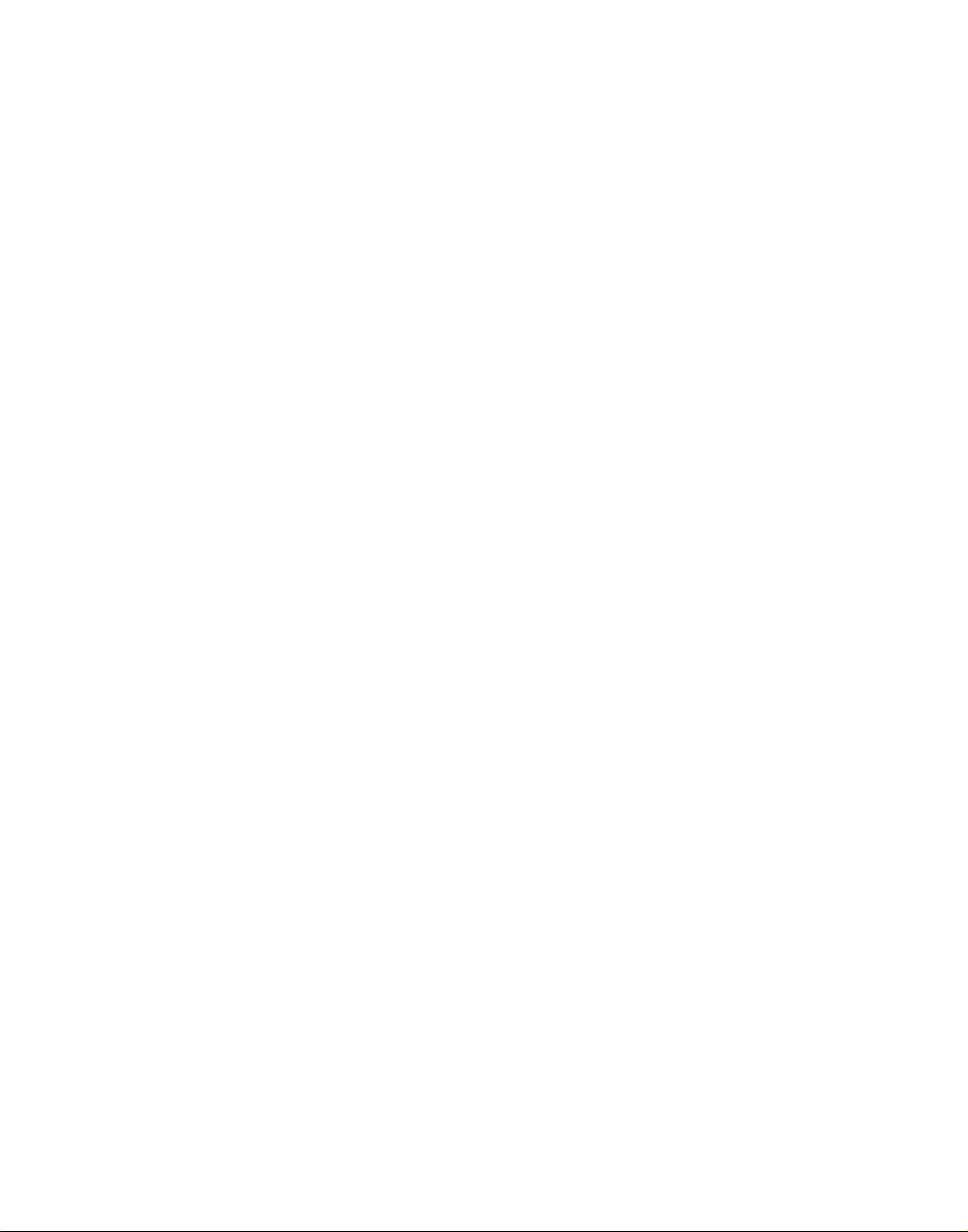
ptg
Structure of This Book
This book is divided into the following chapters.
Chapter 1 introduces the hardware and software concepts that will be encountered
in the rest of the book. The chapter gives an overview of the internals of processors. It is
not necessarily critical for the reader to understand how hardware works before they can
write programs that utilize multicore systems. However, an understanding of the basics of
processor architecture will enable the reader to better understand some of the concepts
relating to application correctness, performance, and scaling that are presented later in
the book. The chapter also discusses the concepts of threads and processes.
Chapter 2 discusses profiling and optimizing applications. One of the book’s prem-
ises is that it is vital to understand where the application currently spends its time before
work is spent on modifying the application to use multiple cores. The chapter covers all
the leading contributors to performance over the application development cycle and dis-
cusses how performance can be improved.
Chapter 3 describes ways that multicore systems can be used to perform more work
per unit time or reduce the amount of time it takes to complete a single unit of work. It
starts with a discussion of virtualization where one new system can be used to replace
multiple older systems. This consolidation can be achieved with no change in the soft-
ware. It is important to realize that multicore systems represent an opportunity to change
the way an application works; they do not require that the application be changed. The
chapter continues with describing various patterns that can be used to write parallel
applications and discusses the situations when these patterns might be useful.
Chapter 4 describes sharing data safely between multiple threads. The chapter leads
with a discussion of data races, the most common type of correctness problem encoun-
tered in multithreaded codes. This chapter covers how to safely share data and synchro-
nize threads at an abstract level of detail. The subsequent chapters describe the operating
system–specific details.
Chapter 5 describes writing parallel applications using POSIX threads. This is the
standard implemented by UNIX-like operating systems, such as Linux, Apple’s OS X,
and Oracle’s Solaris. The POSIX threading library provides a number of useful building
blocks for writing parallel applications. It offers great flexibility and ease of development.
Chapter 6 describes writing parallel applications for Microsoft Windows using
Windows native threading. Windows provides similar synchronization and data sharing
primitives to those provided by POSIX. The differences are in the interfaces and require-
ments of these functions.
Chapter 7 describes opportunities and limitations of automatic parallelization pro-
vided by compilers. The chapter also covers the OpenMP specification, which makes it
relatively straightforward to write applications that take advantage of multicore processors.
Chapter 8 discusses how to write parallel applications without using the functional-
ity in libraries provided by the operating system or compiler. There are some good rea-
sons for writing custom code for synchronization or sharing of data. These might be for
xvii
Preface
Download at www.wowebook.com