"Swift无限循环控件开发" 在iOS应用开发中,经常需要实现一种能够无限循环展示内容的控件,比如广告轮播或者内容滚动通知。本篇内容将详细介绍如何使用Swift语言开发一个自定义的无限循环控件。这个控件通常用于展示一系列固定的项目,如图片、文字或其他UI元素,并在用户滑动时形成无缝衔接的循环效果。 首先,理解无限循环的实现原理至关重要。在iOS中,最常用的实现无限循环的基础控件是UICollectionView,因为它支持横向和纵向的滚动,并且具有Cell复用机制,可以高效地处理大量数据。无限循环的核心在于数据的处理和滚动逻辑,通常我们会将数据量扩大3倍,这样可以确保在用户滑动时,无论是向左还是向右,都能自然过渡到下一个循环。 具体实现步骤如下: 1. 数据准备:创建一个包含原始数据3倍数量的数组,中间部分的数据用于实际展示,两端的数据作为过渡。 2. UICollectionView配置:设置UICollectionView的布局,使其能够水平滚动,并确保每个Cell的大小与数据项匹配。 3. 滚动逻辑:当用户向左滑动并且到达中间数据的最后一项时,自动滚动到中间数据的第一项;反之,当用户向右滑动并且位于第一项时,滚动到中间数据的最后一项。这样,用户会感觉一直在同一组数据中循环。 以下是一个简单的代码实现框架: ```swift import UIKit class InfiniteLoopContentView: UIView { // 声明协议和代理方法 protocol InfiniteLoopContentViewDelegate: AnyObject { func infiniteLoopView(_ loopView: InfiniteLoopContentView, index: Int) -> UICollectionViewCell func numberOfRows(in loopView: InfiniteLoopContentView) -> Int } // 初始化视图并设置代理 weak var delegate: InfiniteLoopContentViewDelegate? // UICollectionView相关属性 private let collectionView: UICollectionView = UICollectionView(frame: .zero, collectionViewLayout: UICollectionViewFlowLayout()) // 初始化方法 override init(frame: CGRect) { super.init(frame: frame) setupCollectionView() } required init?(coder aDecoder: NSCoder) { fatalError("init(coder:) has not been implemented") } // 设置CollectionView相关配置 private func setupCollectionView() { // 添加UICollectionView到视图 addSubview(collectionView) // 配置布局 collectionView.dataSource = self collectionView.delegate = self collectionView.scrollDirection = .horizontal collectionView.translatesAutoresizingMaskIntoConstraints = false NSLayoutConstraint.activate([ collectionView.leadingAnchor.constraint(equalTo: leadingAnchor), collectionView.trailingAnchor.constraint(equalTo: trailingAnchor), collectionView.topAnchor.constraint(equalTo: topAnchor), collectionView.bottomAnchor.constraint(equalTo: bottomAnchor) ]) } // UICollectionViewDataSource方法 func numberOfSections(in collectionView: UICollectionView) -> Int { return 1 } func collectionView(_ collectionView: UICollectionView, numberOfItemsInSection section: Int) -> Int { return delegate?.numberOfRows(in: self) ?? 0 } func collectionView(_ collectionView: UICollectionView, cellForItemAt indexPath: IndexPath) -> UICollectionViewCell { let cell = delegate?.infiniteLoopView(self, index: indexPath.item) return cell! } // UICollectionViewDelegate方法 // 在这里添加滚动逻辑,实现无限循环 } ``` 以上代码仅展示了无限循环视图的基本框架,实际应用中还需要根据滚动事件处理滚动逻辑,确保在边界条件时平滑过渡。同时,你可能需要自定义UICollectionViewCell以适应不同的数据展示需求。 测试环境为Xcode 11.5和Mac OS 10.15.4,创建名为"InfiniteLoopDemo"的项目,并在项目中实现上述代码,可以得到一个基本的无限循环控件。为了进一步完善控件的功能,还可以添加自动滚动、点击回调等特性,以满足不同应用场景的需求。
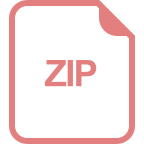
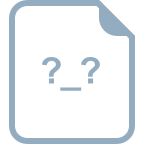
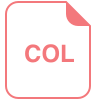
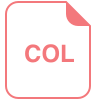
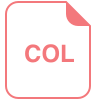
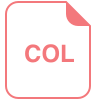













- 粉丝: 5
- 资源: 901
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

最新资源
- OptiX传输试题与SDH基础知识
- C++Builder函数详解与应用
- Linux shell (bash) 文件与字符串比较运算符详解
- Adam Gawne-Cain解读英文版WKT格式与常见投影标准
- dos命令详解:基础操作与网络测试必备
- Windows 蓝屏代码解析与处理指南
- PSoC CY8C24533在电动自行车控制器设计中的应用
- PHP整合FCKeditor网页编辑器教程
- Java Swing计算器源码示例:初学者入门教程
- Eclipse平台上的可视化开发:使用VEP与SWT
- 软件工程CASE工具实践指南
- AIX LVM详解:网络存储架构与管理
- 递归算法解析:文件系统、XML与树图
- 使用Struts2与MySQL构建Web登录验证教程
- PHP5 CLI模式:用PHP编写Shell脚本教程
- MyBatis与Spring完美整合:1.0.0-RC3详解

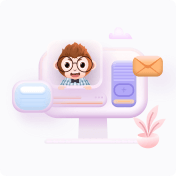
