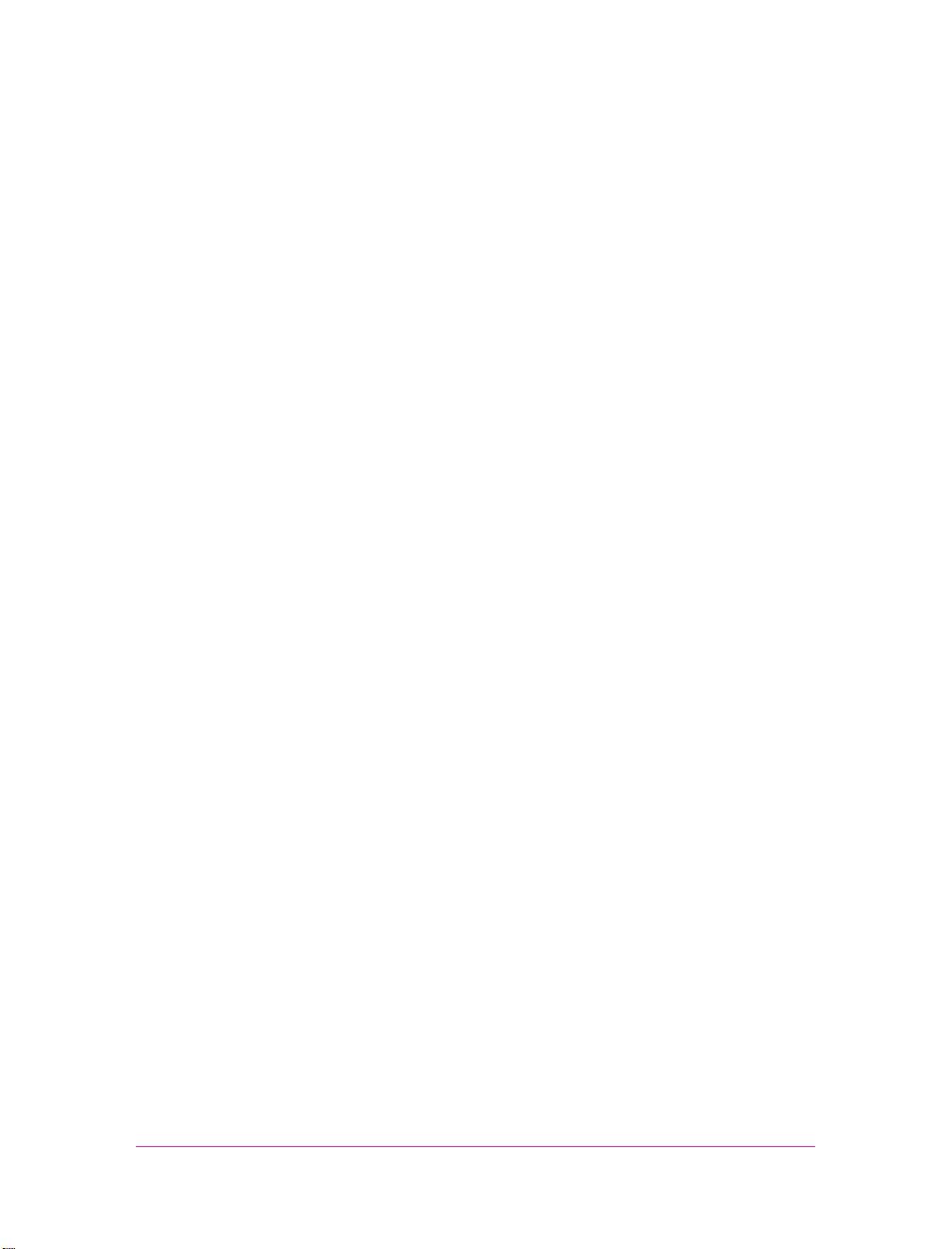
qualifiers?,” posted to Stack Overflow on 14 January 2014. My Item 15 treatment of
C++14’s expanded support for constexpr functions incorporates information I
received from Rein Halbersma. Item 16 is based on Herb Sutter’s C++ and Beyond
2012 presentation, “You don’t know const and mutable.” Item 18’s advice to have
factory functions return std::unique_ptrs is based on Herb Sutter’s 30 May 2013
article, “GotW# 90 Solution: Factories.” In Item 19, fastLoadWidget is derived from
Herb Sutter’s Going Native 2013 presentation, “My Favorite C++ 10-Liner.” My treat‐
ment of std::unique_ptr and incomplete types in Item 22 draws on Herb Sutter’s
27 November 2011 article, “GotW #100: Compilation Firewalls” as well as Howard
Hinnant’s 22 May 2011 answer to the Stack Overflow question, “Is
std::unique_ptr<T> required to know the full definition of T?” The Matrix addition
example in Item 25 is based on writings by David Abrahams. JoeArgonne’s 8 Decem‐
ber 2012 comment on the 30 November 2012 blog post, “Another alternative to
lambda move capture,” was the source of Item 32’s std::bind-based approach to
emulating init capture in C++11. Item 37’s explanation of the problem with an
implicit detach in std::thread’s destructor is taken from Hans-J. Boehm’s 4
December 2008 paper, “N2802: A plea to reconsider detach-on-destruction for thread
objects.” Item 41 was originally motivated by discussions of David Abrahams’ 15
August 2009 blog post, “Want speed? Pass by value.” The idea that move-only types
deserve special treatment is due to Matthew Fioravante, while the analysis of
assignment-based copying stems from comments by Howard Hinnant. In Item 42,
Stephan T. Lavavej and Howard Hinnant helped me understand the relative perfor‐
mance profiles of emplacement and insertion functions, and Michael Winterberg
brought to my attention how emplacement can lead to resource leaks. (Michael cred‐
its Sean Parent’s Going Native 2013 presentation, “C++ Seasoning,” as his source).
Michael also pointed out how emplacement functions use direct initialization, while
insertion functions use copy initialization.
Reviewing drafts of a technical book is a demanding, time-consuming, and utterly
critical task, and I’m fortunate that so many people were willing to do it for me. Full
or partial drafts of Effective Modern C++ were officially reviewed by Cassio Neri,
Nate Kohl, Gerhard Kreuzer, Leor Zolman, Bart Vandewoestyne, Stephan T. Lavavej,
Nevin “:-)” Liber, Rachel Cheng, Rob Stewart, Bob Steagall, Damien Watkins, Bradley
E. Needham, Rainer Grimm, Fredrik Winkler, Jonathan Wakely, Herb Sutter, Andrei
Alexandrescu, Eric Niebler, Thomas Becker, Roger Orr, Anthony Williams, Michael
Winterberg, Benjamin Huchley, Tom Kirby-Green, Alexey A Nikitin, William Deal‐
try, Hubert Matthews, and Tomasz Kamiński. I also received feedback from several
readers through O’Reilly’s Early Release EBooks and Safari Books Online’s Rough
Cuts, comments on my blog (The View from Aristeia), and email. I’m grateful to each
of these people. The book is much better than it would have been without their help.
I’m particularly indebted to Stephan T. Lavavej and Rob Stewart, whose extraordi‐
narily detailed and comprehensive remarks lead me to worry that they spent nearly as
xiv | Acknowledgments