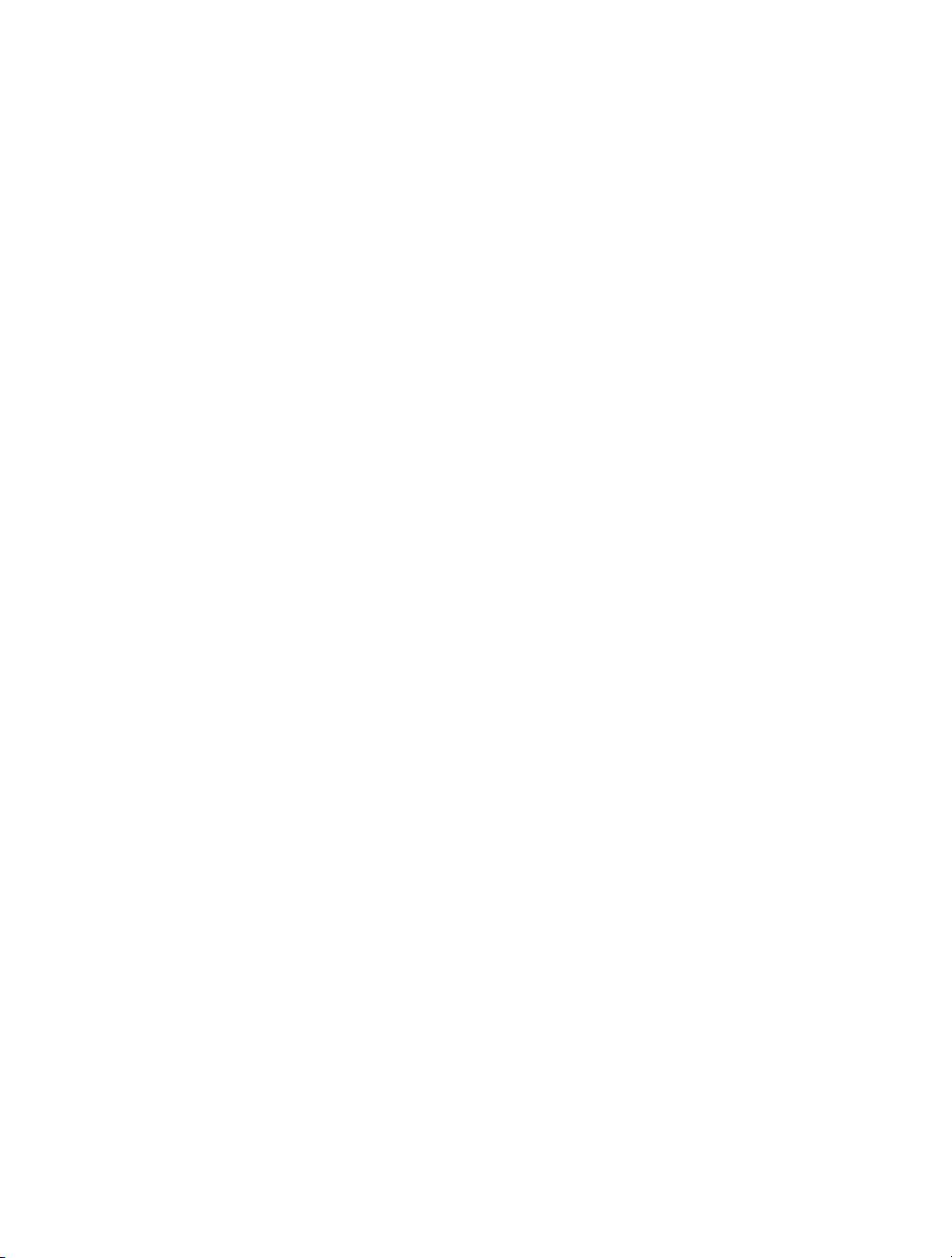
Contents in Detail xvii
Inheritance ..................................................... 172
The __init__() Method for a Child Class ........................... 172
Inheritance in Python 2.7 ..................................... 173
Defining Attributes and Methods for the Child Class .................. 174
Overriding Methods from the Parent Class ......................... 175
Instances as Attributes ....................................... 175
Modeling Real-World Objects ................................. 177
Exercise 9-6: Ice Cream Stand ................................. 178
Exercise 9-7: Admin ........................................ 178
Exercise 9-8: Privileges ...................................... 178
Exercise 9-9: Battery Upgrade ................................. 178
Importing Classes................................................. 179
Importing a Single Class ..................................... 179
Storing Multiple Classes in a Module............................. 180
Importing Multiple Classes from a Module ......................... 181
Importing an Entire Module ................................... 182
Importing All Classes from a Module ............................. 182
Importing a Module into a Module .............................. 183
Finding Your Own Workflow .................................. 184
Exercise 9-10: Imported Restaurant .............................. 184
Exercise 9-11: Imported Admin ................................. 184
Exercise 9-12: Multiple Modules ................................ 184
The Python Standard Library ......................................... 184
Exercise 9-13: OrderedDict Rewrite.............................. 186
Exercise 9-14: Dice ......................................... 186
Exercise 9-15: Python Module of the Week ........................ 186
Styling Classes................................................... 186
Summary ...................................................... 187
10
FILES AND EXCEPTIONS 189
Reading from a File ............................................... 190
Reading an Entire File ....................................... 190
File Paths ................................................ 191
Reading Line by Line ........................................ 193
Making a List of Lines from a File ............................... 194
Working with a File’s Contents ................................. 194
Large Files: One Million Digits ................................. 195
Is Your Birthday Contained in Pi? ............................... 196
Exercise 10-1: Learning Python ................................. 197
Exercise 10-2: Learning C .................................... 197
Writing to a File.................................................. 197
Writing to an Empty File ..................................... 197
Writing Multiple Lines ....................................... 198
Appending to a File......................................... 199
Exercise 10-3: Guest ........................................ 199
Exercise 10-4: Guest Book .................................... 199
Exercise 10-5: Programming Poll................................ 199
Exceptions...................................................... 200
Handling the ZeroDivisionError Exception ......................... 200
Using try-except Blocks ...................................... 200
Using Exceptions to Prevent Crashes ............................. 201