没有合适的资源?快使用搜索试试~ 我知道了~
首页C#语言规范详解:从入门到精通
C#语言规范详解:从入门到精通
需积分: 4 1 下载量 18 浏览量
更新于2024-07-29
收藏 2.91MB PDF 举报
"C#语言规范.pdf"
C#语言规范是微软发布的一份详细文档,旨在定义和指导C#编程语言的使用,适用于初学者和经验丰富的开发者。这份3.0版的规范涵盖了C#的核心概念和语法,帮助开发者理解和遵循语言的规则。
1. **Introduction**:
- **Hello World**:这是学习任何编程语言时的第一个示例,通常用于展示最简单的程序结构。
- **Program structure**:这部分介绍C#程序的基本结构,包括命名空间、类、方法等。
- **Types and variables**:C#中的数据类型(如整型、浮点型、字符串)以及变量的声明和使用。
- **Expressions**:涉及算术、比较、逻辑等表达式,以及运算符的优先级和结合性。
- **Statements**:包括控制流语句,如条件语句(if-else)、循环(for, while, do-while)、跳转语句(break, continue)等。
2. **Classes and objects**:
- **Members**:类可以包含字段、方法、属性等成员。
- **Accessibility**:讨论了public、private、protected等访问修饰符,定义了成员的可见性。
- **Type parameters**:C#支持泛型,允许在类、接口和方法中使用类型参数,提供类型安全的代码复用。
- **Base classes**:介绍了类的继承机制,以及基类和派生类的概念。
- **Fields**:类中的数据成员,存储对象的状态。
- **Methods**:执行特定任务的函数,包括参数、局部变量、静态与实例方法、虚方法、重写、抽象方法和方法重载。
- **Constructors**:类的实例化过程,用于初始化对象。
- **Properties**:提供对字段的访问控制,类似于访问器。
- **Indexers**:允许类模仿数组或其他集合的索引访问方式。
- **Events**:支持事件驱动编程,用于对象间的通信。
- **Operators**:定义自定义操作符,如加法、乘法等。
- **Destructors**:类似于析构函数,用于清理对象资源。
3. **Structs**:结构体是值类型,与类不同,它们在内存中直接存储值,而非引用。
4. **Arrays**:C#中的多维数组、 Jagged arrays 和 Array initializers的使用。
5. **Interfaces**:接口定义了一组成员,类或结构体可以实现这些接口以提供特定功能。
6. **Enums**:枚举类型,用于定义一组命名的常量。
7. **Delegates**:委托是类型安全的函数指针,用于事件处理和回调。
8. **Attributes**:特性允许在元数据中附加信息,用于影响编译器的行为或提供运行时信息。
这份规范深入浅出地解释了C#的各个方面,是学习和精通C#编程的宝贵资料。通过阅读和理解这些内容,开发者能够编写出高效、可维护且符合规范的C#代码。
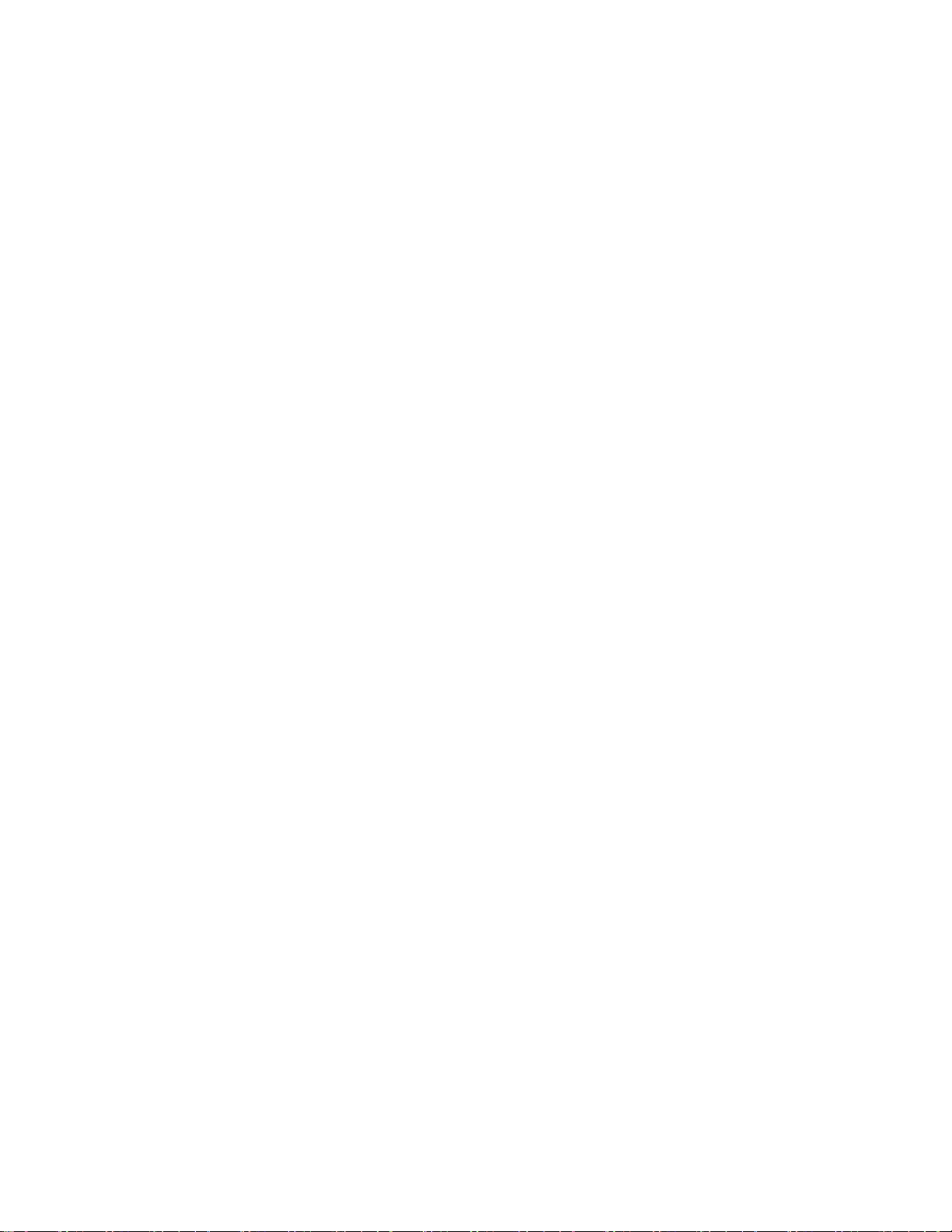
目录
版权所有 Microsoft Corporation 1999-2007。保留所有权利。
xvi
10.10.1 Unary operators ................................................................................................................................... 335
10.10.2 Binary operators ................................................................................................................................... 335
10.10.3 Conversion operators ........................................................................................................................... 336
10.11 Instance constructors .............................................................................................................................. 338
10.11.1 Constructor initializers ........................................................................................................................ 339
10.11.2 Instance variable initializers ............................................................................................................... 340
10.11.3 Constructor execution .......................................................................................................................... 340
10.11.4 Default constructors ............................................................................................................................. 342
10.11.5 Private constructors ............................................................................................................................. 342
10.11.6 Optional instance constructor parameters......................................................................................... 343
10.12 Static constructors ................................................................................................................................... 343
10.13 Destructors ............................................................................................................................................... 345
10.14 Iterators .................................................................................................................................................... 347
10.14.1 Enumerator interfaces ......................................................................................................................... 347
10.14.2 Enumerable interfaces ......................................................................................................................... 347
10.14.3 Yield type .............................................................................................................................................. 347
10.14.4 Enumerator objects .............................................................................................................................. 347
10.14.4.1 The MoveNext method ...................................................................................................................... 348
10.14.4.2 The Current property ....................................................................................................................... 349
10.14.4.3 The Dispose method .......................................................................................................................... 349
10.14.5 Enumerable objects .............................................................................................................................. 349
10.14.5.1 The GetEnumerator method ............................................................................................................ 350
10.14.6 Implementation example ..................................................................................................................... 350
11. Structs .......................................................................................................................................................... 357
11.1 Struct declarations .................................................................................................................................... 357
11.1.1 Struct modifiers ...................................................................................................................................... 357
11.1.2 Partial modifier ......................................................................................................................................
358
11.1.3 Struct interfaces ..................................................................................................................................... 358
11.1.4 Struct body .............................................................................................................................................. 358
11.2 Struct members ......................................................................................................................................... 358
11.3 Class and struct differences ...................................................................................................................... 358
11.3.1 Value semantics ...................................................................................................................................... 359
11.3.2 Inheritance .............................................................................................................................................. 360
11.3.3 Assignment .............................................................................................................................................. 360
11.3.4 Default values ......................................................................................................................................... 360
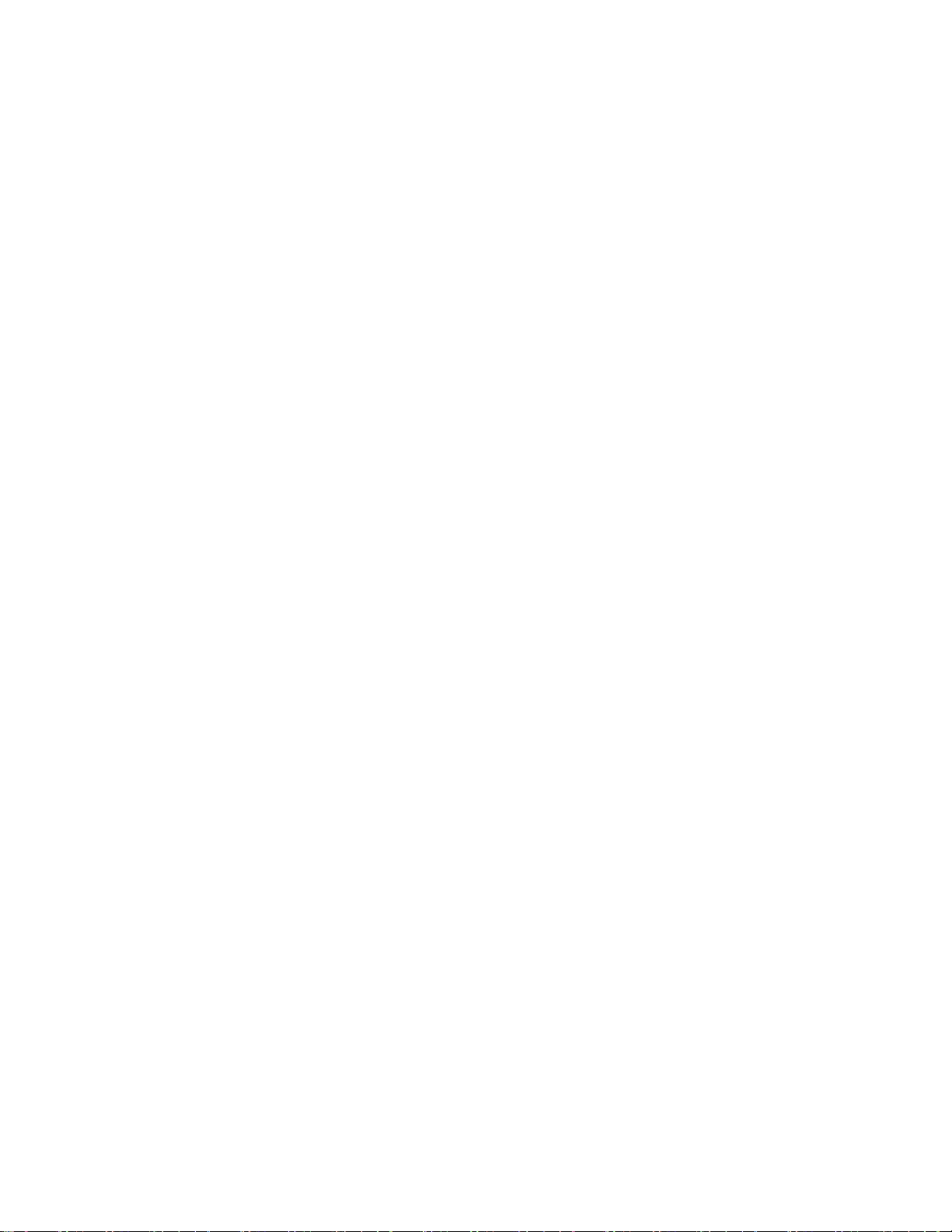
目录
版权所有 Microsoft Corporation 1999-2007。保留所有权利。
xvii
11.3.5 Boxing and unboxing ............................................................................................................................. 361
11.3.6 Meaning of this ....................................................................................................................................... 362
11.3.7 Field initializers ...................................................................................................................................... 363
11.3.8 Constructors ............................................................................................................................................ 363
11.3.9 Destructors .............................................................................................................................................. 364
11.3.10 Static constructors ................................................................................................................................ 364
11.4 Struct examples ......................................................................................................................................... 364
11.4.1 Database integer type ............................................................................................................................. 364
11.4.2 Database boolean type ........................................................................................................................... 366
12. Arrays ........................................................................................................................................................... 369
12.1 Array types ................................................................................................................................................ 369
12.1.1 The System.Array type .......................................................................................................................... 370
12.1.2 Arrays and the generic IList interface ................................................................................................. 370
12.2 Array creation ........................................................................................................................................... 370
12.3 Array element access ................................................................................................................................. 371
12.4 Array members .......................................................................................................................................... 371
12.5 Array covariance ....................................................................................................................................... 371
12.6 Array initializers ....................................................................................................................................... 371
13. Interfaces ...................................................................................................................................................... 375
13.1 Interface declarations ............................................................................................................................... 375
13.1.1 Interface modifiers ................................................................................................................................. 375
13.1.2 Partial modifier ...................................................................................................................................... 375
13.1.3 Base interfaces ........................................................................................................................................ 376
13.1.4 Interface body ......................................................................................................................................... 376
13.2 Interface members .................................................................................................................................... 376
13.2.1 Interface methods ...................................................................................................................................
378
13.2.2 Interface properties ................................................................................................................................ 378
13.2.3 Interface events ....................................................................................................................................... 378
13.2.4 Interface indexers ................................................................................................................................... 378
13.2.5 Interface member access ........................................................................................................................ 379
13.3 Fully qualified interface member names ................................................................................................. 380
13.4 Interface implementations ........................................................................................................................ 381
13.4.1 Explicit interface member implementations ........................................................................................ 382
13.4.2 Uniqueness of implemented interfaces ................................................................................................. 384
13.4.3 Implementation of generic methods ..................................................................................................... 385
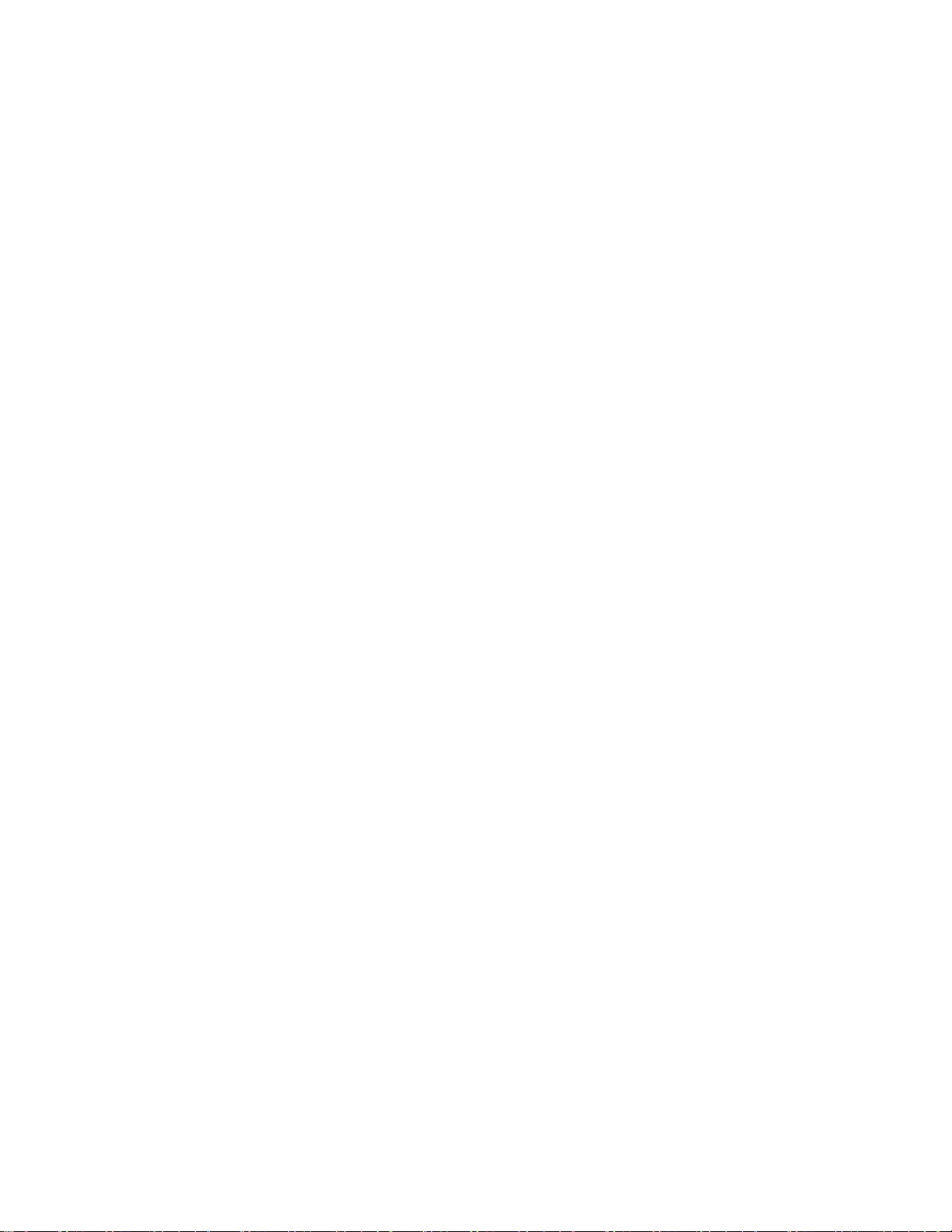
目录
版权所有 Microsoft Corporation 1999-2007。保留所有权利。
xviii
13.4.4 Interface mapping .................................................................................................................................. 385
13.4.5 Interface implementation inheritance .................................................................................................. 388
13.4.6 Interface re-implementation .................................................................................................................. 389
13.4.7 Abstract classes and interfaces.............................................................................................................. 391
14. Enums ........................................................................................................................................................... 393
14.1 Enum declarations .................................................................................................................................... 393
14.2 Enum modifiers ......................................................................................................................................... 393
14.3 Enum members .......................................................................................................................................... 394
14.4 The System.Enum type ............................................................................................................................. 396
14.5 Enum values and operations .................................................................................................................... 396
15. Delegates....................................................................................................................................................... 397
15.1 Delegate declarations ................................................................................................................................ 397
15.2 Delegate compatibility .............................................................................................................................. 399
15.3 Delegate instantiation ................................................................................................................................ 399
15.4 Delegate invocation ................................................................................................................................... 400
16. Exceptions .................................................................................................................................................... 403
16.1 Causes of exceptions .................................................................................................................................. 403
16.2 The System.Exception class ...................................................................................................................... 403
16.3 How exceptions are handled ..................................................................................................................... 403
16.4 Common Exception Classes...................................................................................................................... 404
17. Attributes ..................................................................................................................................................... 407
17.1 Attribute classes ........................................................................................................................................ 407
17.1.1 Attribute usage ....................................................................................................................................... 407
17.1.2 Positional and named parameters ........................................................................................................ 408
17.1.3 Attribute parameter types ..................................................................................................................... 409
17.2 Attribute specification .............................................................................................................................. 409
17.3 Attribute instances .................................................................................................................................... 414
17.3.1 Compilation of an attribute ................................................................................................................... 415
17.3.2 Run-time retrieval of an attribute instance ......................................................................................... 415
17.4 Reserved attributes ................................................................................................................................... 415
17.4.1 The AttributeUsage attribute ................................................................................................................ 415
17.4.2 The Conditional attribute ...................................................................................................................... 416
17.4.2.1 Conditional methods ........................................................................................................................... 416
17.4.2.2 Conditional attribute classes .............................................................................................................. 418
17.4.3 The Obsolete attribute ........................................................................................................................... 419
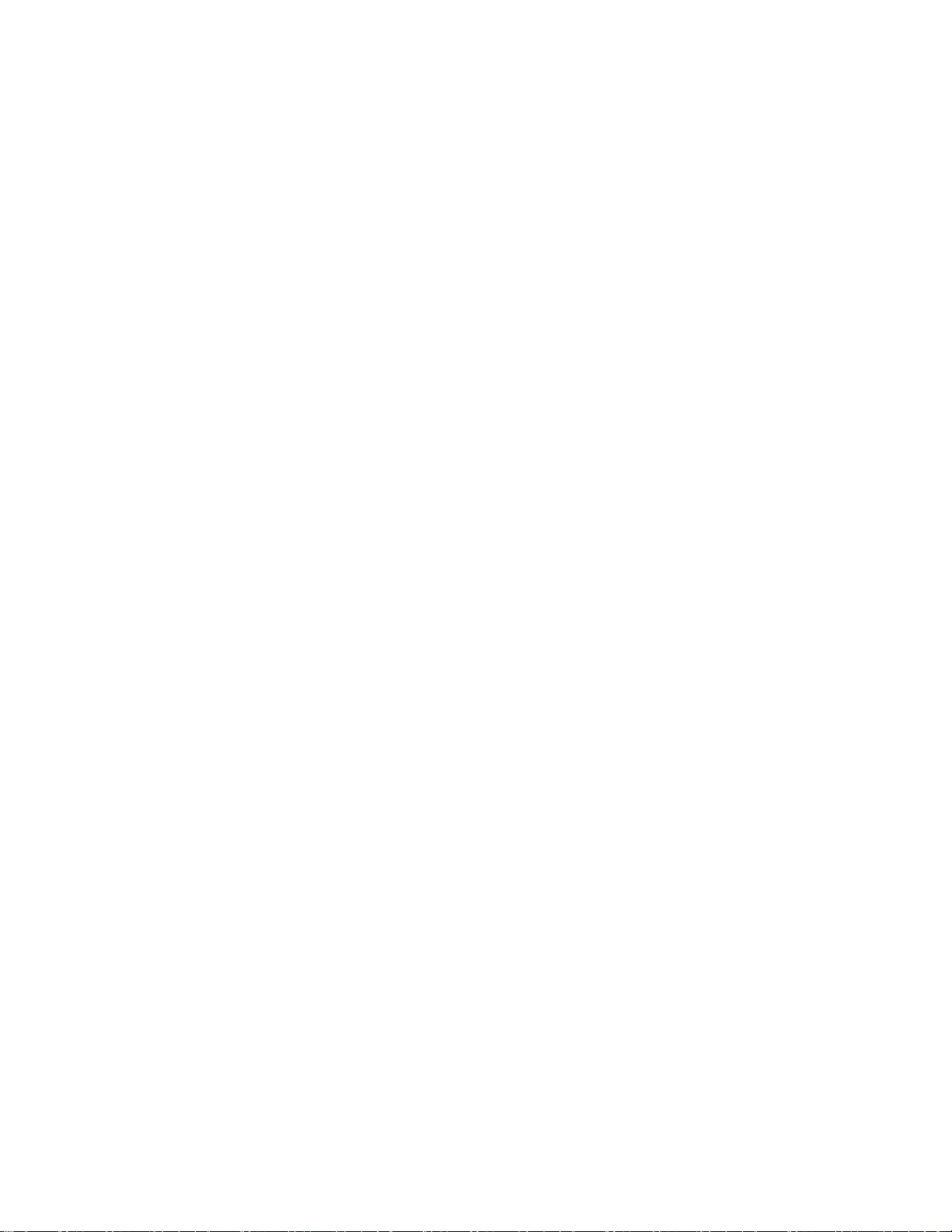
目录
版权所有 Microsoft Corporation 1999-2007。保留所有权利。
xix
17.5 Attributes for Interoperation ................................................................................................................... 420
17.5.1 Interoperation with COM and Win32 components ............................................................................ 421
17.5.2 Interoperation with other .NET languages .......................................................................................... 421
17.5.2.1 The IndexerName attribute ................................................................................................................ 421
18. Unsafe code .................................................................................................................................................. 423
18.1 Unsafe contexts .......................................................................................................................................... 423
18.2 Pointer types .............................................................................................................................................. 425
18.3 Fixed and moveable variables .................................................................................................................. 428
18.4 Pointer conversions ................................................................................................................................... 428
18.5 Pointers in expressions .............................................................................................................................. 429
18.5.1 Pointer indirection ................................................................................................................................. 430
18.5.2 Pointer member access........................................................................................................................... 430
18.5.3 Pointer element access ........................................................................................................................... 431
18.5.4 The address-of operator ......................................................................................................................... 432
18.5.5 Pointer increment and decrement ......................................................................................................... 433
18.5.6 Pointer arithmetic .................................................................................................................................. 433
18.5.7 Pointer comparison ................................................................................................................................ 434
18.5.8 The sizeof operator ................................................................................................................................. 434
18.6 The fixed statement ................................................................................................................................... 435
18.7 Fixed size buffers ....................................................................................................................................... 438
18.7.1 Fixed size buffer declarations ................................................................................................................ 438
18.7.2 Fixed size buffers in expressions ........................................................................................................... 440
18.7.3 Definite assignment checking ................................................................................................................ 440
18.8 Stack allocation .......................................................................................................................................... 441
18.9 Dynamic memory allocation ..................................................................................................................... 442
A. Documentation comments ........................................................................................................................... 445
A.1 Introduction ................................................................................................................................................ 445
A.2 Recommended tags ..................................................................................................................................... 446
A.2.1 <c> ............................................................................................................................................................ 447
A.2.2 <code>....................................................................................................................................................... 447
A.2.3 <example> ................................................................................................................................................ 448
A.2.4 <exception> .............................................................................................................................................. 448
A.2.5 <include> .................................................................................................................................................. 449
A.2.6 <list> ......................................................................................................................................................... 449
A.2.7 <para> ...................................................................................................................................................... 450
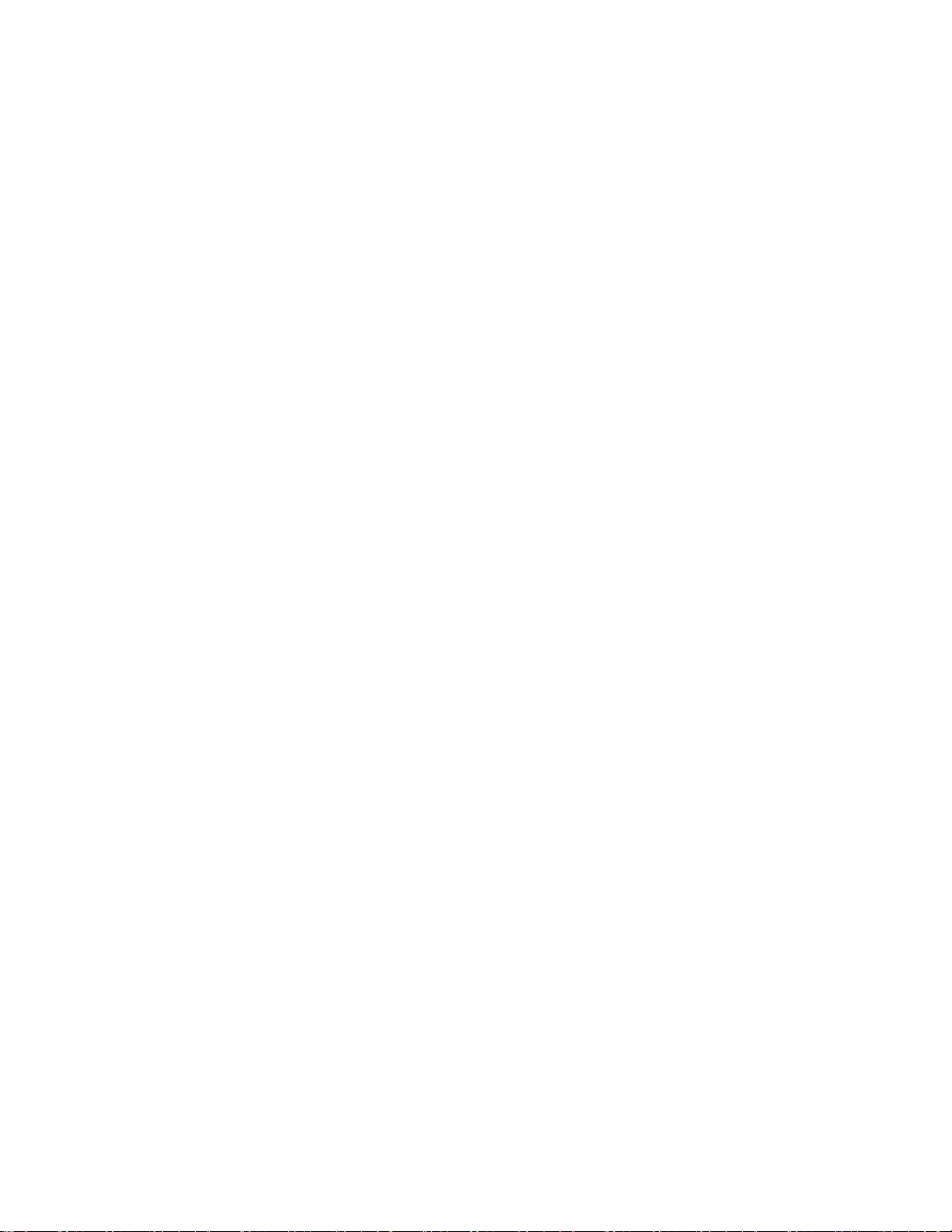
目录
版权所有 Microsoft Corporation 1999-2007。保留所有权利。
xx
A.2.8 <param> ................................................................................................................................................... 451
A.2.9 <paramref> .............................................................................................................................................. 451
A.2.10 <permission> .......................................................................................................................................... 451
A.2.11 <summary> ............................................................................................................................................ 452
A.2.12 <returns> ................................................................................................................................................ 452
A.2.13 <see> ....................................................................................................................................................... 453
A.2.14 <seealso>................................................................................................................................................. 453
A.2.15 <summary> ............................................................................................................................................ 454
A.2.16 <value> ................................................................................................................................................... 454
A.2.17 <typeparam> .......................................................................................................................................... 454
A.2.18 <typeparamref> ..................................................................................................................................... 455
A.3 Processing the documentation file ............................................................................................................. 455
A.3.1 ID string format ....................................................................................................................................... 455
A.3.2 ID string examples ................................................................................................................................... 456
A.4 An example.................................................................................................................................................. 460
A.4.1 C# source code ......................................................................................................................................... 460
A.4.2 Resulting XML ........................................................................................................................................ 462
B. Grammar ....................................................................................................................................................... 468
B.1 Lexical grammar ........................................................................................................................................ 468
B.1.1 Line terminators ...................................................................................................................................... 468
B.1.2 Comments ................................................................................................................................................. 468
B.1.3 White space .............................................................................................................................................. 469
B.1.4 Tokens ....................................................................................................................................................... 469
B.1.5 Unicode character escape sequences ...................................................................................................... 469
B.1.6 Identifiers ................................................................................................................................................. 470
B.1.7 Keywords ..................................................................................................................................................
471
B.1.8 Literals ...................................................................................................................................................... 471
B.1.9 Operators and punctuators..................................................................................................................... 473
B.1.10 Pre-processing directives ...................................................................................................................... 473
B.2 Syntactic grammar ..................................................................................................................................... 476
B.2.1 Basic concepts .......................................................................................................................................... 476
B.2.2 Types ......................................................................................................................................................... 476
B.2.3 Variables ................................................................................................................................................... 477
B.2.4 Expressions ............................................................................................................................................... 478
B.2.5 Statements ................................................................................................................................................ 484
剩余479页未读,继续阅读
点击了解资源详情
点击了解资源详情
点击了解资源详情
115 浏览量
2007-11-19 上传
327 浏览量
127 浏览量
102 浏览量
168 浏览量

简单世界
- 粉丝: 64
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

最新资源
- Android简易音乐播放器实现教程
- C++环境下fftwindow对FFT波形的测试分析
- ISOWorkshop6.0:多功能ISO镜像工具绿色版
- ActiveMQ与Spring结合的Maven项目实践教程
- Kotlin背景示例技术解析与应用
- json2canvas: 绘制复杂图形到Canvas上的新工具
- 驴友社区Android版:分享旅行新鲜事的交友平台
- 掌握Android GLSurfaceView打造炫酷3D UI界面
- 银灿IS903芯片量产软件V2.11.00.39功能详解
- 快速搭建Spring Web工程与MySQL数据库连接
- 纯CSS实现的带三角自定位提示框
- STM32F103平台LoRa模块开发指南
- Julia语言运行在WebAssembly上的实践指南
- 精选NPM包推荐:提高项目开发效率的必备工具
- 可视化设计横断面自动生成软件介绍
- 个性桌面电子时钟的设计与源代码解析
安全验证
文档复制为VIP权益,开通VIP直接复制
