进化策略( 1+1) -ES附上python代码实现
时间: 2024-10-11 22:08:16 浏览: 16
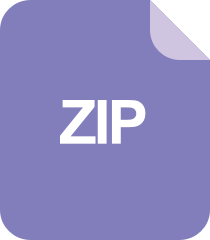
1+1 进化策略(ES):1+1-ES。 最简单的ES。 阅读文件中的注释以获得更深入的解释和参考书目-matlab开发
进化策略 (1+1)-ES是一种简单的遗传算法,也称为单点交叉和变异的进化策略。它基本的思想是在每个迭代步骤中,只选择一对随机解(通常称为“父母”),对它们进行变异操作(如添加一个小的随机变化)生成一个新的解,然后将这个新解替换掉其中一个较差的原始个体。"1"代表一个解,“1+1”表示每次操作只涉及两个个体。
以下是基于Python的一个简单实现:
```python
import numpy as np
class ES1Plus1:
def __init__(self, population_size, problem_dim, mutation_rate):
self.population = np.random.rand(population_size, problem_dim)
self.mutation_rate = mutation_rate
def evolve(self, fitness_func):
# 计算当前种群的适应度
fitness = [fitness_func(individual) for individual in self.population]
# 按照适应度排序
sorted_indices = np.argsort(fitness)[::-1] # 最优个体排在前面
best_individual = self.population[sorted_indices[0]]
# 随机选择一个个体作为父代
parent_index = np.random.randint(len(self.population))
parent = self.population[parent_index]
# 变异操作
offspring = parent + np.random.normal(scale=self.mutation_rate, size=parent.shape)
# 替换适应度较低的个体
self.population[sorted_indices[0]] = offspring if fitness_func(offspring) > fitness[parent_index] else parent
def get_best_solution(self):
return self.population[np.argmax([fitness_func(individual) for individual in self.population])]
# 使用示例
def example_fitness_function(x):
return x**2 # 示例优化函数,求平方最小值
es = ES1Plus1(50, 10, 0.1) # 创建一个10维问题的种群,大小50,变异率为0.1
for _ in range(100): # 迭代100次
es.evolve(example_fitness_function)
best_solution = es.get_best_solution()
```
在这个例子中,`example_fitness_function`是你需要解决的具体问题的目标函数,你可以根据实际问题替换成相应的评估函数。每次`evolve`方法都会改进种群,直到达到预设的迭代次数或满足其他停止条件。
阅读全文
相关推荐
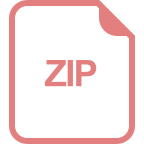
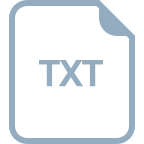
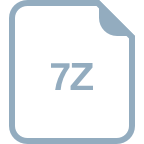
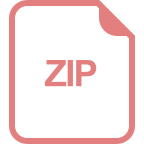
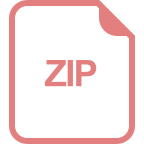
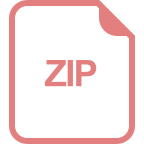
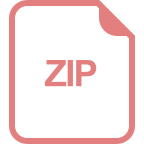
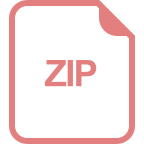
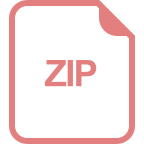
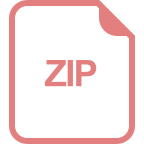
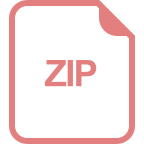
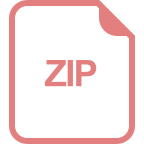
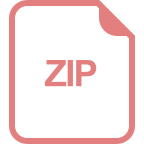
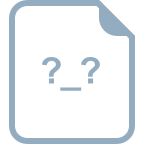
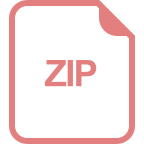
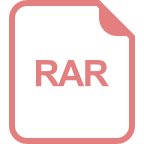
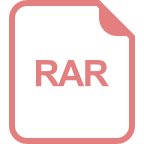
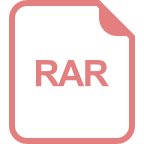