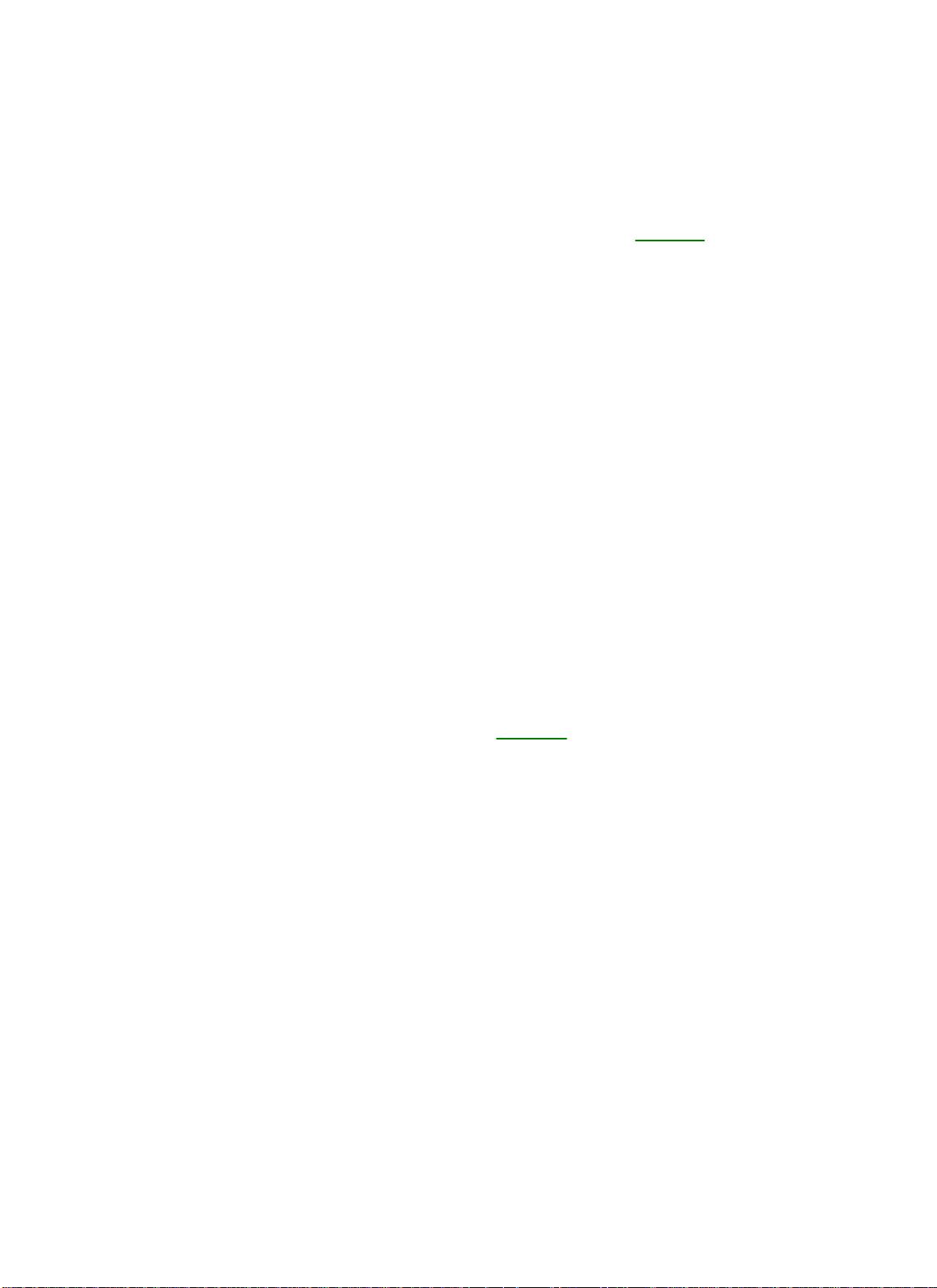
or 20 years ago. The column marked "PROGRAM INSTRUCTIONS" is the main path around the edge
of the board, of which only a portion can be shown here. This is the assembly language computer
program, the actual series of steps and tests that, when executed, causes the computer to do
something useful. Setting up this series of program instructions is what programming in assembly
language actually is.
Everything else is odds and ends in the middle of the board that serve the game in progress. You're
probably noticing (perhaps with sagging spirits) that there are a lot of numbers involved. (They're weird
numbers, too-what, for example, does "004B" mean? I deal with that issue in Chapter 2, Alien Bases.)
I'm sorry, but that's simply the way the game is played. Assembly language, at the innermost level, is
nothing but numbers, and if you hate numbers the way most people hate anchovies, you're going to
have a rough time of it. (I like anchovies, which is part of my legend. Learn to like numbers. They're not
as salty.)
I should caution you that the Game of Assembly Language represents no real computer processor like
the Pentium. Also, I've made the names of instructions more clearly understandable than the names of
the instructions in Intel assembly language. In the real world, instruction names are typically things like
STOSB, DAA, INC, SBB, and other crypticisms that cannot be understood without considerable
explanation. We're easing into this stuff sidewise, and in this chapter I have to sugarcoat certain things
a little to draw the metaphors clearly.
Code and Data
Like most board games (including the Game of Big Bux), the assembly language board game consists
of two broad categories of elements: game steps and places to store things. The "game steps" are the
steps and tests I've been speaking of all along. The places to store things are just that: cubbyholes into
which you can place numbers, with the confidence that those numbers will remain where you put them
until you take them out or change them somehow.
In programming terms, the game steps are called code, and the numbers in their cubbyholes (as
distinct from the cubbyholes themselves) are called data. The cubbyholes themselves are usually
called storage. (The difference between the places you store information and the information you store
in them is crucial. Don't confuse them.)
The Game of Big Bux works the same way. Look back to Figure 1.1 and note that in the Start a
Business detour, there is an instruction reading Add $850,000 to checking account. The checking
account is one of several different kinds of storage in the Game of Big Bux, and money values are a
type of data. It's no different conceptually from an instruction in the Game of Assembly Language
reading ADD 5 to Register A. An ADD instruction in the code alters a data value stored in a cubbyhole
named Register A.
Code and data are two very different kinds of critters, but they interact in ways that make the game
interesting. The code includes steps that place data into storage (MOVE instructions) and steps that
alter data that is already in storage (INCREMENT and DECREMENT instructions). Most of the time
you'll think of code as being the master of data, in that the code writes data values into storage. Data
does influence code as well, however. Among the tests that the code makes are tests that examine
data in storage, the COMPARE instructions. If a given data value exists in storage, the code may do
one thing; if that value does not exist in storage, the code will do something else, as in the JUMP
BACK and JUMP AHEAD instructions.
The short block of instructions marked PROCEDURE is a detour off the main stream of instructions. At
any point in the program you can duck out into the procedure, perform its steps and tests, and then
return to the very place from which you left. This allows a sequence of steps and tests that is generally
useful and used frequently to exist in only one place rather than exist as a separate copy everywhere it
is needed.
Addresses
Another critical concept lies in the funny numbers at the left side of the program step locations and data
locations. Each number is unique, in that a location tagged with that number appears only once inside
the computer. This location is called an address. Data is stored and retrieved by specifying the data's