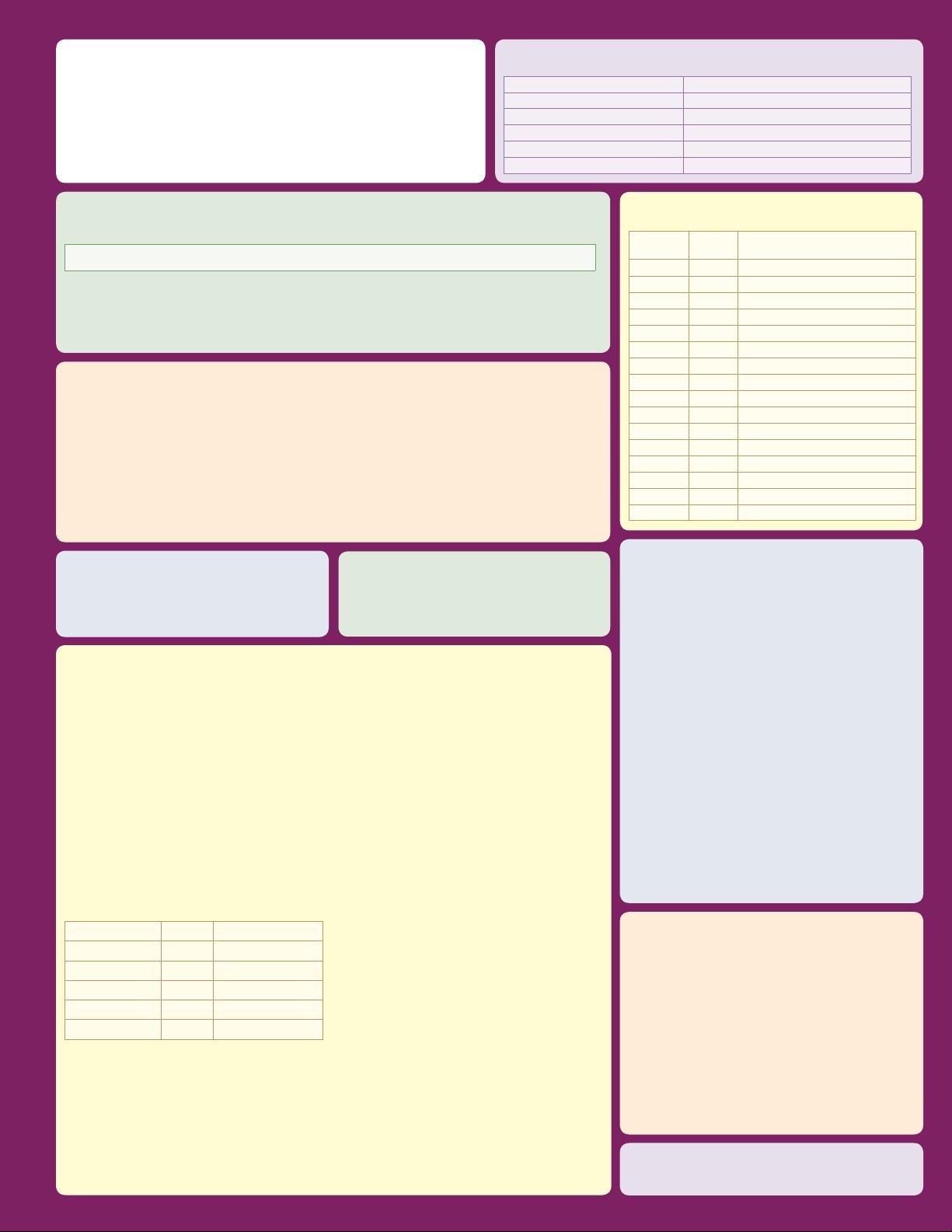
www.khronos.org/opengles©2010 Khronos Group - Rev. 0210
OpenGL ES 2.0 API Quick Reference Card
OpenGL
®
ES is a soware interface to graphics hardware. The interface
consists of a set of procedures and funcons that allow a programmer to specify
the objects and operaons involved in producing high-quality graphical images,
specically color images of three-dimensional objects.
• [n.n.n] refers to secons and tables in the OpenGL ES 2.0 specicaon.
• [n.n.n] refers to secons in the OpenGL ES Shading Language 1.0
specicaon.
Specicaons are available at www.opengl.org/registry/gles
Verces
Current Vertex State [2.7]
void VertexArib{1234}{f}(uint index, T values);
void VertexArib{1234}{f}v(uint index, T values);
Vertex Arrays [2.8]
Vertex data may be sourced from arrays that are stored in applicaon
memory (via a pointer) or faster GPU memory (in a buer object).
void VertexAribPointer(uint index, int size, enum type,
boolean normalized, sizei stride, const void *pointer);
type: BYTE, UNSIGNED_BYTE, SHORT, UNSIGNED_SHORT, FIXED, FLOAT
index: [0, MAX_VERTEX_ATTRIBS - 1]
If an ARRAY_BUFFER is bound, the aribute will be read from the
bound buer, and pointer is treated as an oset within the buer.
void EnableVertexAribArray(uint index);
void DisableVertexAribArray(uint index);
index: [0, MAX_VERTEX_ATTRIBS - 1]
void DrawArrays(enum mode, int rst, sizei count);
void DrawElements(enum mode, sizei count, enum type,
void *indices);
mode: POINTS, LINE_STRIP, LINE_LOOP, LINES, TRIANGLE_STRIP,
TRIANGLE_FAN, TRIANGLES
type: UNSIGNED_BYTE, UNSIGNED_SHORT
If an ELEMENT_ARRAY_BUFFER is bound, the indices will be read
from the bound buer, and indices is treated as an oset within
the buer.
OpenGL ES Command Syntax [2.3]
Open GL ES commands are formed from a return type, a name, and oponally a type leer i for 32-bit int, or f for 32-bit oat,
as shown by the prototype below:
return-type Name{1234}{if}{v} ([args ,] T arg1 , . . . , T argN [, args]);
The arguments enclosed in brackets ([args ,] and [, args]) may or may not be present.
The argument type T and the number N of arguments may be indicated by the command name suxes. N is 1, 2, 3, or 4 if
present, or else corresponds to the type leers. If “v” is present, an array of N items is passed by a pointer.
For brevity, the OpenGL documentaon and this reference may omit the standard prexes.
The actual names are of the forms: glFunconName(), GL_CONSTANT, GLtype
Errors [2.5]
enum GetError( void );
//Returns one of the following:
INVALID_ENUM Enum argument out of range
INVALID_FRAMEBUFFER_OPERATION Framebuer is incomplete
INVALID_VALUE Numeric argument out of range
INVALID_OPERATION Operaon illegal in current state
OUT_OF_MEMORY Not enough memory le to execute command
NO_ERROR No error encountered
Buer Objects
[2.9]
Buer objects hold vertex array data or indices in
high-performance server memory.
void GenBuers(sizei n, uint *buers);
void DeleteBuers(sizei n, const uint *buers);
Creang and Binding Buer Objects
void BindBuer(enum target, uint buer);
target: ARRAY_BUFFER, ELEMENT_ARRAY_BUFFER
Creang Buer Object Data Stores
void BuerData(enum target, sizeiptr size,
const void *data, enum usage);
usage: STATIC_DRAW, STREAM_DRAW, DYNAMIC_DRAW
Updang Buer Object Data Stores
void BuerSubData(enum target, intptr oset,
sizeiptr size, const void *data);
target: ARRAY_BUFFER, ELEMENT_ARRAY_BUFFER
Buer Object Queries [6.1.6, 6.1.3]
boolean IsBuer(uint buer);
void GetBuerParameteriv(enum target, enum value,
T data);
target: ARRAY_BUFFER, ELEMENT_ ARRAY_BUFFER
value: BUFFER_SIZE, BUFFER_USAGE
Viewport and Clipping
Controlling the Viewport
[2.12.1]
void DepthRangef(clampf n, clampf f);
void Viewport(int x, int y, sizei w, sizei h);
Rasterizaon [3]
Points [3.3]
Point size is taken from the shader builn gl_PointSize and
clamped to the implementaon-dependent point size range.
Line Segments [3.4]
void LineWidth(oat width);
Polygons [3.5]
void FrontFace(enum dir);
dir: CCW, CW
void CullFace(enum mode);
mode: FRONT, BACK, FRONT_AND_BACK
Enable/Disable(CULL_FACE)
void PolygonOset(oat factor, oat units);
Enable/Disable(POLYGON_OFFSET_FILL)
Pixel Rectangles [3.6, 4.3]
void PixelStorei(enum pname, int param);
pname: UNPACK_ALIGNMENT, PACK_ALIGNMENT
Texturing [3.7]
Shaders support texturing using at least
MAX_VERTEX_TEXTURE_IMAGE_UNITS images for vertex
shaders and at least MAX_TEXTURE_IMAGE_UNITS images
for fragment shaders.
void AcveTexture(enum texture);
texture: [TEXTURE0..TEXTUREi] where i =
MAX_COMBINED_TEXTURE_IMAGE_UNITS-1
Texture Image Specicaon [3.7.1]
void TexImage2D(enum target, int level, int internalformat,
sizei width, sizei height, int border, enum format,
enum type, void *data);
target: TEXTURE_2D, TEXTURE_CUBE_MAP_POSITIVE_{X,Y,Z}
TEXTURE_CUBE_MAP_NEGATIVE_{X,Y,Z}
internalformat: ALPHA, LUMINANCE, LUMINANCE_ALPHA, RGB,
RGBA
format: ALPHA, RGB, RGBA, LUMINANCE, LUMINANCE_ALPHA
type: UNSIGNED_BYTE, UNSIGNED_SHORT_5_6_5,
UNSIGNED_SHORT_4_4_4_4, UNSIGNED_SHORT_5_5_5_1
Conversion from RGBA pixel components to internal
texture components:
Base Internal Format
RGBA
Internal Components
ALPHA
A
A
LUMINANCE
R L
LUMINANCE _ALPHA R, A L, A
RGB R, G, B R, G, B
RGBA
R, G, B, A R, G, B, A
Alt. Texture Image Specicaon Commands [3.7.2]
Texture images may also be specied using image data taken
directly from the framebuer, and rectangular subregions of
exisng texture images may be respecied.
void CopyTexImage2D(enum target, int level,
enum internalformat, int x, int y, sizei width,
sizei height, int border);
target: TEXTURE_2D, TEXTURE_CUBE_MAP_POSITIVE_{X, Y, Z},
TEXTURE_CUBE_MAP_NEGATIVE_{X, Y, Z}
internalformat: See TexImage2D
void TexSubImage2D(enum target, int level, int xoset,
int yoset, sizei width, sizei height, enum format,
enum type, void *data);
target: TEXTURE_CUBE_MAP_POSITIVE_{X, Y, Z},
TEXTURE_CUBE_MAP_NEGATIVE_{X, Y, Z}
format and type: See TexImage2D
void CopyTexSubImage2D(enum target, int level, int xoset,
int yoset, int x, int y, sizei width, sizei height);
target: TEXTURE_2D, TEXTURE_CUBE_MAP_POSITIVE_{X, Y, Z},
TEXTURE_CUBE_MAP_NEGATIVE_{X, Y, Z}
format and type: See TexImage2D
Compressed Texture Images [3.7.3]
void CompressedTexImage2D(enum target, int level,
enum internalformat, sizei width, sizei height,
int border, sizei imageSize, void *data);
target and internalformat: See TexImage2D
void CompressedTexSubImage2D(enum target, int level,
int xoset, int yoset, sizei width, sizei height,
enum format, sizei imageSize, void *data);
target and internalformat: See TexImage2D
Texture Parameters [3.7.4]
void TexParameter{if}(enum target, enum pname,
T param);
void TexParameter{if}v(enum target, enum pname,
T params);
target: TEXTURE_2D, TEXTURE_CUBE_MAP
pname: TEXTURE_WRAP_{S, T}, TEXTURE_{MIN, MAG}_FILTER
Manual Mipmap Generaon [3.7.11]
void GenerateMipmap(enum target);
target: TEXTURE_2D, TEXTURE_CUBE_MAP
Texture Objects [3.7.13]
void BindTexture(enum target, uint texture);
void DeleteTextures(sizei n, uint *textures);
void GenTextures(sizei n, uint *textures);
Enumerated Queries [6.1.3]
void GetTexParameter{if}v(enum target, enum value,
T data);
target: TEXTURE_2D, TEXTURE_CUBE_MAP
value: TEXTURE_WRAP_{S, T}, TEXTURE_{MIN, MAG}_FILTER
Texture Queries [6.1.4]
boolean IsTexture(uint texture);
GL Data Types [2.3]
GL types are not C types.
GL Type
Minimum
Bit Width Descripon
boolean 1 Boolean
byte 8 Signed binary integer
ubyte 8 Unsigned binary integer
char 8 Characters making up strings
short 16 Signed 2’s complement binary integer
ushort 16 Unsigned binary integer
int 32 Signed 2’s complement binary integer
uint 32 Unsigned binary integer
xed 32 Signed 2’s complement 16.16 scaled integer
sizei 32 Non-negave binary integer size
enum 32 Enumerated binary integer value
intptr ptrbits Signed 2’s complement binary integer
sizeiptr ptrbits Non-negave binary integer size
biield 32 Bit eld
oat 32 Floang-point value
clampf 32 Floang-point value clamped to [0; 1]
Reading Pixels
[4.3.1]
void ReadPixels(int x, int y, sizei width, sizei height,
enum format, enum type, void *data);
format: RGBA type: UNSIGNED_BYTE
Note: ReadPixels() also accepts a queriable implementaon-
dened format/type combinaon, see [4.3.1]
.