SpringMVC加载Properties配置文件实战解析

"本文将详细介绍在SpringMVC框架中加载配置Properties文件的多种方法,适合对SpringMVC感兴趣的开发者参考学习。" 在SpringMVC框架中,经常需要从Properties文件中读取配置信息,以便在运行时动态设置数据库连接、应用参数等。以下是SpringMVC加载Properties文件的几种常见方式: 1. 通过`context:property-placeholder`实现配置文件加载 这是最常用的方法,通过在Spring的配置文件(如`spring.xml`)中引入`context`命名空间,并使用`property-placeholder`标签来指定Properties文件的位置。步骤如下: 1.1 引入`context`命名空间: ```xml <beans xmlns="http://www.springframework.org/schema/beans" xmlns:context="http://www.springframework.org/schema/context" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-4.0.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd"> ``` 1.2 在`spring.xml`中配置`property-placeholder`: ```xml <context:property-placeholder location="classpath:jdbc.properties"/> ``` 这里的`location`属性指定了Properties文件的路径,`classpath:`表示在类路径下查找。 1.3 创建`jdbc.properties`文件,例如: ``` jdbc_driverClassName=com.mysql.jdbc.Driver jdbc_url=jdbc:mysql://localhost/testdb?useUnicode=true&characterEncoding=utf8 jdbc_username=root jdbc_password=123456 ``` 1.4 在其他配置文件(如`spring-mybatis.xml`)中,可以使用`${}`来引用Properties文件中的值: ```xml <bean id="dataSource" class="org.apache.commons.dbcp.BasicDataSource" destroy-method="close"> <property name="driverClassName" value="${jdbc_driverClassName}"/> <property name="url" value="${jdbc_url}"/> <property name="username" value="${jdbc_username}"/> <property name="password" value="${jdbc_password}"/> </bean> ``` 2. 使用`@Value`注解注入属性值 Spring框架提供了`@Value`注解,可以直接在Java类的字段或方法参数上使用,从Properties文件中读取配置值。例如,在Service类中: ```java @Component public class MyService { @Value("${jdbc.url}") private String jdbcUrl; // ... } ``` 这样,`jdbc.url`的值就会被注入到`jdbcUrl`字段中。 3. 使用`Environment`接口 在Spring Bean中,可以通过依赖注入`Environment`接口来获取Properties文件中的值。例如: ```java @Component public class MyService { @Autowired private Environment env; public void init() { String jdbcUrl = env.getProperty("jdbc.url"); // ... } } ``` `Environment`接口提供了`getProperty`方法,可以方便地获取Properties文件中的任意配置项。 4. 使用`PropertySourcesPlaceholderConfigurer` 在Spring配置文件中,可以定义一个`PropertySourcesPlaceholderConfigurer` bean来处理Properties文件。这将自动解析所有`@Value`注解: ```xml <bean id="propertyConfigurer" class="org.springframework.context.support.PropertySourcesPlaceholderConfigurer"> <property name="locations"> <list> <value>classpath:jdbc.properties</value> </list> </property> </bean> ``` 这样,`jdbc.properties`文件的配置就可以在所有需要的地方被解析和注入。 以上就是SpringMVC加载Properties文件的几种常见方法,可以根据项目的具体需求选择合适的方式。这些方法不仅限于SpringMVC,也适用于Spring Boot和其他Spring框架。通过灵活地加载和使用配置文件,开发者可以更好地管理应用程序的配置,提高代码的可维护性和灵活性。
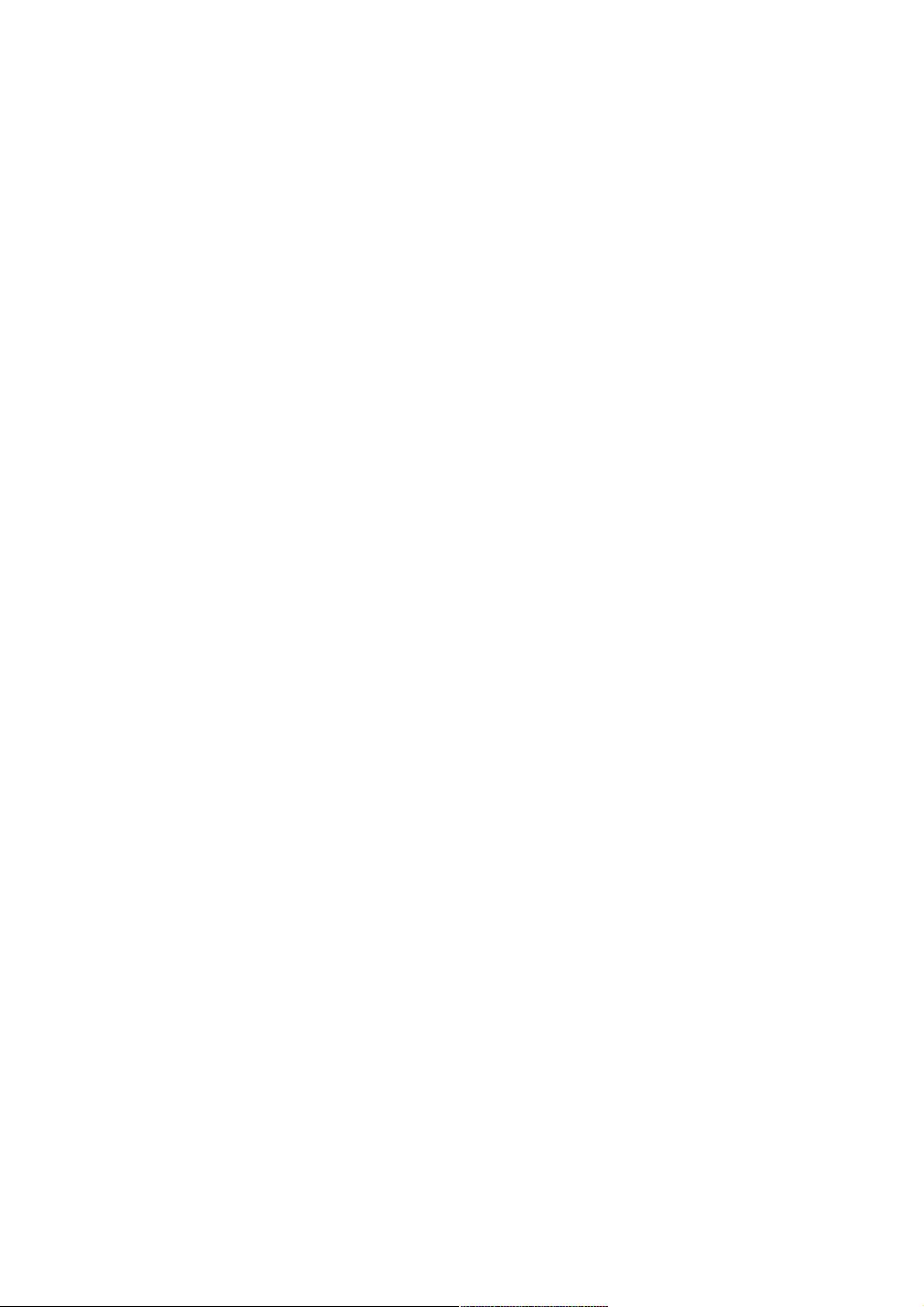
下载后可阅读完整内容,剩余3页未读,立即下载
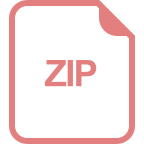

















- 粉丝: 3
- 资源: 943
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

最新资源
- OptiX传输试题与SDH基础知识
- C++Builder函数详解与应用
- Linux shell (bash) 文件与字符串比较运算符详解
- Adam Gawne-Cain解读英文版WKT格式与常见投影标准
- dos命令详解:基础操作与网络测试必备
- Windows 蓝屏代码解析与处理指南
- PSoC CY8C24533在电动自行车控制器设计中的应用
- PHP整合FCKeditor网页编辑器教程
- Java Swing计算器源码示例:初学者入门教程
- Eclipse平台上的可视化开发:使用VEP与SWT
- 软件工程CASE工具实践指南
- AIX LVM详解:网络存储架构与管理
- 递归算法解析:文件系统、XML与树图
- 使用Struts2与MySQL构建Web登录验证教程
- PHP5 CLI模式:用PHP编写Shell脚本教程
- MyBatis与Spring完美整合:1.0.0-RC3详解

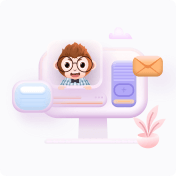
