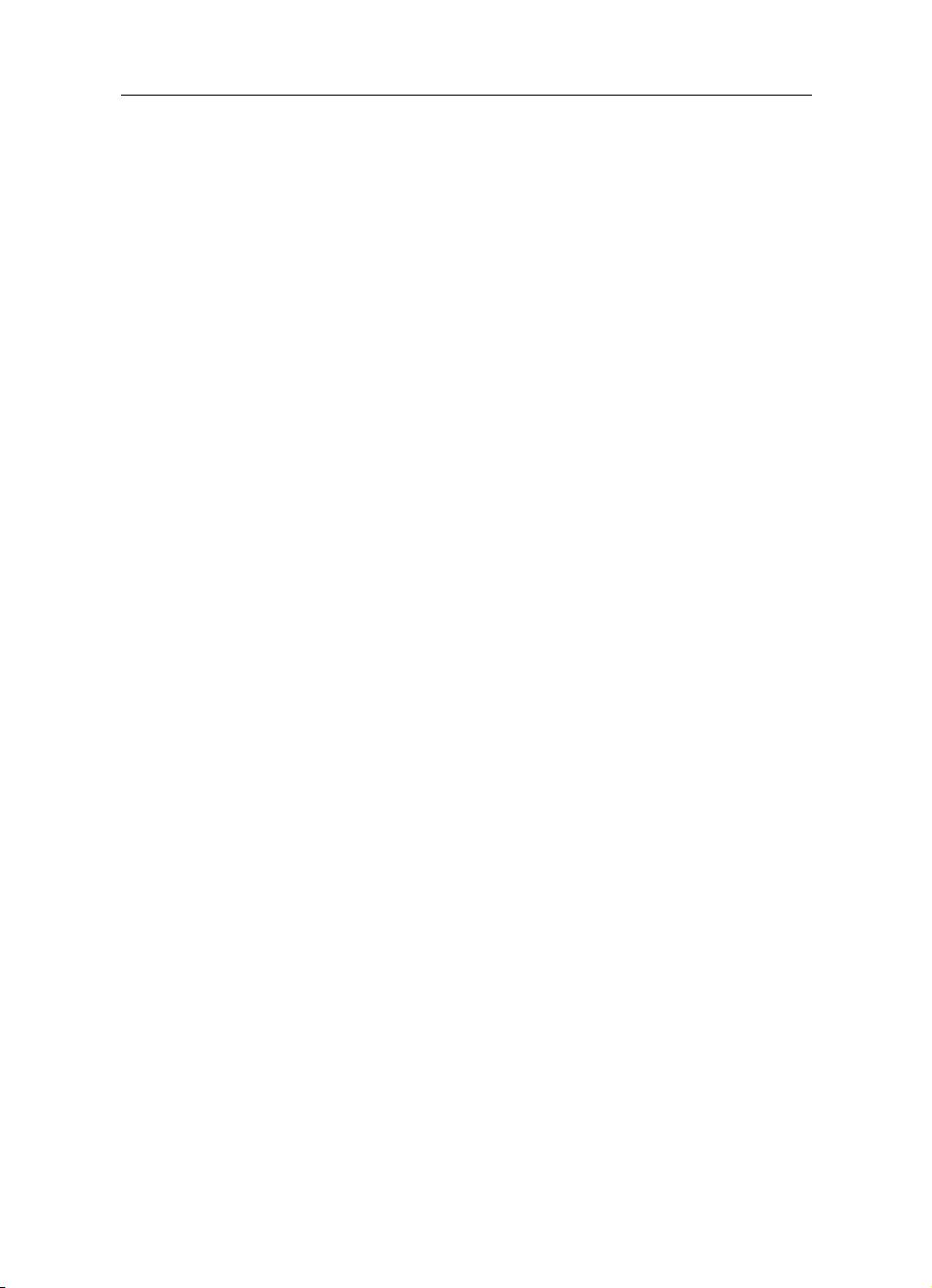
4 Introduction
Part II begins by showing three tiny PyQt GUI applications to give an initial
impression of what PyQt programming is like. It also explains some of the
fundamental concepts involved in GUI programming, including PyQt’s high-
level signals and slots communication mechanism. Chapter 5 shows how to
create dialogs and how to create and lay out widgets (“controls” in Windows-
speak—the graphical elements that make up a user interface such as buttons,
listboxes, and such) in a dialog. Dialogs are central to GUI programming: Most
GUI applications have a single main window, and dozens or scores of dialogs,
so this topic is covered in depth.
After the dialogs chapter comes Chapter 6, which covers main windows,
including menus, toolbars, dock windows, and keyboard shortcuts, as well as
loading and saving application settings. Part II’s final chapters show how to
create dialogs using Qt Designer, Qt’s visual design tool, and how to save data
in binary, text, and XML formats.
Part III gives deeper coverage of some of the topics covered in Part II, and in-
troduces many new topics. Chapter 9 shows how to lay out widgets in quite
sophisticated ways, and how to handle multiple documents. Chapter 10 covers
low-level event handlers, and how to use the clipboard as well as drag and drop,
text, HTML, and binary data. Chapter 11 shows how to modify and subclass
existing widgets, and how to create entirely new widgets from scratch, with
complete control over their appearance and behavior. This chapter also shows
how to do basic graphics. Chapter 12 shows how to use Qt 4.2’s new graphics
view architecture,which is particularly suited to handling large numbers of in-
dependent graphical objects. Qt’s HTML-capable rich text engine is covered in
Chapter 13. This chapter also covers printing both to paper and to PDF files.
Part III concludes with two chapters on model/view programming: Chapter 14
introduces the subject and shows how to use Qt’s built-in views and how to
create custom data models and custom delegates, and Chapter 15 shows how
to use the model/view architecture to perform database programming.
Part IV continues the model/view theme, with coverage of three different
advanced model/view topics in Chapter 16. The first section of Chapter 17
describes the techniques that can be used for providing online help, and the
second section explains how to internationalize an application, including how
to use Qt’s translation tools to create translation files. The Python standard
library provides its own classes for networking and for threading, but in the
last two chapters of Part IV we show how to do networking and threading us-
ing PyQt’s classes.
Appendix A explains where Python, PyQt, and Qt can be obtained, and how to
install them on Windows, Mac OS X, and Linux. PyQt is much easier to learn
if you install it and try out some of the exercises, and if you inspect some of
the example code. Appendix B presents screenshots and brief descriptions
of selected PyQt widgets; this is helpful for those new to GUI programming.
Appendix C presents diagrams of some of PyQt’s key class hierarchies; this