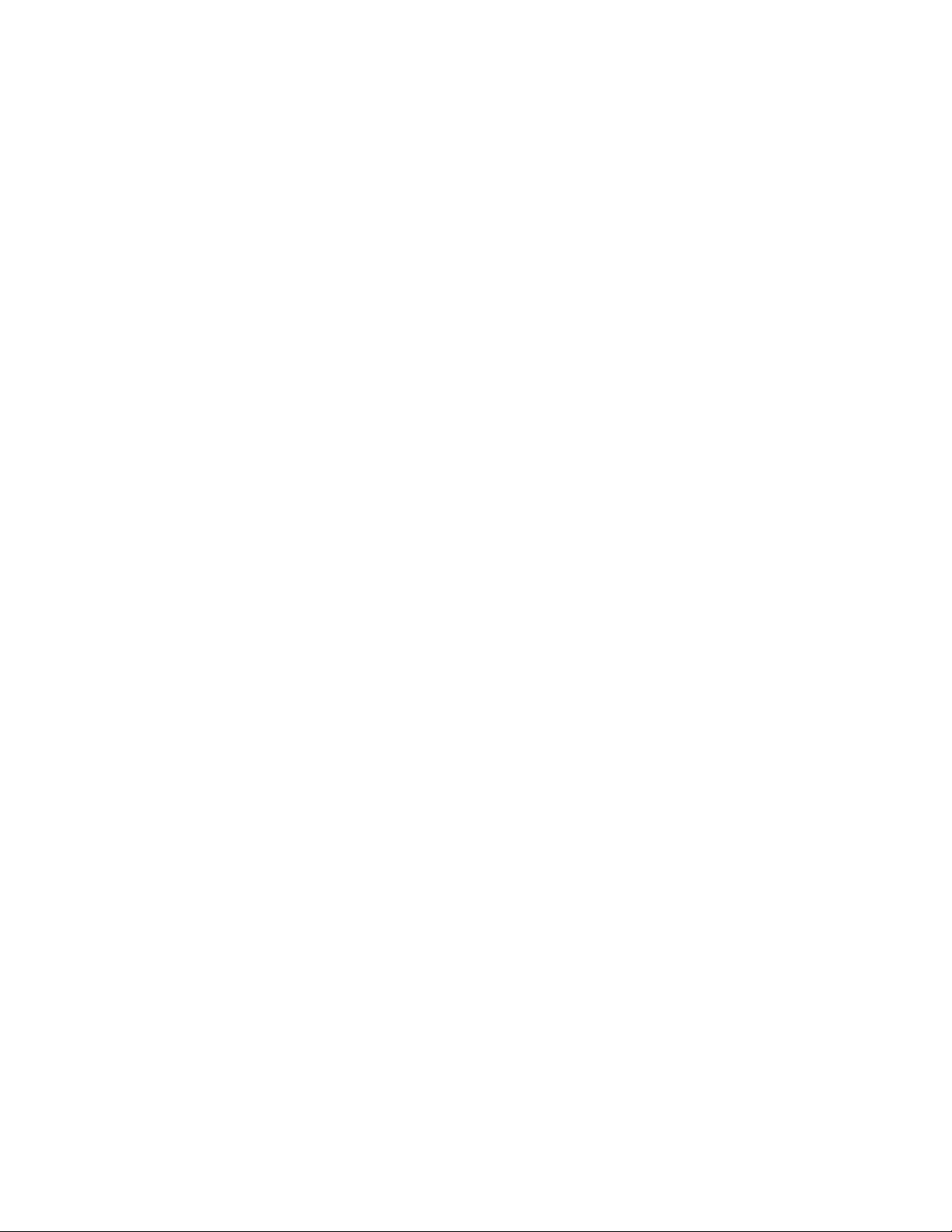
What Do All Those Funny Symbols Mean?
What you've just seen is the definition of a subroutine, which by itself won't do anything. You
use it by including the subroutine in your program and then providing it with the two
parameters it needs: \@array and $word. \@array is a reference to the array named
@array.
The first line, sub binary_search {, begins the definition of the subroutine named
"binary_search". That definition ends with the closing brace } at the very end of the code.break
Page 4
Next, my ($array, $word) = @_;, assigns the first two subroutine arguments to the
scalars $array and $word. You know they're scalars because they begin with dollar signs.
The my statement declares the scope of the variables—they're lexical variables, private to this
subroutine, and will vanish when the subroutine finishes. Use my whenever you can.
The following line, my ($low, $high) = ( 0, @$array - 1 ); declares and
initializes two more lexical scalars. $low is initialized to 0—actually unnecessary, but good
form. $high is initialized to @$array - 1, which dereferences the scalar variable
$array to get at the array underneath. In this context, the statement computes the length
(@$array) and subtracts 1 to get the index of the last element.
Hopefully, the first argument passed to binary_search() was a reference to an array.
Thanks to the first my line of the subroutine, that reference is now accessible as $array, and
the array pointed to by that value can be accessed as @$array.
Then the subroutine enters a while loop, which executes as long as $low <= $high; that
is, as long as our window is still open. Inside the loop, the word to be checked (more
precisely, the index of the word to be checked) is assigned to $try. If that word precedes our
target word,
*
we assign $try + 1 to $low, which shrinks the window to include only the
elements following $try, and we jump back to the beginning of the while loop via the
next. If our target word precedes the current word, we adjust $high instead. If neither word
precedes the other, we have a match, and we return $try. If our while loop exits, we know
that the word isn't present, and so undef is returned.
References
The most significant addition to the Perl language in Perl 5 is references, their use is described
in the perlref documentation bundled with Perl. A reference is a scalar value (thus, all
references begin with a $) whose value is the location (more or less) of another variable. That
variable might be another scalar, or an array, a hash, or even a snippet of Perl code. The
advantage of references is that they provide a level of indirection. Whenever you invoke a
subroutine, Perl needs to copy the subroutine arguments. If you pass an array of ten thousand
elements, those all have to be copied. But if you pass a reference to those elements as we've
done in binary_search(), only the reference needs to be copied. As a result, the
subroutine runs faster and scales up to larger inputs better.
More important, references are essential for constructing complex data structures, as you'll see
in Chapter 2, Basic Data Structures.break