创建数组A: ■(1&0&-1@3&5&0@0&4&1)
时间: 2024-09-20 22:08:30 浏览: 29
创建数组A的描述似乎采用了某种特定的编码规则或者是一种数据结构的简写。这里"■"可能是数组的开始标记,而后面的序列看起来像是元素的值和它们的索引。每个"(&)"后面的一串数字可能表示数组的一个元素及其对应位置:
1. "1&0&-1" 可能意味着第一个元素的值是1,在位置0处;
2. "@3&5&0" 可能指第二个元素的值是5,在索引3处(因为通常数组的索引从0开始);
3. "@0&4&1" 可能表示第三个元素的值是4,在索引0处,另一个值1可能是一个额外的信息。
这种表示可能是一个交错数组(sparse array),其中某些位置是空的(0)。要实际转化为计算机可以处理的数据结构,比如在Python中:
```python
# 假设数组的每个"&"分隔的是两个值:值和索引
A = {
0: 1,
3: 5,
0: 4, # 注意这里的索引可能会有重复,需要处理冲突
1: 1, # 如果最后一个1表示附加到前面元素的值,则可能需要进一步解析
}
```
或者是一个更复杂的数据结构,具体取决于上下文。如果还有其他细节没提供,转换会有所不同。如果没有特别的规则说明,这些只是猜测。
相关问题
设计Account1类,包含: ■ 一个名为id 的int 类型的私有数据域(默认值为0),长度为6位。 ■ 一个名为balance的double类型的私有数据域(默认值为0)。 ■ 一个名为annualInterestRate 的double类型的私有数据域存储当前利率(默认值为0)。假设所有的账户都有相同的利率。 ■ 一个名为dateCreated的Date类型的私有数据域存储账户的开户日期。 ■ 一个能创建默认账户的无参构造方法。 ■ 一个能创建带特定id和初始余额的构造方法,初始余额不能为负数。 ■ id、balance和annualInterestRate 的访问器和修改器。 ■ dateCreated的访问器。 ■ 一个名为getMonthlyInterestRate的方法返回月利率。 ■ 一个名为withDraw的方法从账户提取特定金额。 ■ 一个名为deposit的方法向账户存人特定金额。 ■ double类型的数据域保留2位小数。 ■ 成员方法和数据域应进行基本的合理性检查。 设计测试类ATMMachine1: ■ 创建一个有100个账户的数组,其id 为0,1,2,...99, 并初始化收支为1000美元。 ■ 主菜单如下: Main menu 1: check balance 2: withdraw 3: deposit 4: exit
下面是Account1类和ATMMachine1类的代码实现:
```
import java.util.Date;
public class Account1 {
private int id;
private double balance;
private double annualInterestRate;
private Date dateCreated;
public Account1() {
id = 0;
balance = 0;
annualInterestRate = 0;
dateCreated = new Date();
}
public Account1(int id, double balance) {
this.id = id;
if (balance >= 0) {
this.balance = balance;
} else {
System.out.println("Initial balance cannot be negative.");
this.balance = 0;
}
annualInterestRate = 0;
dateCreated = new Date();
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public double getBalance() {
return balance;
}
public void setBalance(double balance) {
this.balance = balance;
}
public double getAnnualInterestRate() {
return annualInterestRate;
}
public void setAnnualInterestRate(double annualInterestRate) {
this.annualInterestRate = annualInterestRate;
}
public Date getDateCreated() {
return dateCreated;
}
public double getMonthlyInterestRate() {
return annualInterestRate / 12;
}
public void withdraw(double amount) {
if (balance >= amount) {
balance -= amount;
} else {
System.out.println("Insufficient balance.");
}
}
public void deposit(double amount) {
if (amount >= 0) {
balance += amount;
} else {
System.out.println("Deposit amount cannot be negative.");
}
}
public String toString() {
return "Account ID: " + id + "\nBalance: $" + String.format("%.2f", balance) + "\nAnnual Interest Rate: " + annualInterestRate + "%\nDate Created: " + dateCreated;
}
}
import java.util.Scanner;
public class ATMMachine1 {
public static void main(String[] args) {
Account1[] accounts = new Account1[100];
for (int i = 0; i < accounts.length; i++) {
accounts[i] = new Account1(i, 1000);
}
Scanner input = new Scanner(System.in);
int choice = 0;
while (choice != 4) {
System.out.println("Main menu");
System.out.println("1: check balance");
System.out.println("2: withdraw");
System.out.println("3: deposit");
System.out.println("4: exit");
System.out.print("Enter a choice: ");
choice = input.nextInt();
switch (choice) {
case 1:
System.out.print("Enter an account ID (0-99): ");
int id = input.nextInt();
if (id >= 0 && id <= 99) {
System.out.println(accounts[id]);
} else {
System.out.println("Invalid account ID.");
}
break;
case 2:
System.out.print("Enter an account ID (0-99): ");
id = input.nextInt();
if (id >= 0 && id <= 99) {
System.out.print("Enter an amount to withdraw: ");
double amount = input.nextDouble();
accounts[id].withdraw(amount);
} else {
System.out.println("Invalid account ID.");
}
break;
case 3:
System.out.print("Enter an account ID (0-99): ");
id = input.nextInt();
if (id >= 0 && id <= 99) {
System.out.print("Enter an amount to deposit: ");
double amount = input.nextDouble();
accounts[id].deposit(amount);
} else {
System.out.println("Invalid account ID.");
}
break;
case 4:
System.out.println("Goodbye!");
break;
default:
System.out.println("Invalid choice.");
}
System.out.println();
}
}
}
```
这个程序创建了一个有100个账户的数组,初始收支为1000美元。主菜单提供了4个选项:检查余额、提取金额、存款和退出。用户可以根据需要选择相应的选项,对账户进行操作。每个账户都有一个6位的ID、余额和利率,以及一个开户日期。可以使用访问器和修改器来获取或设置这些值。程序还提供了getMonthlyInterestRate、withdraw和deposit等方法来计算月利率、提取特定金额和存入特定金额。这个程序还对输入进行了错误检查,确保提取金额不超过余额,存款金额不为负数,账户ID在0到99之间等。
将上面的抽象类GeometricObject改为接口,接口只保留其中四个抽象方法,声明类Circle、Triangle实现该接口,类的基本要求如上,同时为每个类增加一个将当前对象序列化到指定文件的方法writeToFile(File f)。 测试类TestGeometricObject2设计要求如下: ■ 一个能随机生成Circle类和Triangle类对象的静态方法,该方法将随机生成的象序列化到指定的文件GeometricObjects.dat中,序列化成功返回真,否则返回假。 Boolean RandomCreateGeometricObject() ■ 将GeometricObjects.dat文件中对象全部读出,存储到GeometricObject对象数组中,然后以此数组为参数,输出数组中每个对象的基本信息、周长和面积。 ■ 类中其它方法的测试。 新增一个类Rectangle,也实现接口GeometricObject,同时修改测试类TestGeometricObject2。 版本3: 在第2步的基础上设计实现一个具有GUI界面的几何图形绘制系统系统,要求实现根据选择的几何图形类型来绘制和删除相应的图形,其中相关参数应通过界面输入,并可计算图形的周长和面积。 javafx
抽象接口GeometricObject如下:
```java
public interface GeometricObject {
public double getPerimeter();
public double getArea();
public void draw();
public void writeToFile(File f);
}
```
类Circle实现GeometricObject接口:
```java
public class Circle implements GeometricObject, Serializable {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
@Override
public double getPerimeter() {
return 2 * Math.PI * radius;
}
@Override
public double getArea() {
return Math.PI * radius * radius;
}
@Override
public void draw() {
System.out.println("Draw a circle with radius " + radius);
}
@Override
public void writeToFile(File f) {
try {
ObjectOutputStream out = new ObjectOutputStream(new FileOutputStream(f));
out.writeObject(this);
out.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
类Triangle实现GeometricObject接口:
```java
public class Triangle implements GeometricObject, Serializable {
private double side1;
private double side2;
private double side3;
public Triangle(double side1, double side2, double side3) {
this.side1 = side1;
this.side2 = side2;
this.side3 = side3;
}
@Override
public double getPerimeter() {
return side1 + side2 + side3;
}
@Override
public double getArea() {
double s = getPerimeter() / 2;
return Math.sqrt(s * (s - side1) * (s - side2) * (s - side3));
}
@Override
public void draw() {
System.out.println("Draw a triangle with sides " + side1 + ", " + side2 + ", " + side3);
}
@Override
public void writeToFile(File f) {
try {
ObjectOutputStream out = new ObjectOutputStream(new FileOutputStream(f));
out.writeObject(this);
out.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
类Rectangle实现GeometricObject接口:
```java
public class Rectangle implements GeometricObject, Serializable {
private double width;
private double height;
public Rectangle(double width, double height) {
this.width = width;
this.height = height;
}
@Override
public double getPerimeter() {
return 2 * (width + height);
}
@Override
public double getArea() {
return width * height;
}
@Override
public void draw() {
System.out.println("Draw a rectangle with width " + width + ", height " + height);
}
@Override
public void writeToFile(File f) {
try {
ObjectOutputStream out = new ObjectOutputStream(new FileOutputStream(f));
out.writeObject(this);
out.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
测试类TestGeometricObject2如下:
```java
import java.io.*;
import java.util.Random;
public class TestGeometricObject2 {
public static void main(String[] args) {
// 随机生成Circle和Triangle类对象,并将对象序列化到GeometricObjects.dat文件中
RandomCreateGeometricObject(new File("GeometricObjects.dat"));
// 从GeometricObjects.dat文件中读取对象,并输出每个对象的基本信息、周长和面积
GeometricObject[] objects = readFromFile(new File("GeometricObjects.dat"));
for (GeometricObject obj : objects) {
System.out.println("Object: " + obj.getClass().getSimpleName());
System.out.println("Perimeter: " + obj.getPerimeter());
System.out.println("Area: " + obj.getArea());
obj.draw();
System.out.println();
}
// 测试Rectangle类的方法
GeometricObject rect = new Rectangle(5, 10);
System.out.println("Object: " + rect.getClass().getSimpleName());
System.out.println("Perimeter: " + rect.getPerimeter());
System.out.println("Area: " + rect.getArea());
rect.draw();
}
// 随机生成Circle和Triangle类对象,并将对象序列化到指定文件中
public static boolean RandomCreateGeometricObject(File f) {
try {
Random rand = new Random();
ObjectOutputStream out = new ObjectOutputStream(new FileOutputStream(f));
for (int i = 0; i < 10; i++) {
if (rand.nextBoolean()) {
Circle c = new Circle(rand.nextDouble() * 10);
c.writeToFile(f);
} else {
Triangle t = new Triangle(rand.nextDouble() * 10, rand.nextDouble() * 10, rand.nextDouble() * 10);
t.writeToFile(f);
}
}
out.close();
return true;
} catch (IOException e) {
e.printStackTrace();
return false;
}
}
// 从指定文件中读取对象,并返回一个GeometricObject对象数组
public static GeometricObject[] readFromFile(File f) {
try {
ObjectInputStream in = new ObjectInputStream(new FileInputStream(f));
GeometricObject[] objects = new GeometricObject[10];
for (int i = 0; i < 10; i++) {
objects[i] = (GeometricObject) in.readObject();
}
in.close();
return objects;
} catch (IOException | ClassNotFoundException e) {
e.printStackTrace();
return null;
}
}
}
```
对于版本3,我们需要使用JavaFX来实现GUI界面。界面上应该有下拉框或单选按钮来选择要绘制的几何图形类型,以及输入框来输入相应的参数。当用户点击绘制按钮时,应该根据输入的参数来创建相应的几何图形对象,并在画布上绘制出来。同时,应该显示该几何图形的周长和面积。
由于JavaFX相关知识较多,这里无法详细讲解。建议您参考JavaFX官方文档或在线教程学习。
阅读全文
相关推荐
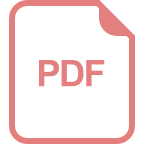
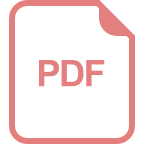
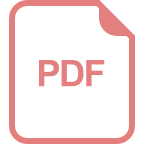
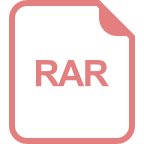
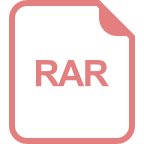
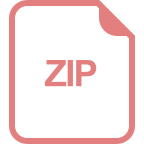
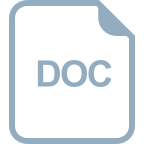
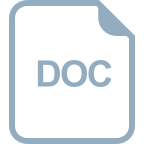
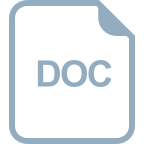
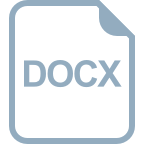
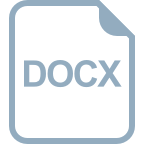
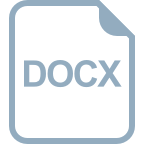