有两个矩阵a和b,均为3行2列,定义矩阵类Matrix,重载运算符“+”,使之能用于两矩阵相加。在主函数中对矩阵a,b相加进行测试,并输出结果。c++
时间: 2024-11-03 18:23:15 浏览: 71
在C++中,你可以定义一个名为`Matrix`的类来表示二维矩阵,并重载`+`运算符以实现矩阵相加的操作。下面是一个简单的实现:
```cpp
#include <iostream>
using namespace std;
class Matrix {
private:
int rows;
int cols;
double data[3][2]; // 假设3行2列
public:
Matrix(int r = 3, int c = 2) : rows(r), cols(c) {
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j) {
data[i][j] = 0.0; // 初始化为零
}
}
}
// 获取元素
double get(int i, int j) const {
return data[i][j];
}
// 设置元素
void set(int i, int j, double value) {
data[i][j] = value;
}
// 矩阵加法运算符+(重载)
Matrix operator+(const Matrix& other) const {
if (rows != other.rows || cols != other.cols) {
throw runtime_error("Matrices must have the same dimensions for addition.");
}
Matrix result(rows, cols);
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j) {
result.data[i][j] = data[i][j] + other.data[i][j];
}
}
return result;
}
// 打印矩阵
void print() const {
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j) {
cout << data[i][j] << " ";
}
cout << endl;
}
}
};
int main() {
Matrix a(3, 2);
Matrix b(3, 2);
// 初始化矩阵a和b
a.set(0, 0, 1);
a.set(0, 1, 2);
a.set(1, 0, 3);
a.set(1, 1, 4);
a.set(2, 0, 5);
a.set(2, 1, 6); // 假设其他元素都为0
b.set(0, 0, 7);
b.set(0, 1, 8);
b.set(1, 0, 9);
b.set(1, 1, 10);
b.set(2, 0, 11);
b.set(2, 1, 12); // 假设其他元素都为0
// 打印原矩阵
cout << "Matrix a:" << endl;
a.print();
cout << "Matrix b:" << endl;
b.print();
// 相加并打印结果
Matrix c = a + b;
cout << "Matrix a + b:" << endl;
c.print();
return 0;
}
```
在这个例子中,我们定义了一个`Matrix`类,包含了矩阵的基本属性和一些操作,包括初始化、获取和设置元素,矩阵加法,以及打印矩阵。在`main`函数中,我们创建了两个矩阵`a`和`b`,进行了加法运算,并打印出结果。
阅读全文
相关推荐

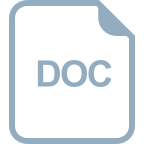
















