Car myCar("宝马", "黑色", 4);
myCar.testFunctions();
return 0;
}
```
在这个例子中,我们创建了一个`Car`类,并定义了它的属性(如品牌、颜色、车门数、车灯状态和速度)以及相应的操作(打开车门、开关车灯、加速和减速)。在`main`函数中,我们创建了一个`myCar`对象并对其进行了一些功能的测试。
阅读全文
相关推荐
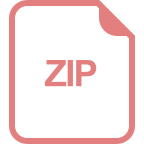
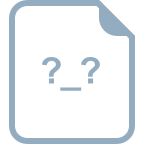
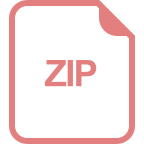
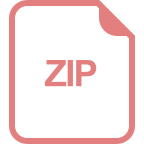
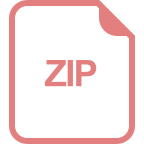
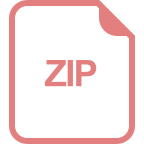
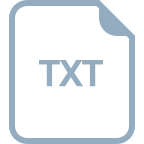
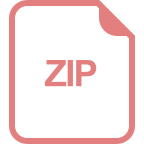
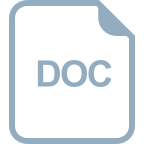
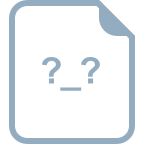
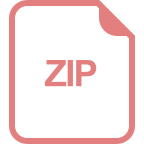
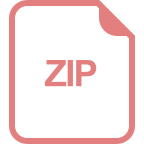
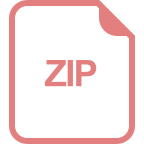
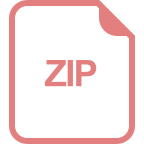
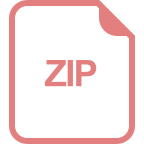
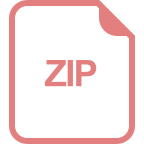
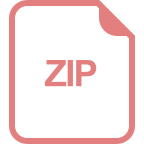
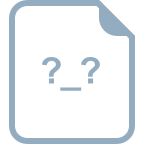
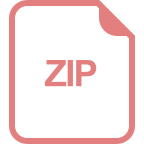