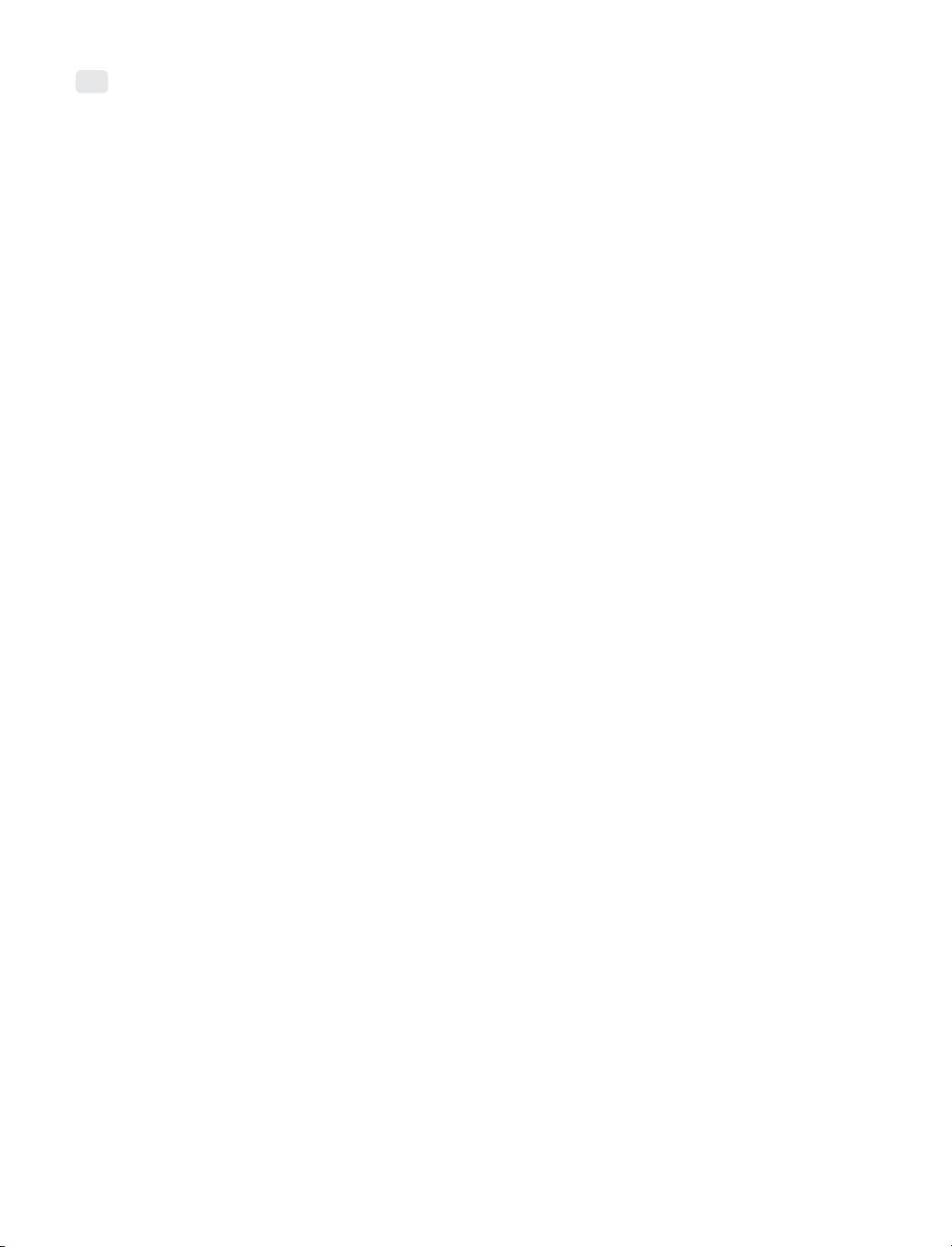
Object-Oriented Programming with C++
6
In the procedure-oriented programming system, procedures are dissociated from data and
are not a part of it. Instead, they receive structure variables or their addresses and work upon
them. The code design is centered around procedures. While this may sound obvious, this
programming pattern has its drawbacks.
The drawback with this programming pattern is that the data is not secure. It can be
manipulated by any procedure. Associated functions that were designed by the library
programmer do not have the exclusive rights to work upon the data. They are not a part of
the structure de¿ nition itself. Let us see why this is a problem.
Suppose the library programmer has de¿ ned a structure and its associated functions as
described above. Further, in order to perfect his/her creation, he/she has rigorously tested
the associated functions by calling them from small test applications. Despite his/her best
efforts, he/she cannot be sure that an application that uses the structure will be bug free. The
application program might modify the structure variables, not by the associated function he/
she has created, but by some code inadvertently written in the application program itself.
Compilers that implement the procedure-oriented programming system do not prevent
unauthorized functions from accessing/manipulating structure variables.
Now, let us look at the situation from the application programmer’s point of view. Consider
an application of around 25,000 lines (quite common in the real programming world), in
which variables of this structure have been used quite extensively. During testing, it is found
that the date being represented by one of these variables has become 29
th
February 1999!
The faulty piece of code that is causing this bug can be anywhere in the program. Therefore,
debugging will involve a visual inspection of the entire code (of 25000 lines!) and will not
be limited to the associated functions only.
The situation becomes especially grave if the execution of the code that is likely to corrupt
the data is conditional. For example,
if(<some condition>)
d.m++; //d is a variable of date structure… d.m may
//become 13!
The condition under which the bug-infested code executes may not arise during testing.
While distributing his/her application, the application programmer cannot be sure that it would
run successfully. Moreover, every new piece of code that accesses structure variables will
have to be visually inspected and tested again to ensure that it does not corrupt the members
of the structure. After all, compilers that implement procedure-oriented programming systems
do not prevent unauthorized functions from accessing/manipulating structure variables.
Let us think of a compiler that enables the library programmer to assign exclusive rights to
the associated functions for accessing the data members of the corresponding structure. If this
happens, then our problem is solved. If a function which is not one of the intended associated
functions accesses the data members of a structure variable, a compile-time error will result.
To ensure a successful compile of his/her application code, the application programmer will
be forced to remove those statements that access data members of structure variables. Thus,
the application that arises out of a successful compile will be the outcome of a piece of code
that is free of any unauthorized access to the data members of the structure variables used
therein. Consequently, if a run-time error arises, attention can be focused on the associated
library functions.
It is the lack of data security of procedure-oriented programming systems that led to object-
oriented programming systems (OOPS). This new system of programming is the subject of
our next discussion.