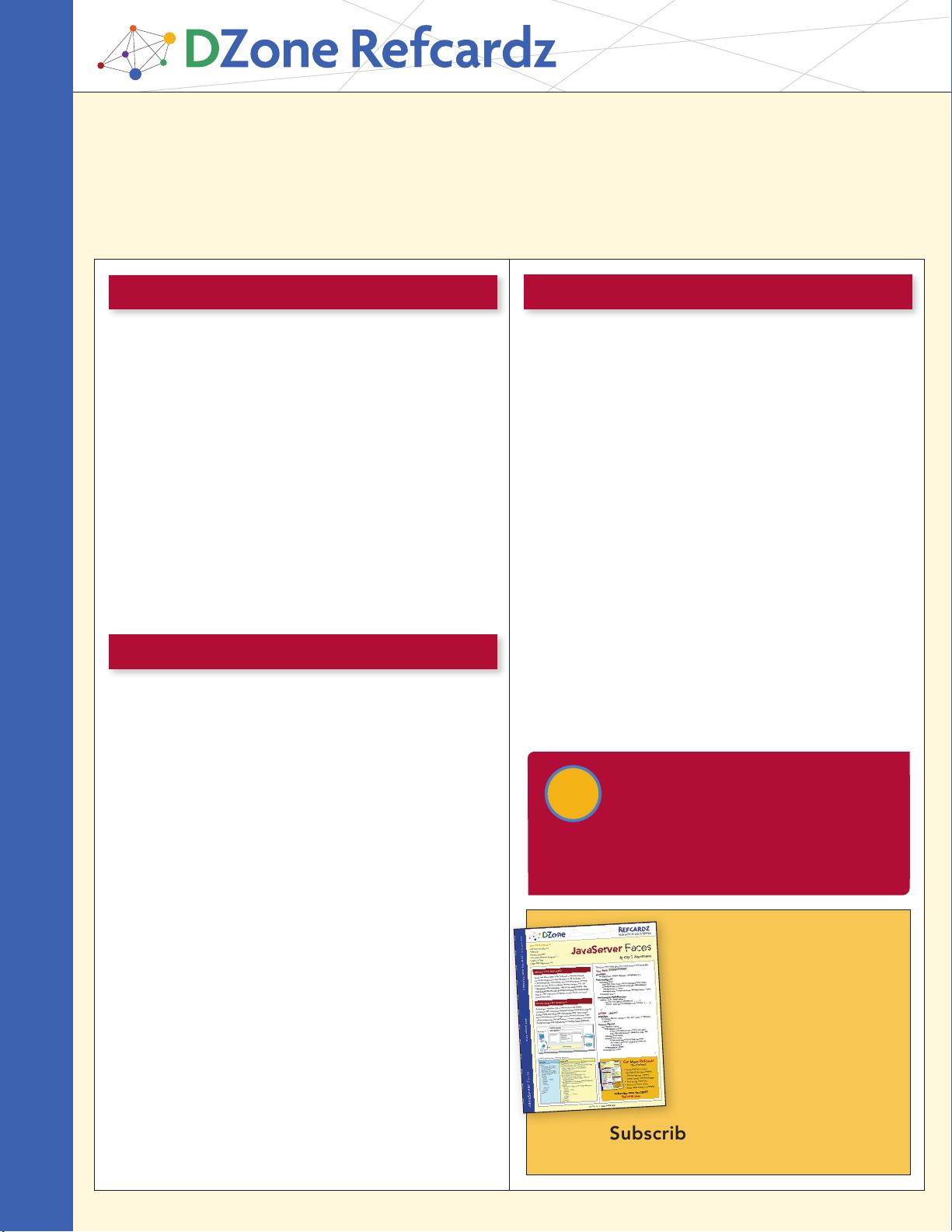
DZone, Inc.
|
www.dzone.com
CONTENTS INCLUDE:
n
Ruby Language Overview
n
Simple Ruby Examples
n
IRB
n
RubyGems
n
Ruby Language Reference Tables
n
Hot Tips and More...
Ruby is an easy-to-learn, dynamic, object-oriented programming
language with dynamic typing and automatic memory
management. While object-oriented at heart, it provides
facilities for procedural and functional programming as well as
extensive support for introspection and meta-programming.
Ruby’s core API, extensive standard library, and thousands of
high-quality external libraries make it suitable for many different
programming tasks in multiple disciplines (key examples being
network programming, Web applications, shell scripts, data
processing, and text manipulation).
Ruby is already installed on Mac OS X and many Linux
distributions. For Windows the easiest way to install everything
necessary is the Ruby Installer (http://rubyinstaller.rubyforge.org).
This refcard provides a quick reference to language elements
and many important API functions for quick lookup.
Ruby is considered to be a “pure” object-oriented language
because almost every concept within Ruby is object-oriented
in some sense. Yukihiro “Matz“ Matsumoto, Ruby’s creator,
wanted to develop a language that operated on the “principle
of least surprise” meaning that code should behave in a non-
confusing manner and be reasonably self-explanatory (beyond
the basic syntax). Matz also wanted Ruby to be a pleasurable
language with which to program, and not make unnecessary
demands upon the programmer.
Ruby is considered a “reflective” language because it’s possible
for a Ruby program to analyze itself (in terms of its make-up),
make adjustments to the way it works, and even overwrite
its own code with other code. It’s also considered to be
“dynamically typed” because you don’t need to specify what
type of objects can be associated with certain variables. Objects
are considered prime in Ruby and whether you’re passing
around a string, a number, a regular expression, or even a class,
you’re just dealing with an object from Ruby’s point of view.
Ruby will seem reasonably familiar to Python and Perl
programmers (and to a lesser extent C# and JavaScript
developers) as Ruby was heavily inspired by Perl in certain areas
(as was Python). Ruby is less similar to languages like C, C++ or
Java because these languages are compiled (not interpreted),
statically typed, and focused on performance rather than
flexibility and conciseness.
Despite being an object-oriented language, it is not necessary
to use explicitly object-oriented syntax within a basic Ruby
program. While everything works on objects (and methods
called upon those objects) behind the scenes, you can write
a program as simply as this:
def b(i)
if i.zero?
0
elsif i == 1
1
else
b(i - 2) + b(i - 1)
end
end
puts b(10)
This script prints to screen the 10th number in the Fibonacci
sequence. It defines a method called
b that returns the
relevant result from a simple
if/elsif/else expression. Note
the use of standard equality syntax (
==), addition (+), subtraction
(
-), and method calling (b(10)), but also note the possibility
of using methods in somewhat idiomatic ways (
i.zero? rather
than
i == 0—though the latter would also work). The use of
i.zero? demonstrates calling a method upon an object (where
i is the object, and zero? is the method).
AbOUT RUby
RUby LANgUAgE OvERvIEw
SImpLE RUby ExAmpLES
Essential Ruby
By Melchior Brislinger and Peter Cooper
Essential Ruby www.dzone.com Get More Refcardz! Visit refcardz.com
#30
n
Authoritative content
n
Designed for developers
n
Written by top experts
n
Latest tools & technologies
n
Hot tips & examples
n
Bonus content online
n
New issue every 1-2 weeks
Subscribe Now for FREE!
Refcardz.com
Get More Refcardz
(
They’re free!
)
Hot
Tip
The main Ruby interpreter is usually invoked by
running “ruby” from the command line. If it is
given a filename as an argument that file will be
run (e.g. ruby myscript.rb). The interpreter has several
other options that are listed in the “Ruby Interpreter Argu-
ments” table in this card’s reference section.