没有合适的资源?快使用搜索试试~ 我知道了~
首页ARM汇编入门教程:精要解析与实战
ARM汇编编程入门教程深入浅出地探讨了32位ARM处理器家族的底层原理和实践。作者G.Agosta以米兰理工学院的身份,于2011年12月3日撰写了这篇15页的指南,旨在帮助读者掌握ARM汇编语言的基础知识。 首先,文章介绍了学习ARM汇编的前置条件,这些可能包括对计算机体系结构的基本理解,特别是ARM架构的特性和工作方式。ARM架构因其低功耗和广泛的应用而在嵌入式系统中占据重要地位,对硬件级别的控制能力是学习者必备的基础。 在实际编程部分,作者提供了一个组装程序模板,展示了如何构造一个基本的ARM汇编程序,包括如何使用GNU汇编器编写指令。这涵盖了指令集的关键元素,如算术逻辑指令(ALU)的操作,如加、减、乘和除等,以及分支指令,用于控制程序流程的转移。 文章还详细讲解了如何将条件语句(如if-else和switch)转换为机器码,以及循环结构(while和do-while)的实现,这些都是程序控制结构的核心内容。此外,通过学习load和store指令,读者能理解如何与内存进行数据交互,这对于理解和优化程序性能至关重要。 接下来,章节转向函数调用和返回机制。在ARM汇编中,理解程序运行时架构以及栈的作用至关重要,因为函数调用涉及参数传递、局部变量管理和返回地址的管理。这部分内容深入剖析了如何在硬件层面实现函数调用,以及堆栈在执行上下文切换中的作用。 这篇教程不仅为初学者提供了入门ARM汇编的坚实基础,也对进阶开发者在理解和优化性能方面具有实用价值。通过阅读并实践这些内容,读者能够建立起从高级语言到硬件级编程的桥梁,进一步提升自己的技术能力。
资源详情
资源推荐
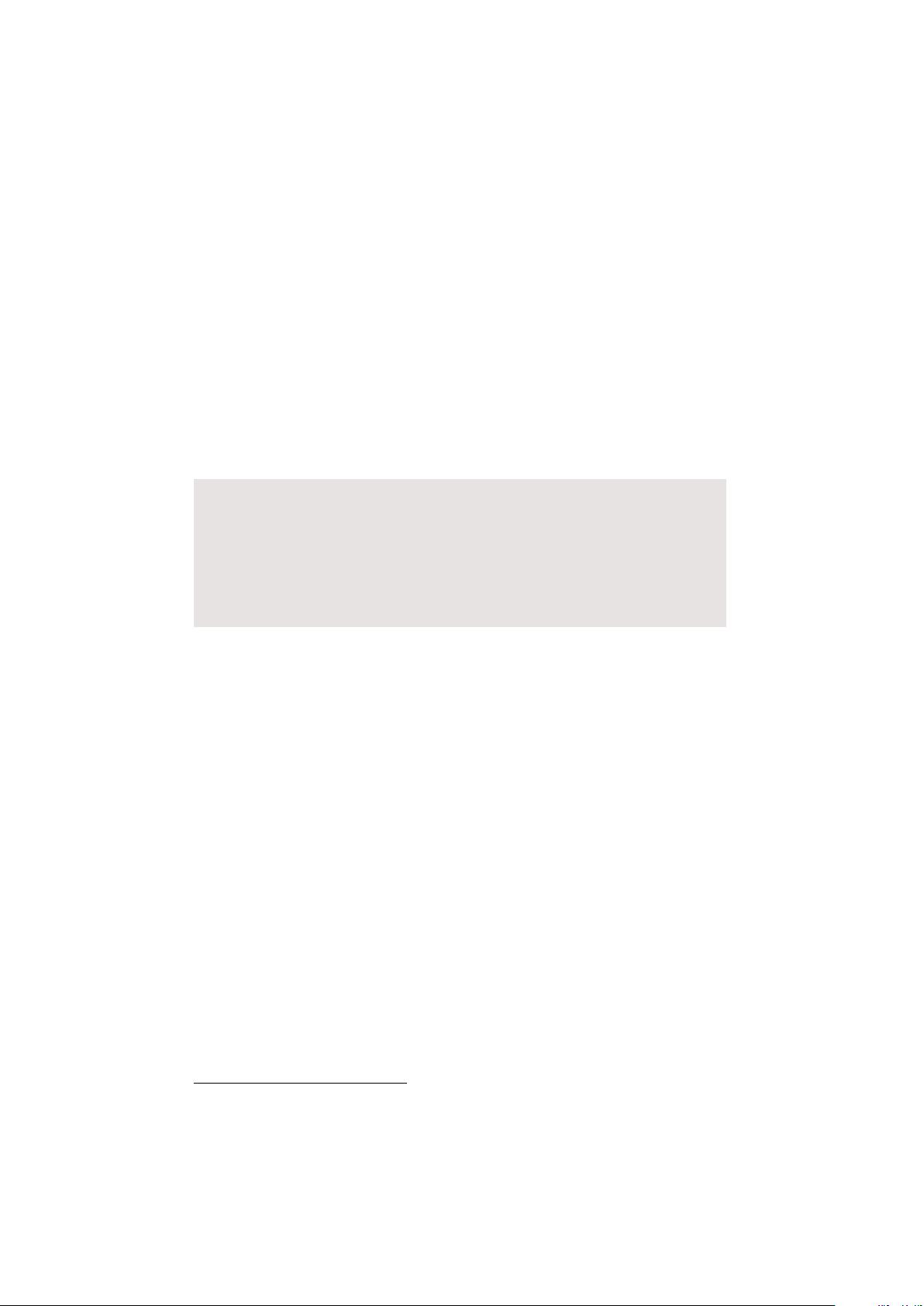
2 A First Assembly Program
In this section, we will write a very simple assembly program, which can be
used as a template for others, compile and link it, and execute it. To do so,
it is assumed that you have installed a Qemu ARM virtual machine, using the
instructions provided on my website.
3
Assembler languages only define instructions and hardware resources. To
drive the assembly and linking processes, we also need to use directives, which
are interpreted by the assembler. We will use the GNU Assembler (as), with
its specific directives.
2.1 Assembler Program Template
Here is the simplest program we can write in the ARM assembler language, one
that immediately ends, returning control to the caller.
1 .arm /* Specify this is an ARM 32 - bit program */
2 .text /* Start of the program code section */
3 .global main /* declares the main ident i fier */
4 .type main , % functi o n
5 main : /* Address of the main function */
6 /* Program code would go here */
7 BX LR /* Return to the caller */
8 .end /* End of the program */
The .arm directive specifies that this program is an ARM 32-bit assembly
code. The ARM architecture includes other types of programs (which we will
not see here), so this directive is needed for the Assembler to decide how to
assemble the program.
The .text directive indicates the start of a section of the assembly program
that contains code. Other sections could contain, e.g., the definition of global
data (i.e., global variables).
The .global main line specifies that main is a globally visible identifier,
which can be referred to from outside this file
4
This is necessary, because main
is the program entry point, and the corresponding function will be invoked from
outside the program!
.type main, %function specifies that the main identifier refers to a func-
tion. More precisely, it is a label indicating the address of the first instruction
of the main function.
main: BX LR is the first (and only) instruction of this program. It is prefixed
with a label, which allows to refer to the instruction address from other points
in the same compilation unit, or other units if, as in this case, the symbol main
is defined as a global identifier.
The .end directive specifies the end of the program.
2.2 Using the GNU Assembler
The GNU Assembler can be invoked as a standalone program, with a line such
as:
3
http://home.dei.polimi.it/agosta
4
More precisely, outside this compilation unit.
3
剩余14页未读,继续阅读
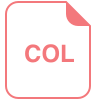
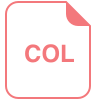















huangyime
- 粉丝: 0
- 资源: 6
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

最新资源
- AirKiss技术详解:无线传递信息与智能家居连接
- Hibernate主键生成策略详解
- 操作系统实验:位示图法管理磁盘空闲空间
- JSON详解:数据交换的主流格式
- Win7安装Ubuntu双系统详细指南
- FPGA内部结构与工作原理探索
- 信用评分模型解析:WOE、IV与ROC
- 使用LVS+Keepalived构建高可用负载均衡集群
- 微信小程序驱动餐饮与服装业创新转型:便捷管理与低成本优势
- 机器学习入门指南:从基础到进阶
- 解决Win7 IIS配置错误500.22与0x80070032
- SQL-DFS:优化HDFS小文件存储的解决方案
- Hadoop、Hbase、Spark环境部署与主机配置详解
- Kisso:加密会话Cookie实现的单点登录SSO
- OpenCV读取与拼接多幅图像教程
- QT实战:轻松生成与解析JSON数据
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


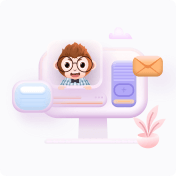
安全验证
文档复制为VIP权益,开通VIP直接复制
