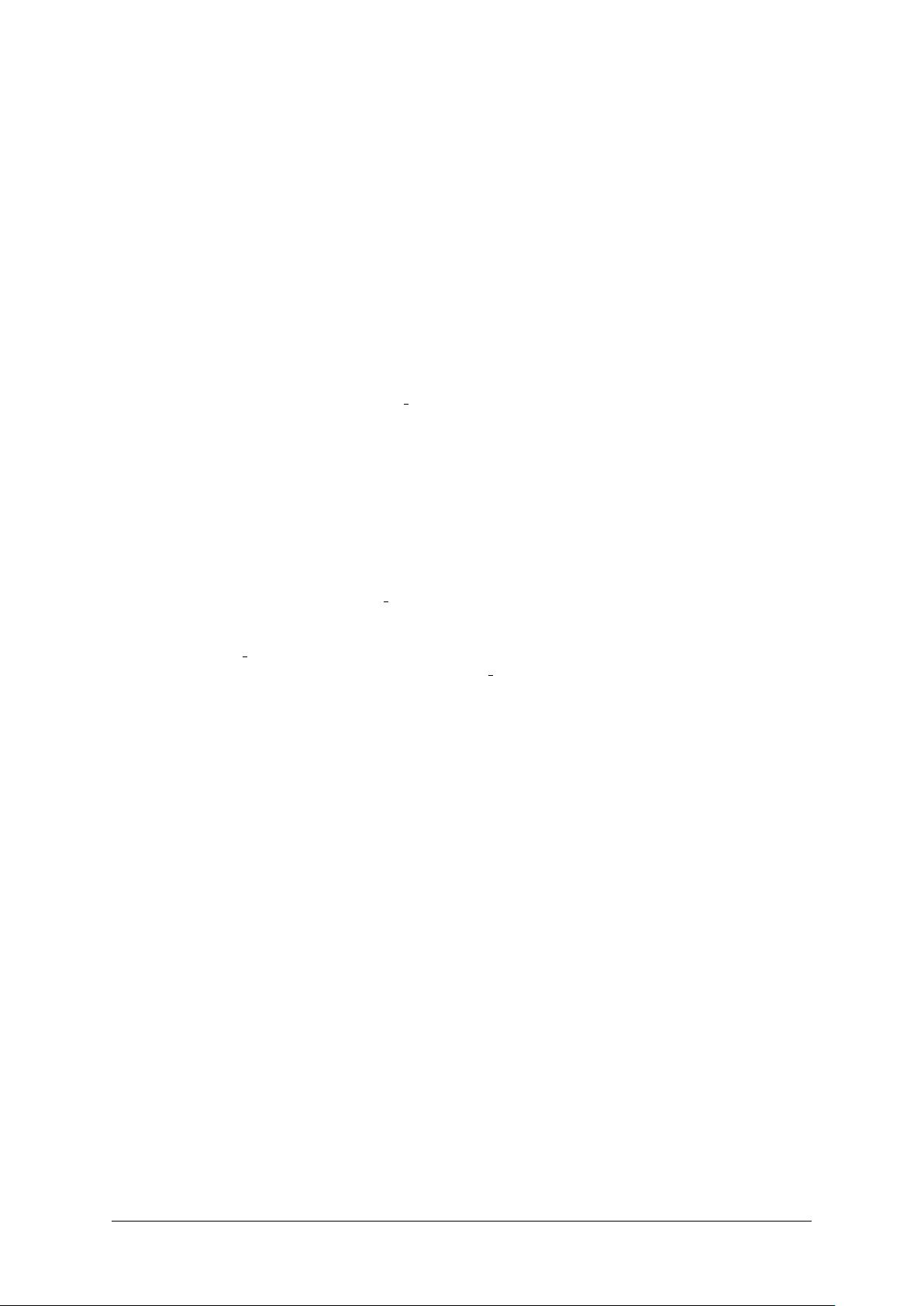
The @classmethod form is a function decorator – see the description of function definitions in chapter 7
of the Python Reference Manual for details.
It can be called either on the class (such as C.f()) or on an instance (such as C().f()). The instance is
ignored except for its class. If a class method is called for a derived class, the derived class object is passed
as the implied first argument.
Class methods are different than C
++
or Java static methods. If you want those, see staticmethod() in
this section.
For more information on class methods, consult the documentation on the standard type hierarchy in chapter
3 of the Python Reference Manual (at the bottom). New in version 2.2. Changed in version 2.4: Function
decorator syntax added.
cmp(x, y)
Compare the two objects x and y and return an integer according to the outcome. The return value is negative
if x < y, zero if x == y and strictly positive if x > y.
compile(string, filename, kind[, flags[, dont inherit ]])
Compile the string into a code object. Code objects can be executed by an exec statement or evaluated by
a call to eval(). The filename argument should give the file from which the code was read; pass some
recognizable value if it wasn’t read from a file (’<string>’ is commonly used). The kind argument spec-
ifies what kind of code must be compiled; it can be ’exec’ if string consists of a sequence of statements,
’eval’ if it consists of a single expression, or ’single’ if it consists of a single interactive statement
(in the latter case, expression statements that evaluate to something else than None will be printed).
When compiling multi-line statements, two caveats apply: line endings must be represented by a single
newline character (’\n’), and the input must be terminated by at least one newline character. If line
endings are represented by ’\r\n’, use the string replace() method to change them into ’\n’.
The optional arguments flags and dont inherit (which are new in Python 2.2) control which future statements
(see PEP 236) affect the compilation of string. If neither is present (or both are zero) the code is compiled
with those future statements that are in effect in the code that is calling compile. If the flags argument is
given and dont inherit is not (or is zero) then the future statements specified by the flags argument are used
in addition to those that would be used anyway. If dont inherit is a non-zero integer then the flags argument
is it – the future statements in effect around the call to compile are ignored.
Future statements are specified by bits which can be bitwise or-ed together to specify multiple statements.
The bitfield required to specify a given feature can be found as the compiler_flag attribute on the
_Feature instance in the __future__ module.
complex([real[, imag ]])
Create a complex number with the value real + imag*j or convert a string or number to a complex number.
If the first parameter is a string, it will be interpreted as a complex number and the function must be called
without a second parameter. The second parameter can never be a string. Each argument may be any
numeric type (including complex). If imag is omitted, it defaults to zero and the function serves as a
numeric conversion function like int(), long() and float(). If both arguments are omitted, returns
0j.
delattr(object, name)
This is a relative of setattr(). The arguments are an object and a string. The string must be the name
of one of the object’s attributes. The function deletes the named attribute, provided the object allows it. For
example, delattr(x, ’foobar’) is equivalent to del x.foobar.
dict([mapping-or-sequence ])
Return a new dictionary initialized from an optional positional argument or from a set of keyword argu-
ments. If no arguments are given, return a new empty dictionary. If the positional argument is a mapping
object, return a dictionary mapping the same keys to the same values as does the mapping object. Otherwise
the positional argument must be a sequence, a container that supports iteration, or an iterator object. The
elements of the argument must each also be of one of those kinds, and each must in turn contain exactly two
objects. The first is used as a key in the new dictionary, and the second as the key’s value. If a given key is
seen more than once, the last value associated with it is retained in the new dictionary.
If keyword arguments are given, the keywords themselves with their associated values are added as items
to the dictionary. If a key is specified both in the positional argument and as a keyword argument, the value
2.1. Built-in Functions 5