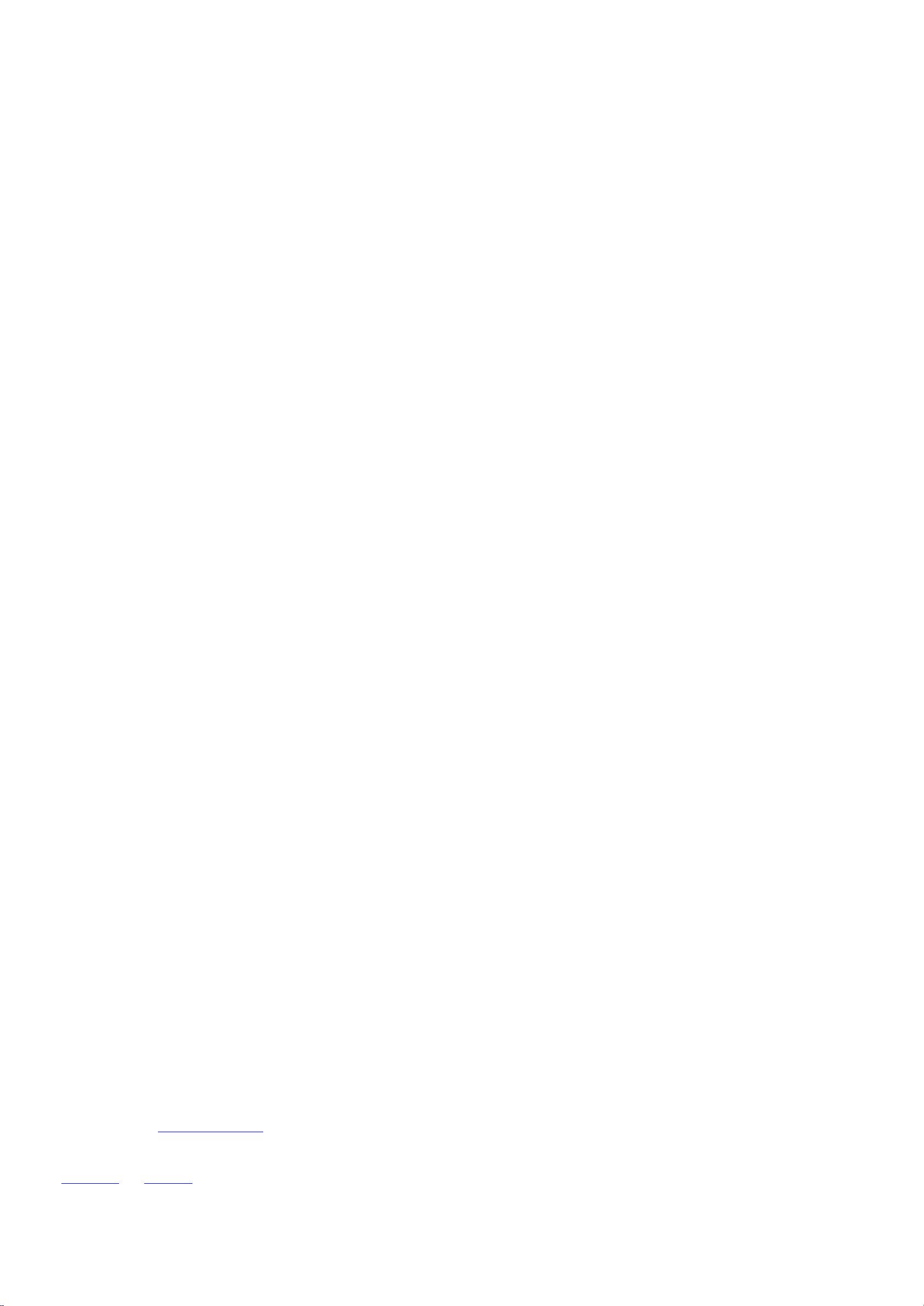
CefRefPtr<MyApp> app(new MyApp);
// Populate this structure to customize CEF behavior.
CefSettings settings;
// Specify the path for the sub-process executable.
CefString(&settings.browser_subprocess_path).FromASCII(“/path/to/subprocess”);
// Initialize CEF in the main process.
CefInitialize(main_args, settings, app.get());
// Run the CEF message loop. This will block until CefQuitMessageLoop() is called.
CefRunMessageLoop();
// Shut down CEF.
CefShutdown();
return 0;
}
Sub-process application entry-point function:
// Program entry-point function.
int main(int argc, char* argv[]) {
// Structure for passing command-line arguments.
// The definition of this structure is platform-specific.
CefMainArgs main_args(argc, argv);
// Optional implementation of the CefApp interface.
CefRefPtr<MyApp> app(new MyApp);
// Execute the sub-process logic. This will block until the sub-process should exit.
return CefExecuteProcess(main_args, app.get());
}
Message Loop Integration
CEF can also integrate with an existing application message loop instead of running its own message loop. There are two ways to do this.
1.
Call CefDoMessageLoopWork() on a regular basis instead of calling CefRunMessageLoop(). Each call to CefDoMessageLoopWork() will perform a single iteration of the
CEF message loop. Caution should be used with this approach. Calling the method too infrequently will starve the CEF message loop and negatively impact browser
performance. Calling the method too frequently will negatively impact CPU usage.
2.
Set CefSettings.multi_threaded_message_loop = true (Windows only). This will cause CEF to run the browser UI thread on a separate thread from the main application
thread. With this approach neither CefDoMessageLoopWork() nor CefRunMessageLoop() need to be called. CefInitialize() and CefShutdown() should still be called on
the main application thread. You will need to provide your own mechanism for communicating with the main application thread (see for example the message window
usage in cefclient_win.cpp). You can test this mode in cefclient on Windows by running with the “--multi-threaded-message-loop” command-line flag.
CefSettings
The CefSettings structure allows configuration of application-wide CEF settings. Some commonly configured members include:
browser_subprocess_path The path to a separate executable that will be launched for sub-processes. See the “Separate Sub-Process Executable” section for more
information.
multi_threaded_message_loop Set to true to have the browser process message loop run in a separate thread. See the “Message Loop Integration” section for more
information.
command_line_args_disabled Set to true to disable configuration of browser process features using standard CEF and Chromium command-line arguments. See the
“Command Line Arguments” section for more information.
cache_path The location where cache data will be stored on disk. If empty an in-memory cache will be used for some features and a temporary disk cache will be
used for others. HTML5 databases such as localStorage will only persist across sessions if a cache path is specified.
locale The locale string that will be passed to Blink. If empty the default locale of "en-US" will be used. This value is ignored on Linux where locale is determined using
environment variable parsing with the precedence order: LANGUAGE, LC_ALL, LC_MESSAGES and LANG. Also configurable using the "lang" command-line switch.
log_file The directory and file name to use for the debug log. If empty, the default name of "debug.log" will be used and the file will be written to the application
directory. Also configurable using the "log-file" command-line switch.
log_severity The log severity. Only messages of this severity level or higher will be logged. Also configurable using the "log-severity" command-line switch with a
value of "verbose", "info", "warning", "error", "error-report" or "disable".
resources_dir_path The fully qualified path for the resources directory. If this value is empty the cef.pak and/or devtools_resources.pak files must be located in the
module directory on Windows/Linux or the app bundle Resources directory on Mac OS X. Also configurable using the "resources-dir-path" command-line switch.
locales_dir_path The fully qualified path for the locales directory. If this value is empty the locales directory must be located in the module directory. This value is
ignored on Mac OS X where pack files are always loaded from the app bundle Resources directory. Also configurable using the "locales-dir-path" command-line
switch.
remote_debugging_port Set to a value between 1024 and 65535 to enable remote debugging on the specified port. For example, if 8080 is specified the remote
debugging URL will be http://localhost:8080. CEF can be remotely debugged from any CEF or Chrome browser window. Also configurable using the "remote-
debugging-port" command-line switch.
CefBrowser and CefFrame
The CefBrowser and CefFrame objects are used for sending commands to the browser and for retrieving state information in callback methods. Each CefBrowser
object will have a single main CefFrame object representing the top-level frame and zero or more CefFrame objects representing sub-frames. For example, a browser
that loads two iframes will have three CefFrame objects (the top-level frame and the two iframes).
To load a URL in the browser main frame:
browser->GetMainFrame()->LoadURL(some_url);