Python属性探秘:从attribute到property
"本文主要探讨了Python中的attribute与property的区别,并引用了Webster对这两个词的解释,同时结合Python编程语言的特点进行了详细阐述。在Python中,attribute与property的使用和概念有所不同,property通常用于描述类的本质特征,而attribute则更偏向于表示对象的具体状态。" 在Python编程中,attribute和property都是描述对象特性的关键概念,但它们之间存在着微妙的差异。首先,让我们理解一下Webster对于"property"和"attribute"的定义: - "property"是指属于事物本质的特性,它可以用来定义或描述一个类型或种类。在面向对象编程中,property通常被看作是类的核心特征,用于定义类的行为和状态。 - "attribute"则更像是一个修饰词,用于限制、识别、特定化、描述或补充其所修饰的单词的含义。在面向对象编程中,它更多地表示对象的某个具体状态或者附加信息。 Python中的attribute包括所有数据属性(data attribute)和方法,它们可以分为两类:class attribute和instance attribute。Class attribute属于类本身,是所有实例共享的,而instance attribute是每个实例独有的。这些attribute都存储在对象的`__dict__`属性中,可以用来查看和修改对象的状态。 ```python class MyClass: class_attribute = "shared_value" def __init__(self, instance_attribute): self.instance_attribute = instance_attribute my_object = MyClass("unique_value") print(my_object.__dict__) # 输出: {'instance_attribute': 'unique_value'} ``` 在Python中,property是一个内置的描述符(descriptor),它允许我们创建具有存取器和设值器的方法,从而控制对类属性的访问。通过使用@property装饰器,我们可以将对attribute的直接修改转化为调用设值器函数,这使得在修改属性时可以添加验证、计算或其他逻辑。 ```python class ComplexNumber: def __init__(self, real, imag): self._real = real self._imag = imag @property def real(self): return self._real @real.setter def real(self, value): if not isinstance(value, (int, float)): raise ValueError("Real part must be a number.") self._real = value @property def imag(self): return self._imag @imag.setter def imag(self, value): if not isinstance(value, (int, float)): raise ValueError("Imaginary part must be a number.") self._imag = value try: complex_number = ComplexNumber(1, "two") except ValueError as e: print(e) # 输出: Imaginary part must be a number. ``` 在这个例子中,`ComplexNumber`类使用了property来保护其内部的`_real`和`_imag`属性,确保它们始终接收数值类型的值。当尝试设置一个非数值类型的值时,会抛出一个`ValueError`。 attribute是对象状态的直接反映,而property是Python提供的一种机制,用于在访问或修改attribute时添加控制逻辑,使得代码更加健壮和安全。理解这两个概念的区别,有助于更好地设计和实现面向对象的Python程序。
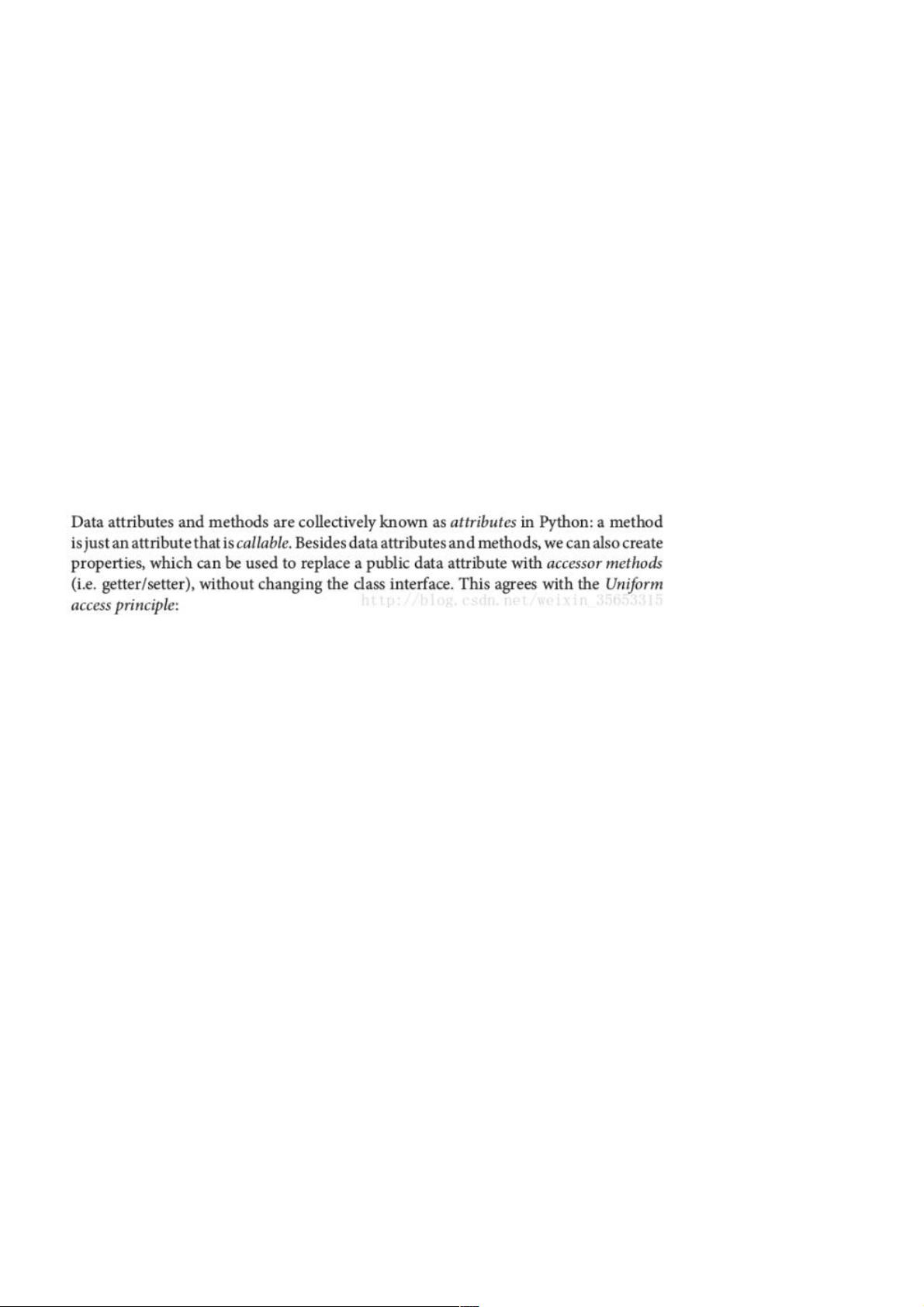
下载后可阅读完整内容,剩余4页未读,立即下载
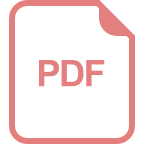



















- 粉丝: 8
- 资源: 928
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

最新资源
- OptiX传输试题与SDH基础知识
- C++Builder函数详解与应用
- Linux shell (bash) 文件与字符串比较运算符详解
- Adam Gawne-Cain解读英文版WKT格式与常见投影标准
- dos命令详解:基础操作与网络测试必备
- Windows 蓝屏代码解析与处理指南
- PSoC CY8C24533在电动自行车控制器设计中的应用
- PHP整合FCKeditor网页编辑器教程
- Java Swing计算器源码示例:初学者入门教程
- Eclipse平台上的可视化开发:使用VEP与SWT
- 软件工程CASE工具实践指南
- AIX LVM详解:网络存储架构与管理
- 递归算法解析:文件系统、XML与树图
- 使用Struts2与MySQL构建Web登录验证教程
- PHP5 CLI模式:用PHP编写Shell脚本教程
- MyBatis与Spring完美整合:1.0.0-RC3详解

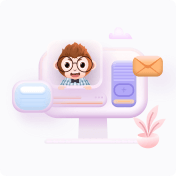
