2,将字符中单词用空格隔开 # 已知传入的字符串中只有字母,每个单词的首字母大写, # 请将每个单词用空格隔开,只保留第一个单词的首字母大写 # 传入:”HelloMyWorld” # 返回:”Hello my world”
时间: 2024-09-30 14:14:21 浏览: 30
这个任务涉及到了字符串处理和格式化,主要目标是将每个单词的首字母大写并用空格隔开。在英语中,一般采用 `title()` 函数来实现单词首字母大写的效果。下面是一个简单的 Python 解决方案:
```python
def format_string(input_str):
formatted_words = input_str.title().split() # 将字符串转化为单词列表,首字母大写
formatted_result = " ".join(formatted_words) # 用空格连接成新字符串
return formatted_result
input_str = "HelloMyWorld"
formatted_output = format_string(input_str)
print(f"格式化后的字符串: {formatted_output}")
```
在这个例子中,`title()` 方法将每个单词的首字母转换为大写,然后 `split()` 函数将字符串拆分成单词列表。最后,`join()` 方法通过空格把这些单词再组合成一个新的字符串。
相关问题
将从键盘上输入的一个字符串的每个单词的第一个字母转换为大写字母,输入时各单词必须用空格隔开。
可以使用字符串的split()方法将输入的字符串按空格分割成单词,然后遍历每个单词,将第一个字母转换为大写字母,最后将所有单词拼接成一个新的字符串输出。
示例代码如下:
```
s = input("请输入字符串:")
words = s.split() # 按空格分割成单词
new_words = []
for word in words:
new_word = word.capitalize() # 将第一个字母转换为大写字母
new_words.append(new_word)
new_s = ' '.join(new_words) # 拼接成新的字符串
print(new_s)
```
输入示例:
```
请输入字符串:hello world
```
输出示例:
```
Hello World
```
字符串每个单词首字母大写
可以使用 Python 的 title() 方法来实现字符串每个单词首字母大写的操作,示例如下:
```python
s = "hello world"
s = s.title()
print(s)
```
输出结果为:
```
Hello World
```
title() 方法会将字符串中每个单词的首字母转换为大写,并返回转换后的字符串。注意,该方法不会改变原始字符串,而是返回一个新的字符串。
阅读全文
相关推荐
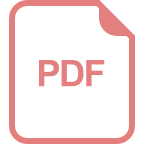
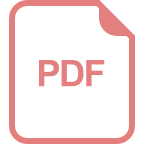
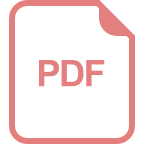
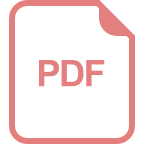












