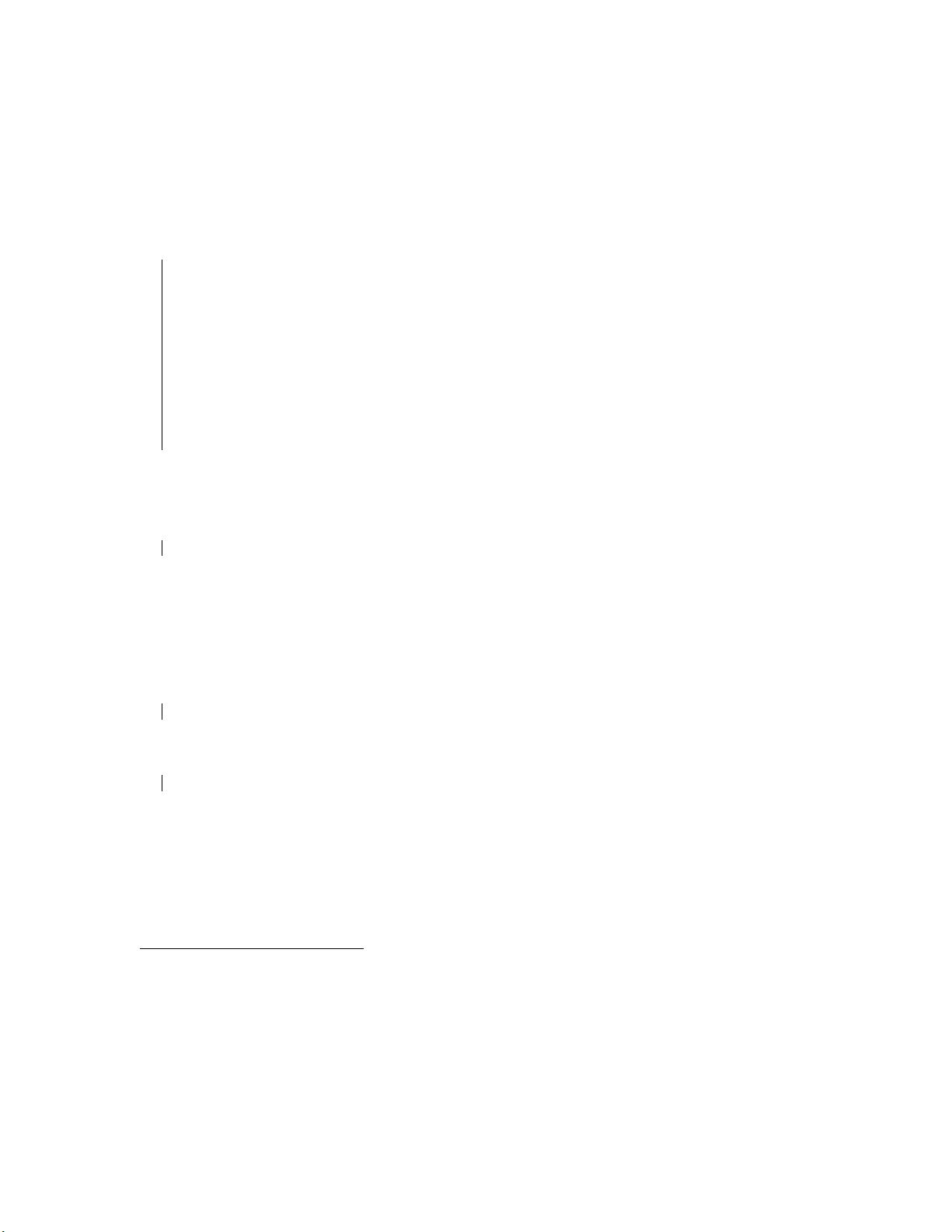
call. To get out of this bookkeeping chore, we can employ the using namespace cv; directive as
shown in Example 2-2
1
. This tells the compiler to assume that functions might belong to that namespace.
Note also the difference in include files between Example 2-1 and Example 2-2; in the former, we used the
general include opencv.hpp, whereas in the latter, we used only the necessary include file to improve
compile time.
Example 2-2: Same as Example 2-1 but employing the “using namespace” directive. For faster compile, we
use only the needed header file, not the generic opencv.hpp.
#include "opencv2/highgui/highgui.hpp"
using namespace cv;
int main( int argc, char** argv ) {
Mat img = imread( argv[1], -1 );
if( img.empty() ) return -1;
namedWindow( "Example2", WINDOW_AUTOSIZE );
imshow( "Example2", img );
waitKey( 0 );
destroyWindow( "Example2" );
}
When compiled and run from the command line with a single argument, Example 2-1 loads an image into
memory and displays it on the screen. It then waits until the user presses a key, at which time it closes the
window and exits. Let’s go through the program line by line and take a moment to understand what each
command is doing.
cv::Mat img = cv::imread( argv[1], -1 );
This line loads the image.
2
The function cv::imread() is a high-level routine that determines the file
format to be loaded based on the file name; it also automatically allocates the memory needed for the image
data structure. Note that cv::imread() can read a wide variety of image formats, including BMP, DIB,
JPEG, JPE, PNG, PBM, PGM, PPM, SR, RAS, and TIFF. A cv::Mat structure is returned. This structure
is the OpenCV construct with which you will deal the most. OpenCV uses this structure to handle all kinds
of images: single-channel, multichannel, integer-valued, floating-point-valued, and so on. The line
immediately following
if( img.empty() ) return -1;
checks to see if an image was in fact read. Another high-level function, cv::namedWindow(), opens a
window on the screen that can contain and display an image.
cv::namedWindow( "Example2", cv::WINDOW_AUTOSIZE );
This function, provided by the HighGUI library, also assigns a name to the window (in this case,
"Example2"). Future HighGUI calls that interact with this window will refer to it by this name.
The second argument to cv::namedWindow() defines window properties. It may be set either to 0 (the
default value) or to cv::WINDOW_AUTOSIZE. In the former case, the size of the window will be the
same regardless of the image size, and the image will be scaled to fit within the window. In the latter case,
1
Of course, once you do this, you risk conflicting names with other potential namespaces. If the function foo()
exists, say, in the cv and std namespaces, you must specify which function you are talking about using either
cv::foo() or std::foo() as you intend. In this book, other than in our specific example of Example 2-2, we
will use the explicit form cv:: for objects in the OpenCV namespace, as this is generally considered to be better
programming style.
2
A proper program would check for the existence of argv[1] and, in its absence, deliver an instructional error
message for the user. We will abbreviate such necessities in this book and assume that the reader is cultured enough to
understand the importance of error-handling code.