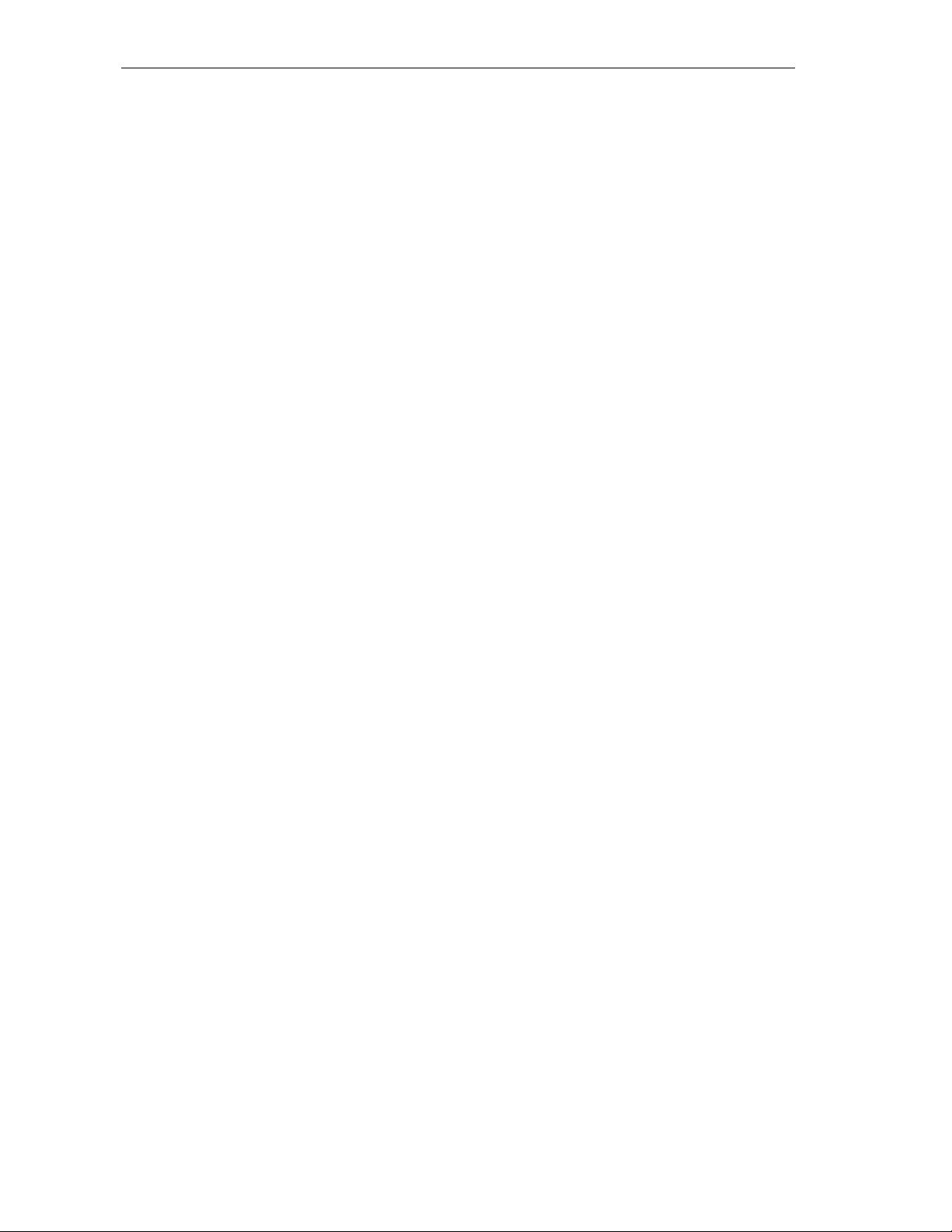
Chapter 1
20
Computers can't yet do any of these things very well – it's still much easier to explain to someone how
to tie their shoelaces than it is to set the clock on the video machine.
The most important thing you need to keep in mind, though, is that you're never going to be able to
express a task to a computer if you can't express it to yourself. Computer programming leaves little
room for vague specifications and hand waving. If you want to write a program to, say, remove useless
files from your computer, you need to be able to explain how to determine whether a file is useless or
not. You need to examine and break down your own mental processes when carrying out the task for
yourself: Should you delete a file that hasn't been accessed for a long time? How long, precisely? Do
you delete it immediately, or do you examine it? If you examine it, how much of it? And what are you
examining it for?
The first step in programming is to stop thinking in terms of 'I want a program that removes useless
files,' but instead thinking 'I want a program that looks at each file on the computer in turn and deletes
the file if it is over six months old and if the first five lines do not contain any of the words 'Simon',
'Perl' or 'important'. In other words, you have to specify your task precisely.
When you're able to restructure your question, you need to translate that into the programming
language you're using. Unfortunately, the programming language may not have a direct equivalent for
what you're trying to say. So, you have to get your meaning across using what parts of the language are
available to you, and this may well mean breaking down your task yet further. For instance, there's no
way of saying 'if the first five lines do not contain any of the following words' in Perl. However, there is
a way of saying 'if a line contains this word', a way of saying 'get another line', and 'do this five times'.
Programming is the art of putting those elements together to get them to do what you want.
So much for what you have to do – what does the computer have to do? Once we have specified the
task in our programming language, the computer takes our instructions and performs them. We call this
running or executing the program. Usually, we'll specify the instructions in a file, which we edit with an
ordinary text editor; sometimes, if we have a small program, we can get away with typing the whole
thing in at the command line. Either way, the instructions that we give to the computer – in our case,
written in Perl – are collectively called the source code (or sometimes just code) to our program.
Interpreted vs. Compiled Source Code
What exactly does the computer do with our source code, then? Traditionally, there were two ways to
describe what computer languages did with their code: You could say they were compiled, or that they
were interpreted.
An interpreted language, such as Basic, needs another program called an interpreter to process the
source code every time you want to run the program. This translates the source code down to a lower
level for the computer's consumption as it goes along. We call the lower-level language machine code,
because it's for machines to read, whereas source code is for humans. While the latter can look relatively
like English, for example, ("do_this() if $that"), machine code looks a lot more like what you'd
expect computers to be happier with, for example, "4576616E67656C6961", and that's the easy-to-
read version! The exact machine code produced depends on the processor of the computer and the
operating system it runs, the translation would be very different for an x86 computer running Windows
NT compared to a Sun or Digital computer running Unix.
A compiled language, on the other hand, such as C, uses a compiler to do all this processing one time
only before the code is ever run. After that, you can run the machine code directly, without needing the