"单链表的插入和删除实验报告:对线性表的逻辑结构和链式存储结构进行探究与分析"
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
本实验旨在通过对单链表的插入和删除操作进行实验,深入了解和掌握线性表的逻辑结构和链式存储结构,并掌握单链表的基本算法及相关的时间性能分析。 实验要求建立一个数据域定义为字符串的单链表,在链表中不允许有重复的字符串,并根据输入的字符串,先找到相应的结点,然后进行删除操作。 实验源代码如下所示: ```C #include <stdio.h> #include <stdlib.h> #include <string.h> typedef struct Node { char data[100]; struct Node *next; } Node; Node *createList() { Node *head = (Node *)malloc(sizeof(Node)); head->next = NULL; return head; } void insertNode(Node *head, char *str) { Node *p = head; while (p->next != NULL) { p = p->next; } Node *newNode = (Node *)malloc(sizeof(Node)); strcpy(newNode->data, str); newNode->next = NULL; p->next = newNode; } void deleteNode(Node *head, char *str) { Node *p = head->next; Node *pre = head; while (p != NULL) { if (strcmp(p->data, str) == 0) { pre->next = p->next; free(p); p = pre->next; } else { pre = p; p = p->next; } } } void displayList(Node *head) { Node *p = head->next; while (p != NULL) { printf("%s ", p->data); p = p->next; } printf("\n"); } int main() { Node *head = createList(); insertNode(head, "apple"); insertNode(head, "banana"); insertNode(head, "orange"); insertNode(head, "apple"); printf("The original list is: "); displayList(head); deleteNode(head, "apple"); printf("After deleting 'apple', the list is: "); displayList(head); return 0; } ``` 以上是本次单链表的插入和删除实验的程序源代码。在这次实验中,我们建立了一个数据域定义为字符串的单链表,并通过插入和删除操作,成功实现了对单链表的操作。通过该实验,我们深入了解了单链表的基本算法及相关的时间性能分析,加深了对线性表的逻辑结构和链式存储结构的掌握,为进一步深入学习和应用数据结构打下了坚实的基础。
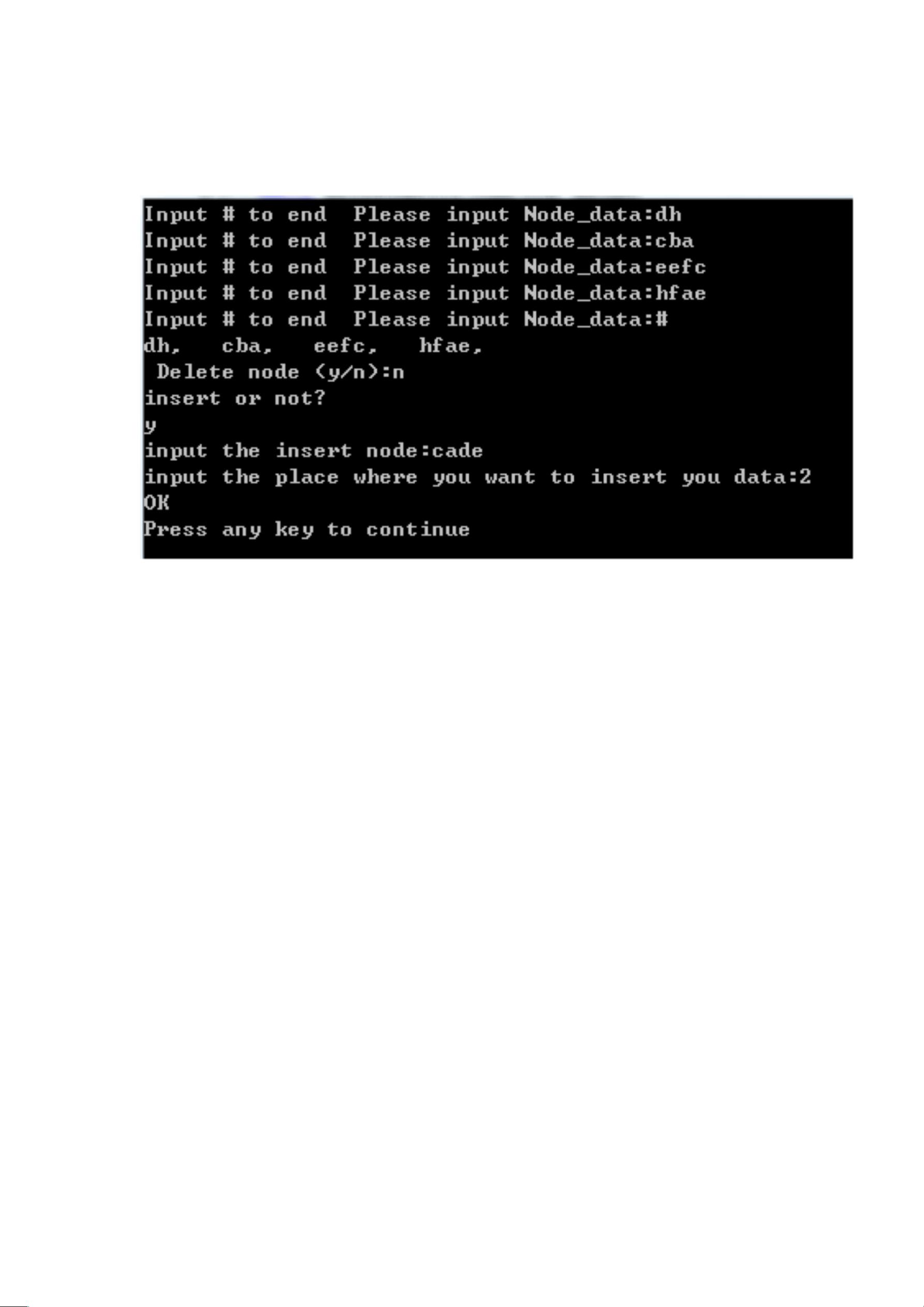
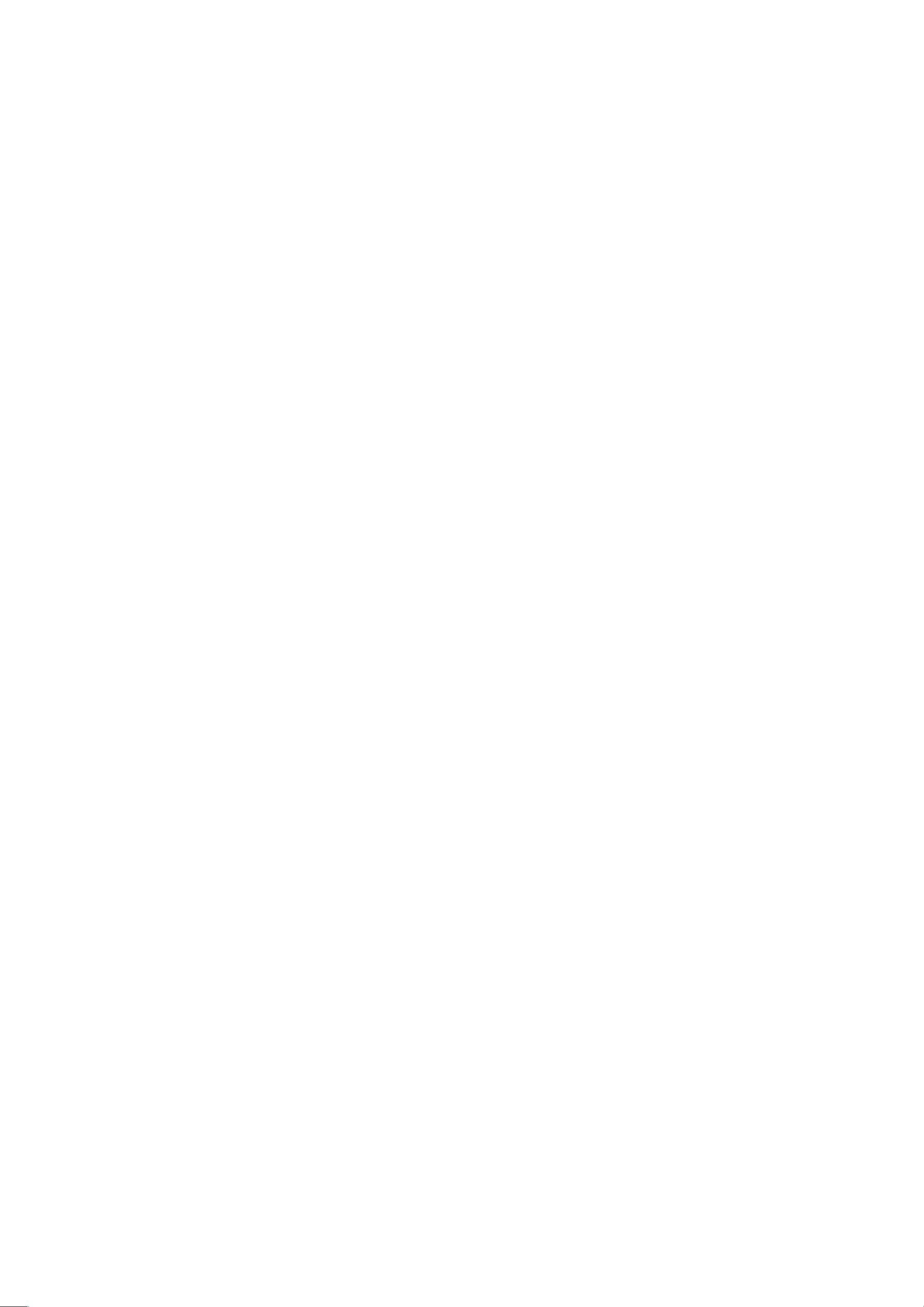
剩余40页未读,继续阅读
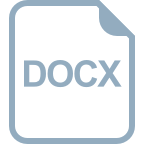
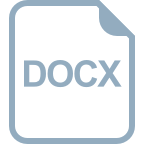

















- 粉丝: 6670
- 资源: 3万+
我的内容管理 收起
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

会员权益专享
最新资源
- zigbee-cluster-library-specification
- JSBSim Reference Manual
- c++校园超市商品信息管理系统课程设计说明书(含源代码) (2).pdf
- 建筑供配电系统相关课件.pptx
- 企业管理规章制度及管理模式.doc
- vb打开摄像头.doc
- 云计算-可信计算中认证协议改进方案.pdf
- [详细完整版]单片机编程4.ppt
- c语言常用算法.pdf
- c++经典程序代码大全.pdf
- 单片机数字时钟资料.doc
- 11项目管理前沿1.0.pptx
- 基于ssm的“魅力”繁峙宣传网站的设计与实现论文.doc
- 智慧交通综合解决方案.pptx
- 建筑防潮设计-PowerPointPresentati.pptx
- SPC统计过程控制程序.pptx

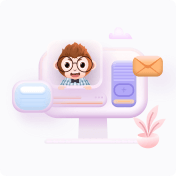

评论0