c语言链表的基本形式
时间: 2024-12-18 15:05:19 浏览: 2
链表是一种常见的数据结构,它由一系列节点组成,每个节点包含数据和指向下一个节点的指针。链表的基本形式如下:
1. **节点结构**:每个节点包含数据和指向下一个节点的指针。
2. **头指针**:指向链表的第一个节点。
3. **尾指针**:指向链表的最后一个节点(可选)。
以下是一个简单的C语言链表节点结构的定义:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构
struct Node {
int data; // 数据部分
struct Node* next; // 指向下一个节点的指针
};
// 创建一个新节点
struct Node* createNode(int data) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
if (!newNode) {
printf("内存分配失败\n");
exit(1);
}
newNode->data = data;
newNode->next = NULL;
return newNode;
}
// 插入节点到链表末尾
void appendNode(struct Node** headRef, int data) {
struct Node* newNode = createNode(data);
if (*headRef == NULL) {
*headRef = newNode;
return;
}
struct Node* current = *headRef;
while (current->next != NULL) {
current = current->next;
}
current->next = newNode;
}
// 打印链表
void printList(struct Node* head) {
struct Node* current = head;
while (current != NULL) {
printf("%d -> ", current->data);
current = current->next;
}
printf("NULL\n");
}
// 主函数
int main() {
struct Node* head = NULL;
appendNode(&head, 1);
appendNode(&head, 2);
appendNode(&head, 3);
printList(head);
return 0;
}
```
在这个示例中,我们定义了一个链表节点结构 `Node`,并实现了创建节点、插入节点到链表末尾以及打印链表的功能。
阅读全文
相关推荐






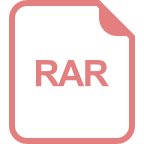











