没有合适的资源?快使用搜索试试~ 我知道了~
首页《Essential Angular for ASP.NET Core MVC》:Adam Freeman 指南
"Apress.Essential.Angular.for.ASP.NET.Core.MVC.1484229150.pdf" 是一本由Adam Freeman编著的专业书籍,旨在帮助读者深入理解并掌握Angular框架在ASP.NET Core MVC(Asynchronous Programming Model for Microsoft Common Language Infrastructure)环境中的应用。这本书是Apress出版社发行,出版日期为2017年,具有国际标准书号(ISBN-13):纸质版为978-1-4842-2915-6,电子版为978-1-4842-2916-3,还拥有DOI(Digital Object Identifier)10.1007/978-1-4842-2916-3。
Adam Freeman以其丰富的经验和深厚的技术背景,为读者提供了一本实用且详尽的指南,涵盖了Angular的核心概念、设计模式以及如何在ASP.NET Core MVC架构中有效地集成和优化Angular应用程序。书中不仅阐述了Angular 2及后续版本的基本原理,还会深入探讨Angular CLI (Command Line Interface) 的使用、模块化开发、组件开发、依赖注入、指令、服务、路由管理、表单验证等关键知识点。
对于ASP.NET Core MVC开发者来说,这本教程具有很高的价值,因为它强调了Angular如何提升Web应用程序的用户体验,以及如何与.NET Core的后端技术无缝对接。作者可能会通过实例演示和最佳实践来帮助读者理解Angular在处理复杂前端交互、数据绑定和异步操作方面的优势。
版权方面,该书受Adam Freeman所有,任何复制、重印、改编或传播行为都必须得到版权所有者的许可,以尊重作者的知识产权。书中可能会包含一些商标名、标志和图像,这些内容仅用于编辑目的,以提高读者对品牌的认知,并非意图侵犯商标权。
"Essential Angular for ASP.NET Core MVC"是一本针对.NET Core开发者打造的Angular框架入门与进阶教程,适合希望通过Angular增强Web开发能力的读者深入学习和实践。
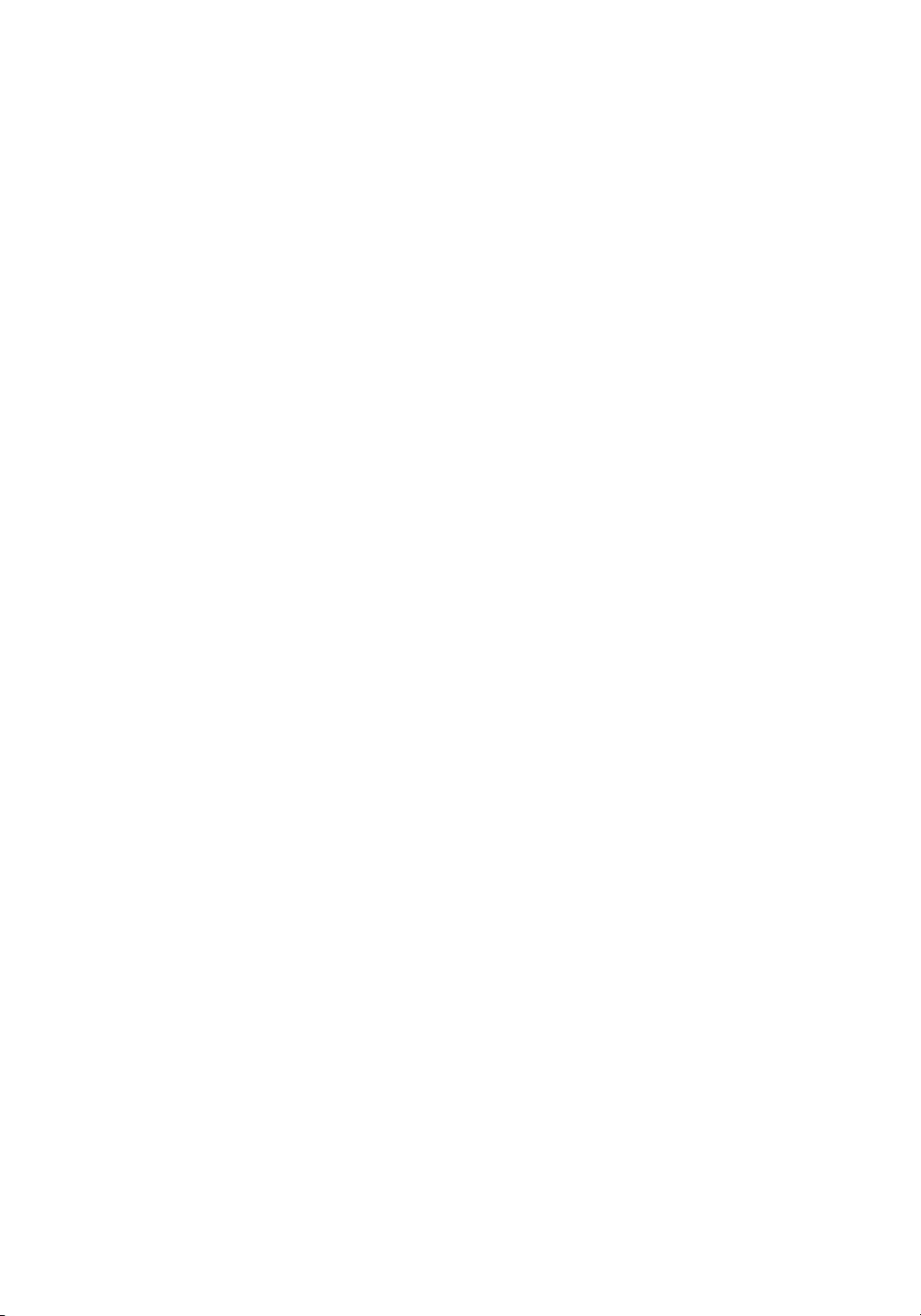
Chapter 1 ■ Understanding angUlar and asp.net Core MVC
2
I have written other books that provide the deep-dive for each framework. If you are unfamiliar with
ASP.NET Core MVC development, then you should read Pro ASP.NET Core MVC before this book. Once
you have mastered the basics of Angular development, then Pro Angular provides a comprehensive tour of
Angular features.
What Do You Need to Know?
Before reading this book, you should have a working knowledge of ASP.NET Core MVC development and
have a good understanding of JavaScript, HTML, and CSS.
Are There Lots of Examples?
There are loads of examples. The best way to learn is by example, and I have packed as many of them as
I can into this book. To maximize the number of examples in this book, I have adopted a simple convention
to avoid listing the same code over and over again.
To help you navigate the project, the caption for each listing includes the name of the file and the folder
in which it can be found, like in Listing 1-1.
Listing 1-1. The Contents of the CheckoutState.cs File in the Models/BindingTargets Folder
namespace SportsStore.Models.BindingTargets {
public class CheckoutState {
public string name { get; set; }
public string address { get; set; }
public string cardNumber { get; set; }
public string cardExpiry { get; set; }
public string cardSecurityCode { get; set; }
}
}
This is a listing from Chapter 9, and the caption tells you that it refers to a file called CheckoutState.cs,
which can be found in the Models/BindingTargets folder. A project that combines Angular and ASP.NET
Core MVC can have a lot of files, and it is important to change the right one. (Don’t worry about the code in
this listing or the folder structure for the moment.)
When I make changes to a file, I show the altered statements in bold, like in Listing 1-2.
Listing 1-2. Adding Methods in the SessionValuesController.cs File in the Controllers Folder
using Microsoft.AspNetCore.Http;
using Microsoft.AspNetCore.Mvc;
using Newtonsoft.Json;
using SportsStore.Models;
using SportsStore.Models.BindingTargets;
namespace SportsStore.Controllers {
[Route("/api/session")]
public class SessionValuesController : Controller {
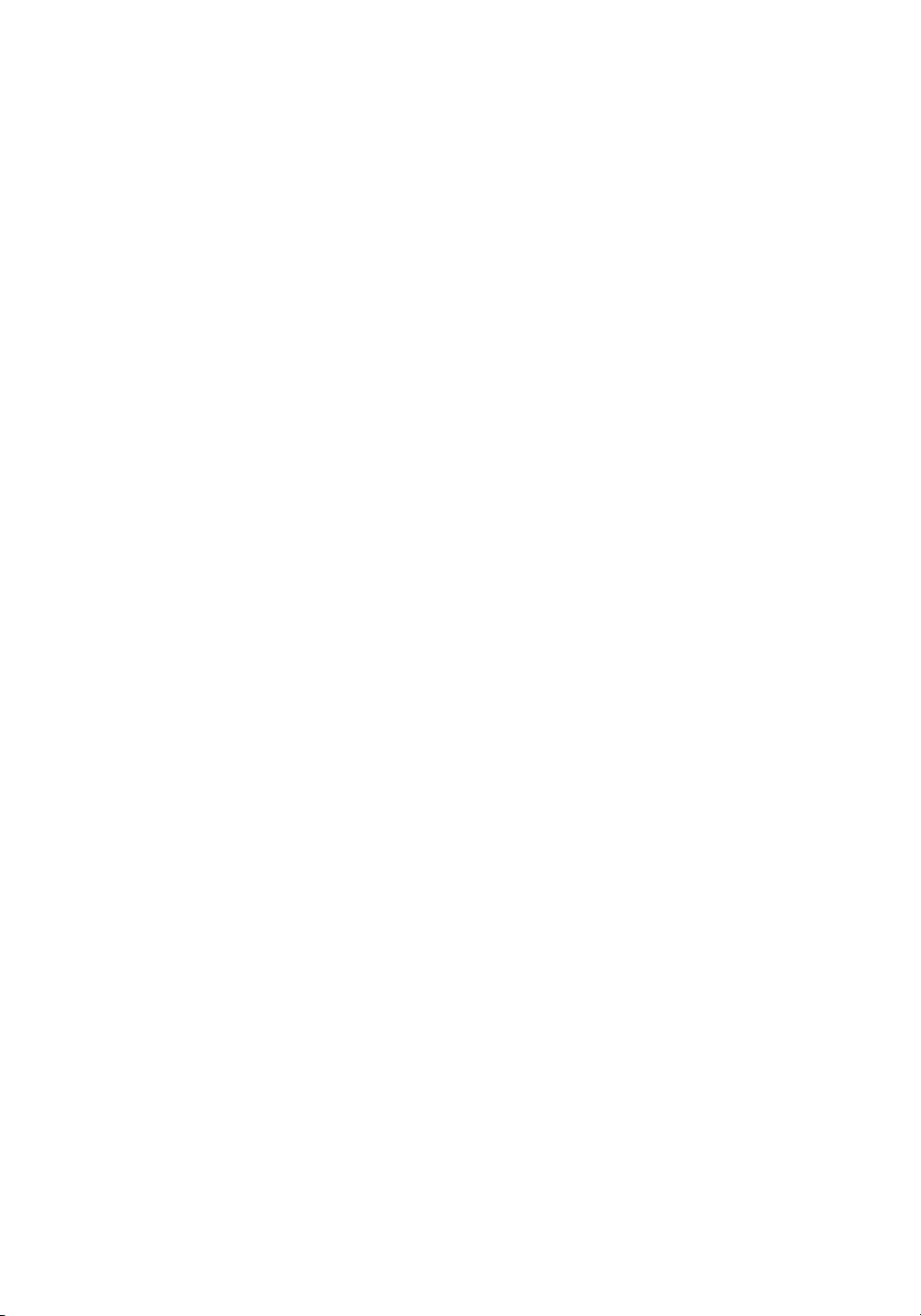
Chapter 1 ■ Understanding angUlar and asp.net Core MVC
3
[HttpGet("cart")]
public IActionResult GetCart() {
return Ok(HttpContext.Session.GetString("cart"));
}
[HttpPost("cart")]
public void StoreCart([FromBody] ProductSelection[] products) {
var jsonData = JsonConvert.SerializeObject(products);
HttpContext.Session.SetString("cart", jsonData);
}
[HttpGet("checkout")]
public IActionResult GetCheckout() {
return Ok(HttpContext.Session.GetString("checkout"));
}
[HttpPost("checkout")]
public void StoreCheckout([FromBody] CheckoutState data) {
HttpContext.Session.SetString("checkout",
JsonConvert.SerializeObject(data));
}
}
}
This is another listing from Chapter 9, and the bold statements indicate the changes that you should
make if you are following the example.
I use two different conventions to avoid repeating code in long files. For long class files, I omit methods
and properties, like in Listing 1-3.
Listing 1-3. Restricting Access in the SupplierValuesController.cs File in the Controllers Folder
using Microsoft.AspNetCore.Mvc;
using SportsStore.Models;
using SportsStore.Models.BindingTargets;
using System.Collections.Generic;
using Microsoft.AspNetCore.Authorization;
namespace SportsStore.Controllers {
[Route("api/suppliers")]
[Authorize(Roles = "Administrator")]
public class SupplierValuesController : Controller {
private DataContext context;
// ...methods omitted for brevity...
}
}
This listing from Chapter 11 shows you that a new attribute must be applied to the
SupplierValuesController class but doesn’t list the constructor or other methods, which remain
unchanged.
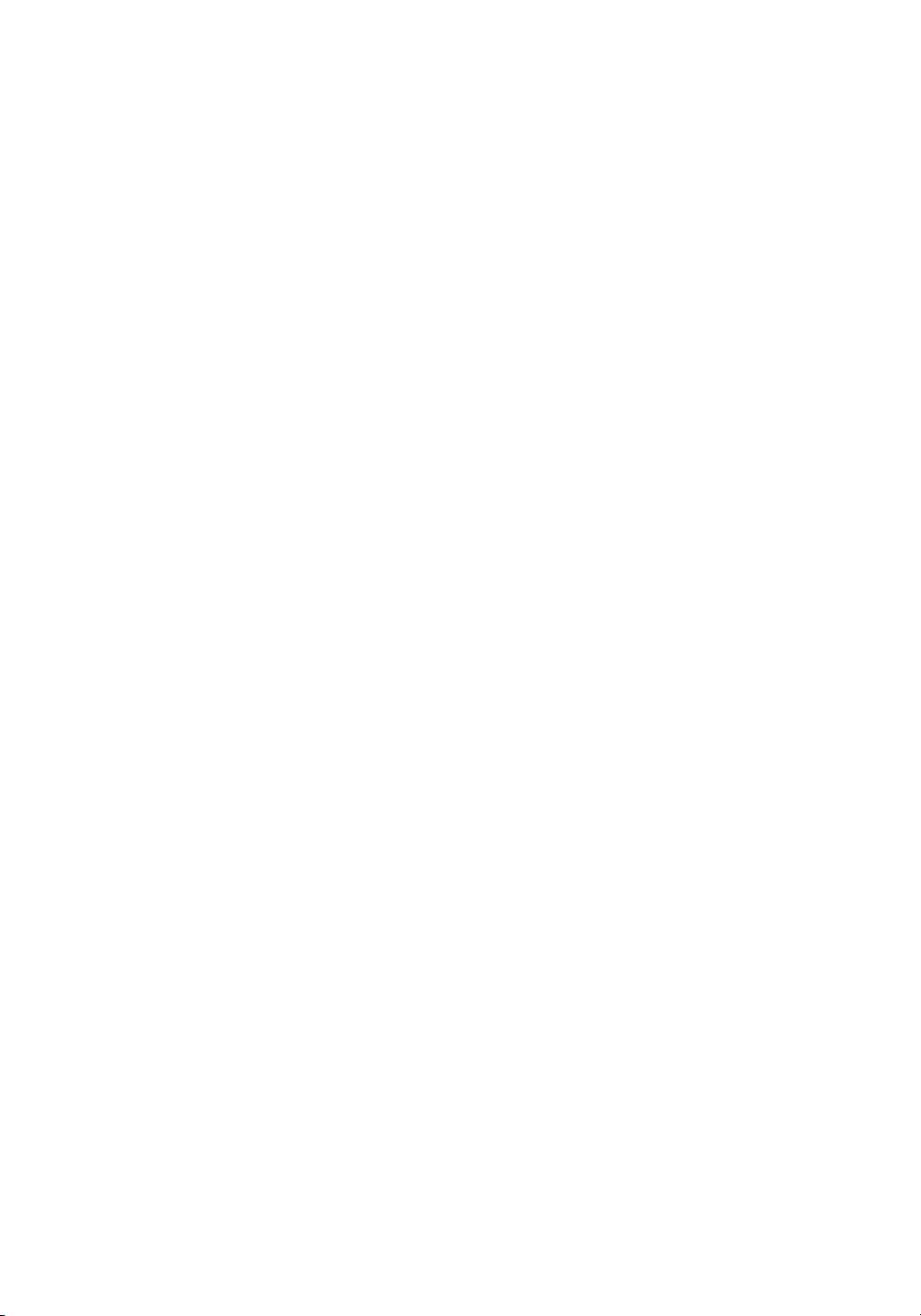
Chapter 1 ■ Understanding angUlar and asp.net Core MVC
4
This is the convention that I follow to highlight changes to a region within a file, such as when new
statements are required in a single method in a long file, like in Listing 1-4.
Listing 1-4. Configuring the JSON Serializer in the Startup.cs File in the SportsStore Folder
...
public void ConfigureServices(IServiceCollection services) {
services.AddDbContext<DataContext>(options =>
options.UseSqlServer(Configuration
["Data:Products:ConnectionString"]));
services.AddMvc().AddJsonOptions(opts => {
opts.SerializerSettings.ReferenceLoopHandling
= ReferenceLoopHandling.Serialize;
opts.SerializerSettings.NullValueHandling = NullValueHandling.Ignore;
});
}
...
This is a listing from Chapter 5 that requires a new statement in the ConfigureServices method of the
Startup class, which is defined in the Startup.cs file in the SportsStore folder, while the rest of the file
remains unchanged.
Where Can You Get the Example Code?
You can download the example projects for all the chapters in this book from https://github.com/apress/
esntl-angular-for-asp.net-core-mvc. The download is available without charge and includes all the
supporting resources that are required to re-create the examples without having to type them in. You don’t
have to download the code, but it is the easiest way of experimenting with the examples and makes it easy
to copy and paste code into your own projects.
Where Can You Get Corrections for This Book?
You can find corrections for this book in the Errata file in the GitHub repository for this book
(https://github.com/apress/esntl-angular-for-asp.net-core-mvc).
Contacting the Author
If you have problems making the examples in this chapter work or if you find a problem in the book, then
you can e-mail me at adam@adam-freeman.com, and I will try my best to help. Please check the errata for this
book at https://github.com/apress/esntl-angular-for-asp.net-core-mvc to see if it contains a solution
to your problem before contacting me.
Summary
In this chapter, I described the purpose and content of this book, explained how you can download the
project used for each chapter of the book, and described the conventions I use in the code listings.
In the next chapter, I show you how to set up your development environment in preparation for creating a
combined Angular and ASP.NET Core MVC project in Chapter 3.
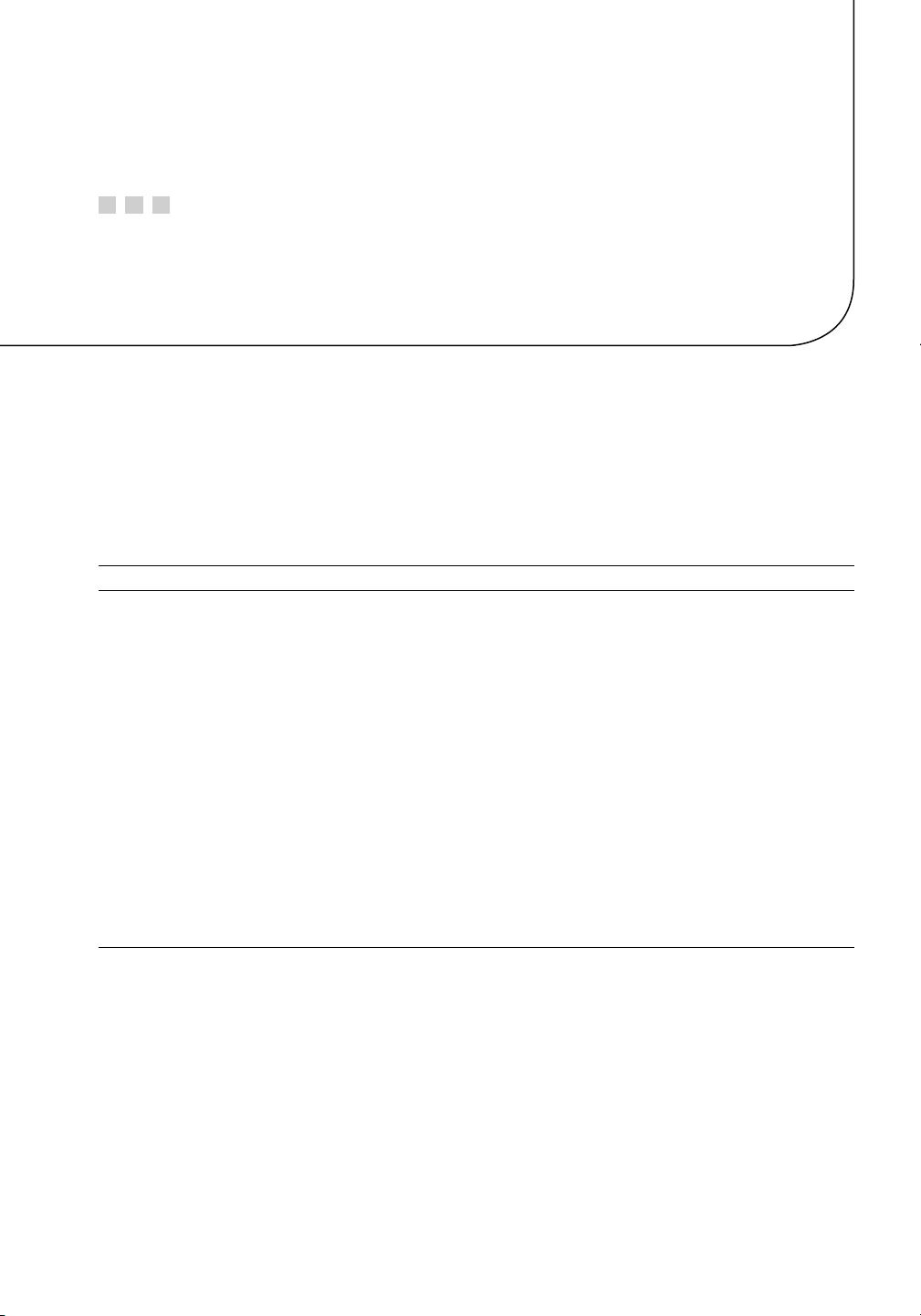
5
© Adam Freeman 2017
A. Freeman, Essential Angular for ASP.NET Core MVC, DOI 10.1007/978-1-4842-2916-3_2
CHAPTER 2
Getting Ready
In this chapter, I explain how to set up the tools and packages required for Angular and ASP.NET Core MVC
development. There are instructions for Windows, Linux, and macOS, which are the three operating systems
that can be used for .NET Core projects. For quick reference, Table2-1 lists the packages and explains their
purpose. Follow the instructions for your preferred operating system to install the tools that are required for
the rest of this book.
Getting Ready on Windows
The following sections describe the setup required for Windows. All the tools used are available for free,
although some are offered in commercial versions with additional features (but these are not needed for the
examples in this book). If you are using Windows, you can use Visual Studio, which is the traditional IDE for
.NET projects, or Visual Studio Code, which offers a lighter-weight alternative.
Table 2-1. The Software Packages Used in This Book
Name Description
Visual Studio Visual Studio is the Windows-only IDE that provides the full-featured development
experience for .NET.
Visual Studio Code Visual Studio Code is a lightweight IDE that can be used on Windows, macOS,
and Linux. It doesn’t provide the full range of features of the Windows-only Visual
Studio product but is well-suited to Angular and ASP.NET Core MVC development.
.NET SDK The .NET Core Software Development Kit includes the .NET runtime for
executing .NET applications and the development tools required to build and test
applications.
Node.js Node.js is used for many client-side development tools, delivered through its
package manager, NPM. It is used to prepare the Angular code for the browser.
Git Git is a revision control system. It is used by some of the NPM packages commonly
used for client-side development.
Docker The Docker package includes the tools required to run applications in containers.
The databases in this book are run inside Docker containers, which makes them
easy to install and manage.
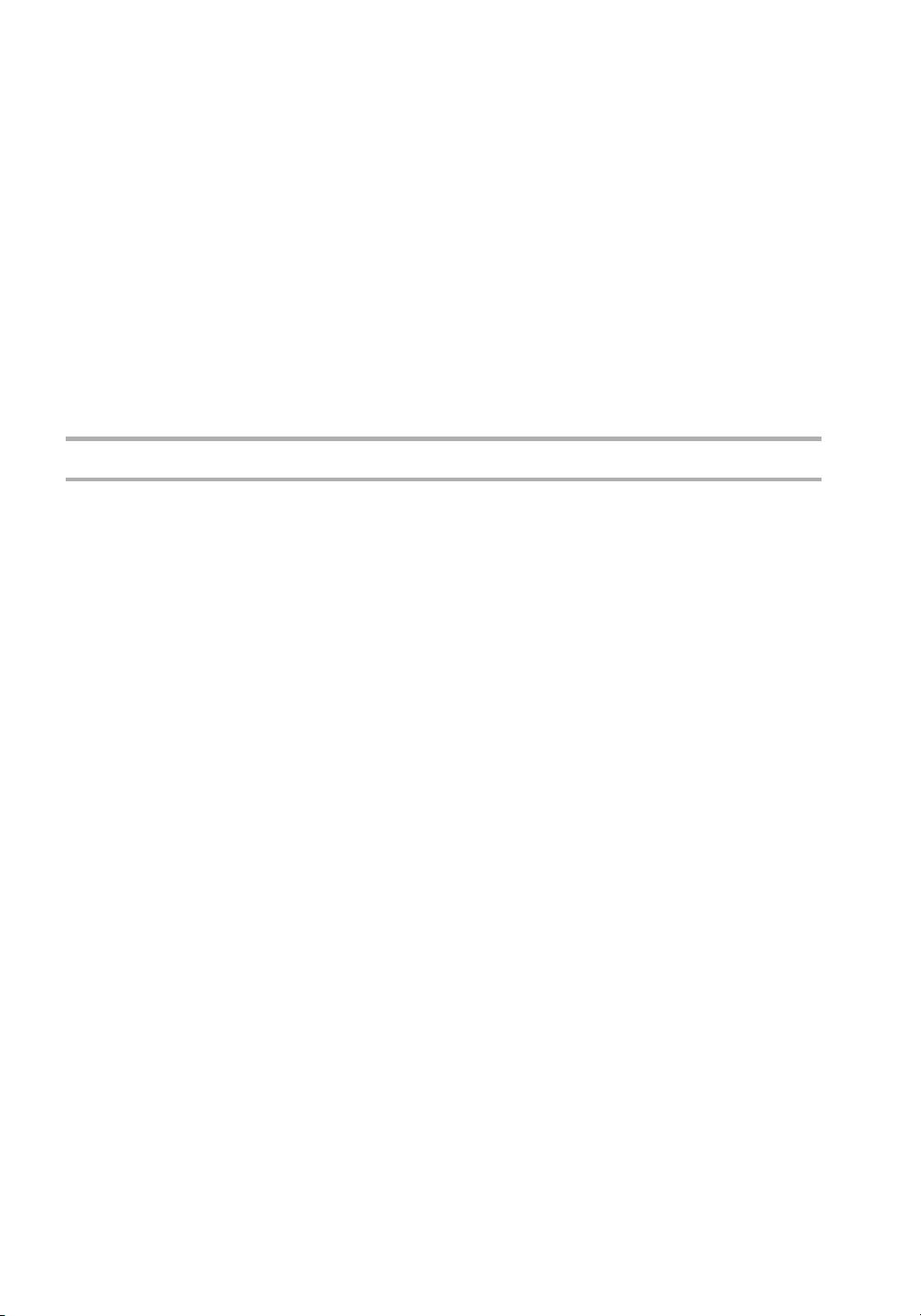
Chapter 2 ■ GettinG ready
6
Installing .NET Core
The .NET Core Software Development Kit (SDK) includes the runtime and development tools needed to
start the development project and perform database operations. To install the .NET Core SDK on Windows,
download the installer from https://download.microsoft.com/download/B/9/F/B9F1AF57-C14A-4670-
9973-CDF47209B5BF/dotnet-dev-win-x64.1.0.4.exe. This URL is for the 64-bit .NET Core SDK, which is
the version that I use throughout this book and which you should install to ensure that you get the expected
results from the examples. Rather than type in a complex URL, you can to go to https://www.microsoft.
com/net/download/core and select the 64-bit installer for the .NET Core SDK. (Microsoft also publishes a
runtime-only installer, but this does not contain the tools that are required for this book.)
Run the installer. Once the install process is complete, open a new PowerShell command prompt and
run the command shown in Listing 2-1 to check that .NET Core is working.
Listing 2-1. Testing .NET Core
dotnet --version
The output from this command will display the latest version of the .NET Core runtime that is installed.
If you have installed only the version specified, this will be 1.0.4.
Installing Node.js
Node.js is a runtime for server-side JavaScript applications and has become a popular platform for
development tools. In this book, Node.js is used by the Angular build tools to compile and prepare the code
that ASP.NET Core MVC will send to the browser.
It is important that you download the same version of Node.js that I use throughout this book. Although
Node.js is relatively stable, there are still breaking API changes from time to time, and they may stop the
examples from working. To install Node.js, download and run the installer from https://nodejs.org/dist/
v6.10.3/node-v6.10.3-x64.msi. This is the installer for version 6.10.3. You may prefer more recent releases
for your projects, but you should stick with the 6.10.3 release for the rest of this book. Run the installer and
ensure that the npm package manager and Add to PATH options are selected, as shown in Figure2-1.
剩余306页未读,继续阅读


yan_ncwu
- 粉丝: 3
- 资源: 8
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

安全验证
文档复制为VIP权益,开通VIP直接复制
