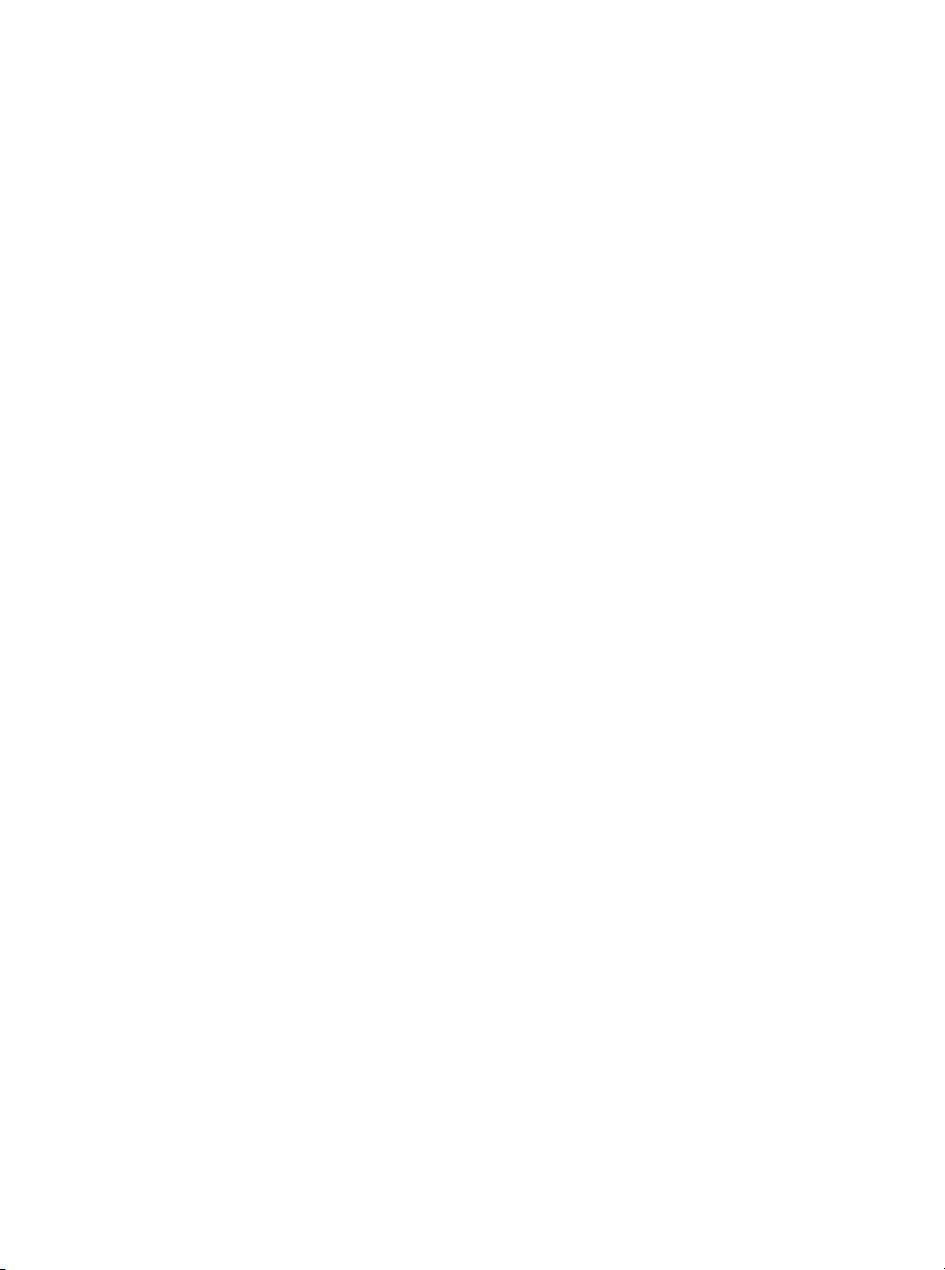
ptg11539604
Section 1.5 Types, Variables, and Arithmetic 7
complex<double> z = 1; // a complex number with double-precision floating-point scalars
complex<double> z2 {d1,d2};
complex<double> z3 = {1,2}; // the = is optional with { ... }
vector<int> v {1,2,3,4,5,6}; // a vector of ints
The = form is traditional and dates back to C, but if in doubt, use the general {}-list form. If nothing
else, it saves you from conversions that lose information:
int i1 = 7.2; // i1 becomes 7 (surpr ise?)
int i2 {7.2}; // error : floating-point to integer conversion
int i3 = {7.2}; // error : floating-point to integer conversion (the = is redundant)
Unfortunately, conversions that lose information, narrowing conversions, such as double to int and
int to char are allowed and implicitly applied. The problems caused by implicit narrowing conver-
sions is a price paid for C compatibility (§14.3).
A constant (§1.7) cannot be left uninitialized and a variable should only be left uninitialized in
extremely rare circumstances. Don’t introduce a name until you have a suitable value for it. User-
defined types (such as
string, vector, Matrix, Motor_controller, and Orc_warrior) can be defined to be
implicitly initialized (§4.2.1).
When defining a variable, you don’t actually need to state its type explicitly when it can be
deduced from the initializer:
auto b = true; // a bool
auto ch = 'x'; // a char
auto i = 123; // an int
auto d = 1.2; // a double
auto z = sqrt(y); // z has the type of whatever sqr t(y) retur ns
With auto, we use the = because there is no potentially troublesome type conversion involved.
We use
auto where we don’t hav e a specific reason to mention the type explicitly. ‘‘Specific
reasons’’ include:
• The definition is in a large scope where we want to make the type clearly visible to readers
of our code.
• We want to be explicit about a variable’s range or precision (e.g.,
double rather than float).
Using
auto, we avoid redundancy and writing long type names. This is especially important in
generic programming where the exact type of an object can be hard for the programmer to know
and the type names can be quite long (§10.2).
In addition to the conventional arithmetic and logical operators, C++ offers more specific opera-
tions for modifying a variable:
x+=y // x = x+y
++x // increment: x = x+1
x−=y // x = x-y
−−x // decrement: x = x-1
x∗=y // scaling: x = x*y
x/=y // scaling: x = x/y
x%=y // x = x%y
These operators are concise, convenient, and very frequently used.