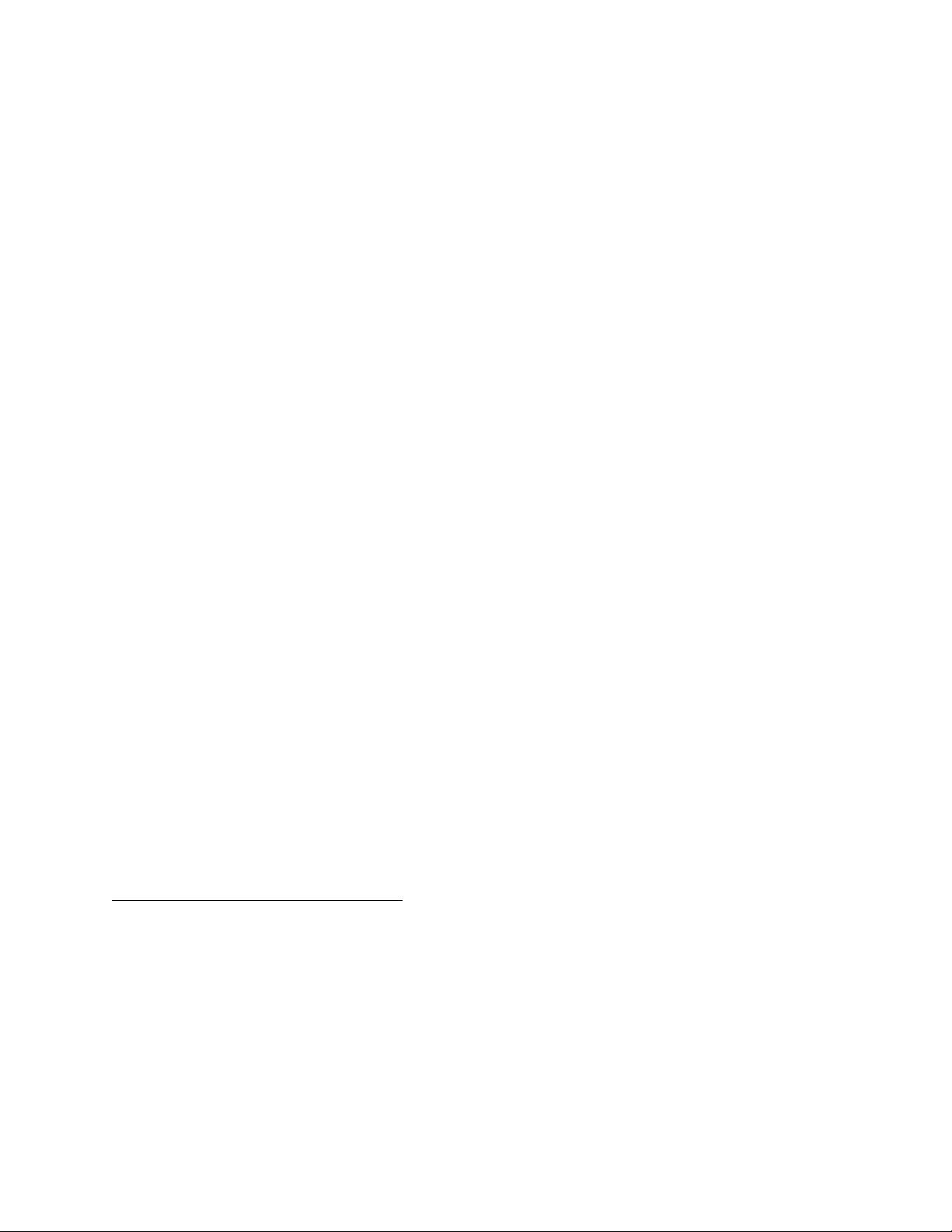
8 CHAPTER 1. INTRODUCTION
ferent Python implementations available. The one that we will use, i.e., the one available from
python.org, is sometimes called CPython and was written in the C programming language.
Other implementations that exist include IronPython (which works with the Microsoft .NET frame-
work), Jython (which was written in Java), and PyPy (which is written in Python). The details of
how these different implementations translate statements from the Python language into some-
thing the computer understands is not our concern. However, it is worthwhile to try to distinguish
between compilers and interpreters.
Some computer languages, such as FORTRAN, C, and C++, typically require that you write
a program, then you compile it (i.e., have a separate program known as a compiler translate your
program into executable code the computer understands), and finally you run it. The CPython
implementation of Python is different in that we can write statements and have the Python in-
terpreter act on them immediately. In this way we can instantly see what individual statements
do. The instant feedback provided by interpreters, such as CPython, is useful in learning to pro-
gram. An interpreter is a program that is somewhat like a compiler in that it takes statements
that we’ve written in a computer language and translates them into something the computer under-
stands. However, with an interpreter, the translation is followed immediately by execution. Each
statement is executed “on the fly.”
12
1.6 Running Python
With Python we can use interactive sessions in which we enter statements one at a time and the
interpreter acts on them. Alternatively, we can write all our commands, i.e., our program, in a file
that is stored on the computer and then have the interpreter act on that stored program. In this
case some compilation may be done behind the scenes, but Python will still not typically provide
speeds comparable to a true compiled language.
13
We will discuss putting programs in files in Sec.
1.6.2. First, we want to consider the two most common forms of interactive sessions for the Python
interpreter.
Returning to the statements in Listing 1.3, if they are entered in an interactive session, it is
difficult to observe the behavior that was described for that listing because the print() state-
ments have to be entered one at a time and output will be produced immediately after each entry.
In Python we can have multiple statements on a single line if the statements are separated by a
semicolon. Thus, if you want to verify that the code in Listing 1.3 is correct, you should enter it as
shown in Listing 1.5.
12
Compiled languages, such as C++ and Java, typically have an advantage in speed over interpreted languages such
as Python. When speed is truly critical in an application, it is unlikely one would want to use Python. However, in
most applications Python is “fast enough.” Furthermore, the time required to develop a program in Python is typically
much less than in other languages. This shorter development time can often more than compensate for the slower
run-time. For example, if it takes one day to write a program in Python but a week to write it in Java, is it worth the
extra development time if the program takes one second to run in Java but two seconds to run in Python? Sometimes
the answer to this is definitely yes, but this is more the exception rather than the rule. Although it is beyond the scope
of this book, one can create programs that use Python together with code written in C. This approach can be used to
provide execution speeds that exceed the capabilities of programs written purely in Python.
13
When the CPython interpreter runs commands from a file for the first time, it compiles a “bytecode” version of
the code which is then run by the interpreter. The bytecode is stored in a file with a .pyc extension. When the file
code is rerun, the Python interpreter actually uses the bytecode rather than re-interpreting the original code as long as
the Python statements have not been changed. This speeds up execution of the code.