C++编程,从键盘上输入一个字符串,然后统计其中大写字母、小写字母、数字、空格和其它字符的个数。(用指针实现)
时间: 2024-12-12 17:26:26 浏览: 11
在C++中,你可以使用指针来遍历输入的字符串并分别计数各种字符。这里是一个简单的示例,展示如何完成这个任务:
```cpp
#include <iostream>
#include <cctype> // 引入标准库中的ctype_h头文件,用于处理字符类别
int main() {
char input[100]; // 存储输入字符串
std::cout << "请输入一个字符串: ";
std::cin.getline(input, sizeof(input)); // 从键盘获取输入
int uppercase = 0;
int lowercase = 0;
int digits = 0;
int spaces = 0;
int other_chars = 0;
for (char* ptr = input; *ptr != '\0'; ++ptr) { // 遍历字符串直到遇到结束符
if (isupper(*ptr)) { // 判断是否为大写字母
uppercase++;
} else if (islower(*ptr)) { // 判断是否为小写字母
lowercase++;
} else if (isdigit(*ptr)) { // 判断是否为数字
digits++;
} else if (*ptr == ' ') { // 判断是否为空格
spaces++;
} else { // 其他字符(非字母、数字、空格)
other_chars++;
}
}
std::cout << "大写字母: " << uppercase << "\n";
std::cout << "小写字母: " << lowercase << "\n";
std::cout << "数字: " << digits << "\n";
std::cout << "空格: " << spaces << "\n";
std::cout << "其他字符: " << other_chars << "\n";
return 0;
}
```
阅读全文
相关推荐
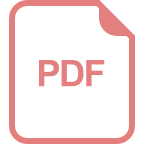
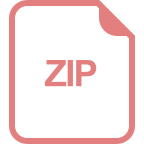
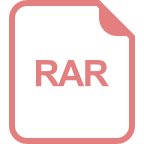















