SpringBoot异步任务与定时任务详解
"本文将介绍如何在SpringBoot中实现异步任务、定时任务和邮件服务,帮助开发者快速上手这些常见的后台处理需求。" 在SpringBoot框架中,处理异步任务、定时任务和邮件服务是非常常见且重要的功能。下面将详细阐述如何在SpringBoot项目中实现这些功能。 ### 异步任务 1. 启用异步注解:首先,我们需要在SpringBoot的主配置类上启用@EnableAsync注解,这将激活Spring的异步任务支持。 ```java import org.springframework.scheduling.annotation.EnableAsync; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; @SpringBootApplication @EnableAsync public class Application { public static void main(String[] args) { SpringApplication.run(Application.class, args); } } ``` 2. 创建异步服务:接下来,创建一个@Service类,并使用@Async注解标记希望异步执行的方法。例如,在`AsyncService`类中: ```java package com.kuang.springboot09test.service; import org.springframework.scheduling.annotation.Async; import org.springframework.stereotype.Service; @Service public class AsyncService { @Async public void hello() { try { Thread.sleep(3000); } catch (InterruptedException e) { e.printStackTrace(); } System.out.println("数据正在处理"); } } ``` 3. 调用异步方法:在Controller类中注入并调用这个异步服务,如`AsyncController`所示: ```java package com.kuang.springboot09test.controller; import com.kuang.springboot09test.service.AsyncService; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController; @RestController public class AsyncController { @Autowired private AsyncService asyncService; @RequestMapping("/async") public String asyncTask() { asyncService.hello(); return "任务已启动,页面可以立即响应"; } } ``` ### 定时任务 1. 引入Quartz或Cron:SpringBoot支持两种定时任务方式,一是使用Quartz库,二是通过Spring的@Scheduled和Cron表达式。这里以Cron为例。 2. 启用定时任务:在配置类上启用@EnableScheduling注解: ```java import org.springframework.scheduling.annotation.EnableScheduling; // ... @SpringBootApplication @EnableAsync @EnableScheduling public class Application { // ... } ``` 3. 创建定时任务服务:创建一个@Service类,并使用@Scheduled注解来指定定时任务。例如: ```java package com.kuang.springboot09test.service; import org.springframework.scheduling.annotation.Scheduled; import org.springframework.stereotype.Service; @Service public class ScheduledService { @Scheduled(cron = "0 0 2 * * ?") // 每天凌晨2点执行 public void dailyTask() { System.out.println("每天凌晨的任务开始执行"); // 这里执行你的日志分析等操作 } } ``` ### 邮件服务 1. 引入依赖:在pom.xml或build.gradle中添加Spring Boot的邮件服务依赖,例如Maven: ```xml <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-mail</artifactId> </dependency> ``` 2. 配置邮件服务:在application.properties或application.yml中配置邮件服务器的属性,如发件人、用户名、密码、SMTP服务器等。 3. 创建邮件服务:创建一个@Service类,使用JavaMailSender接口发送邮件。例如: ```java package com.kuang.springboot09test.service; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.mail.SimpleMailMessage; import org.springframework.mail.javamail.JavaMailSender; import org.springframework.stereotype.Service; @Service public class MailService { @Autowired private JavaMailSender javaMailSender; public void sendSimpleMail(String to, String subject, String text) { SimpleMailMessage message = new SimpleMailMessage(); message.setTo(to); message.setSubject(subject); message.setText(text); javaMailSender.send(message); } } ``` 4. 在Controller中调用邮件服务:在需要发送邮件的地方,调用邮件服务类的方法。 通过以上步骤,你可以在SpringBoot项目中轻松实现异步任务、定时任务和邮件服务,提高系统的响应速度和效率。记得根据实际需求调整配置和代码,以满足特定场景下的应用。

- 粉丝: 129
- 资源: 2
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

最新资源
- 构建Cadence PSpice仿真模型库教程
- VMware 10.0安装指南:步骤详解与网络、文件共享解决方案
- 中国互联网20周年必读:影响行业的100本经典书籍
- SQL Server 2000 Analysis Services的经典MDX查询示例
- VC6.0 MFC操作Excel教程:亲测Win7下的应用与保存技巧
- 使用Python NetworkX处理网络图
- 科技驱动:计算机控制技术的革新与应用
- MF-1型机器人硬件与robobasic编程详解
- ADC性能指标解析:超越位数、SNR和谐波
- 通用示波器改造为逻辑分析仪:0-1字符显示与电路设计
- C++实现TCP控制台客户端
- SOA架构下ESB在卷烟厂的信息整合与决策支持
- 三维人脸识别:技术进展与应用解析
- 单张人脸图像的眼镜边框自动去除方法
- C语言绘制图形:余弦曲线与正弦函数示例
- Matlab 文件操作入门:fopen、fclose、fprintf、fscanf 等函数使用详解

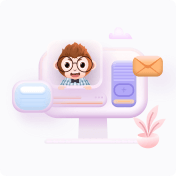
