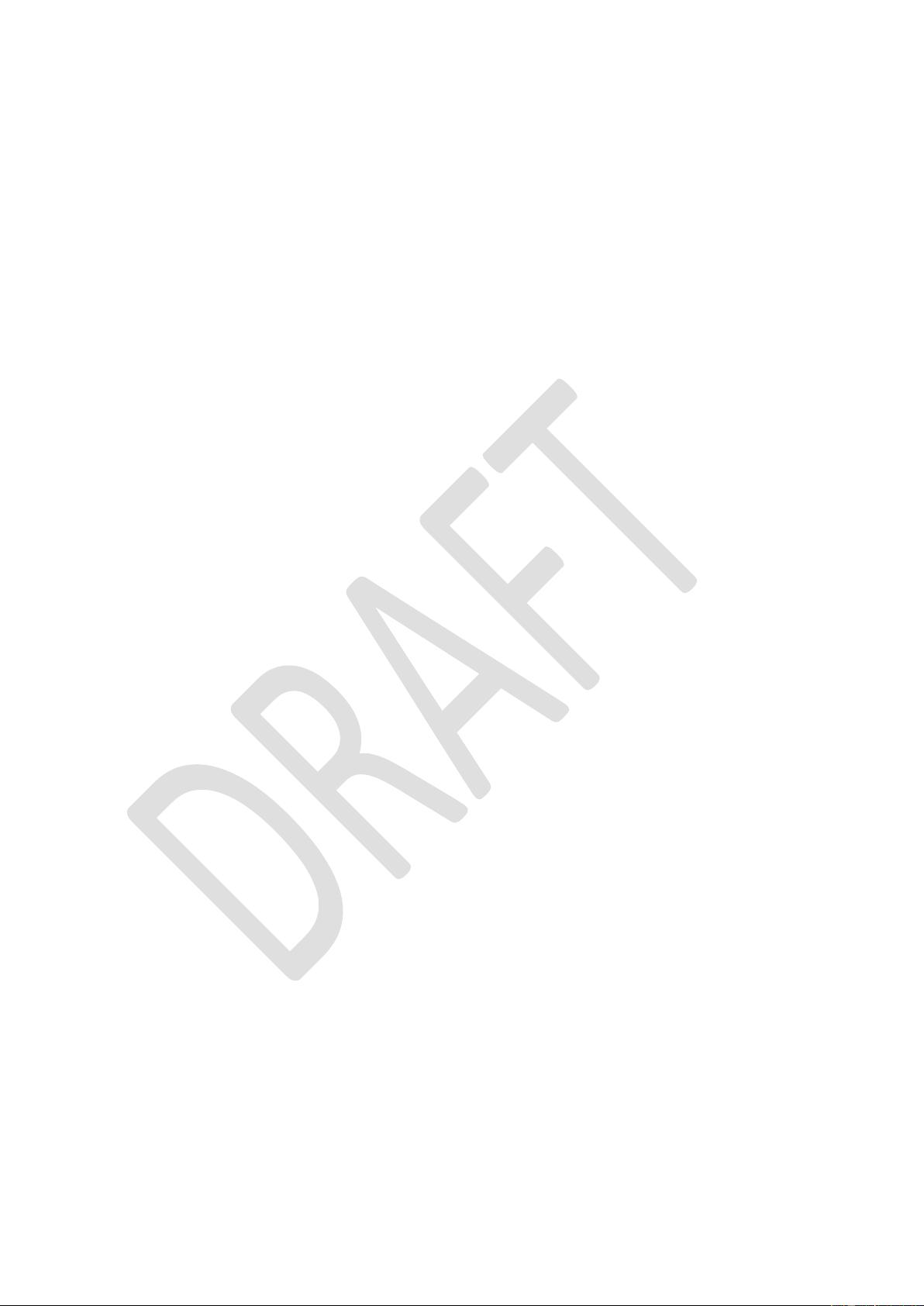
- 2 -
Some of the facilities of ECMAScript are similar to those used in other programming languages; in particular
Java, Self, and Scheme as described in:
Gosling, James, Bill Joy and Guy Steele. The Java
Language Specification. Addison Wesley Publishing
Co., 1996.
Ungar, David, and Smith, Randall B. Self: The Power of Simplicity. OOPSLA '87 Conference
Proceedings, pp. 227–241, Orlando, FL, October 1987.
IEEE Standard for the Scheme Programming Language. IEEE Std 1178-1990.
4.1 Web Scripting
A web browser provides an ECMAScript host environment for client-side computation including, for
instance, objects that represent windows, menus, pop-ups, dialog boxes, text areas, anchors, frames, history,
cookies, and input/output. Further, the host environment provides a means to attach scripting code to events
such as change of focus, page and image loading, unloading, error and abort, selection, form submission,
and mouse actions. Scripting code appears within the HTML and the displayed page is a combination of
user interface elements and fixed and computed text and images. The scripting code is reactive to user
interaction and there is no need for a main program.
A web server provides a different host environment for server-side computation including objects
representing requests, clients, and files; and mechanisms to lock and share data. By using browser -side and
server-side scripting together, it is possible to distribute computation between the client and server while
providing a customised user interface for a Web-based application.
Each Web browser and server that supports ECMAScript supplies its own host environment, completing the
ECMAScript execution environment.
4.2 Language Overview
The following is an informal overview of ECMAScript—not all parts of the language are described. This
overview is not part of the standard proper.
ECMAScript is object-based: basic language and host facilities are provided by objects, and an
ECMAScript program is a cluster of communicating objects. An ECMAScript object is a collection of
properties each with zero or more attributes that determine how each property can be used—for example,
when the Writable attribute for a property is set to false, any attempt by executed ECMAScript code to
change the value of the property fails. Properties are containers that hold other objects, primitive values, or
methods. A primitive value is a member of one of the following built-in types: Undefined, Null, Boolean,
Number, and String; an object is a member of the remaining built-in type Object; and a method is a
function associated with an object via a property.
ECMAScript defines a collection of built-in objects that round out the definition of ECMAScript entities.
These built-in objects include the Global object, the Object object, the Function object, the Array object,
the String object, the Boolean object, the Number object, the Math object, the Date object, the RegExp
object, the JSON object, and the Error objects Error, EvalError, RangeError, ReferenceError,
SyntaxError, TypeError and URIError.
ECMAScript also defines a set of built-in operators. ECMAScript operators include various unary
operations, multiplicative operators, additive operators, bitwise shift operators, relational operators,
equality operators, binary bitwise operators, binary logical operators, assignment operators, and the comma
operator.
ECMAScript syntax intentionally resembles Java syntax. ECMAScript syntax is relaxed to enable it to
serve as an easy-to-use scripting language. For example, a variable is not required to have its type declared
nor are types associated with properties, and defined functions are not required to have their declarations
appear textually before calls to them.
4.2.1 Objects
ECMAScript does not contain classes such as those in C++, Smalltalk, or Java. Instead objects may be
created in various ways including via a literal notation or via constructors which create objects by
executing code that allocates storage for the objects and initialises all or part of them by assigning initial
values to their properties. Each constructor has a property named ―prototype‖ that is used to