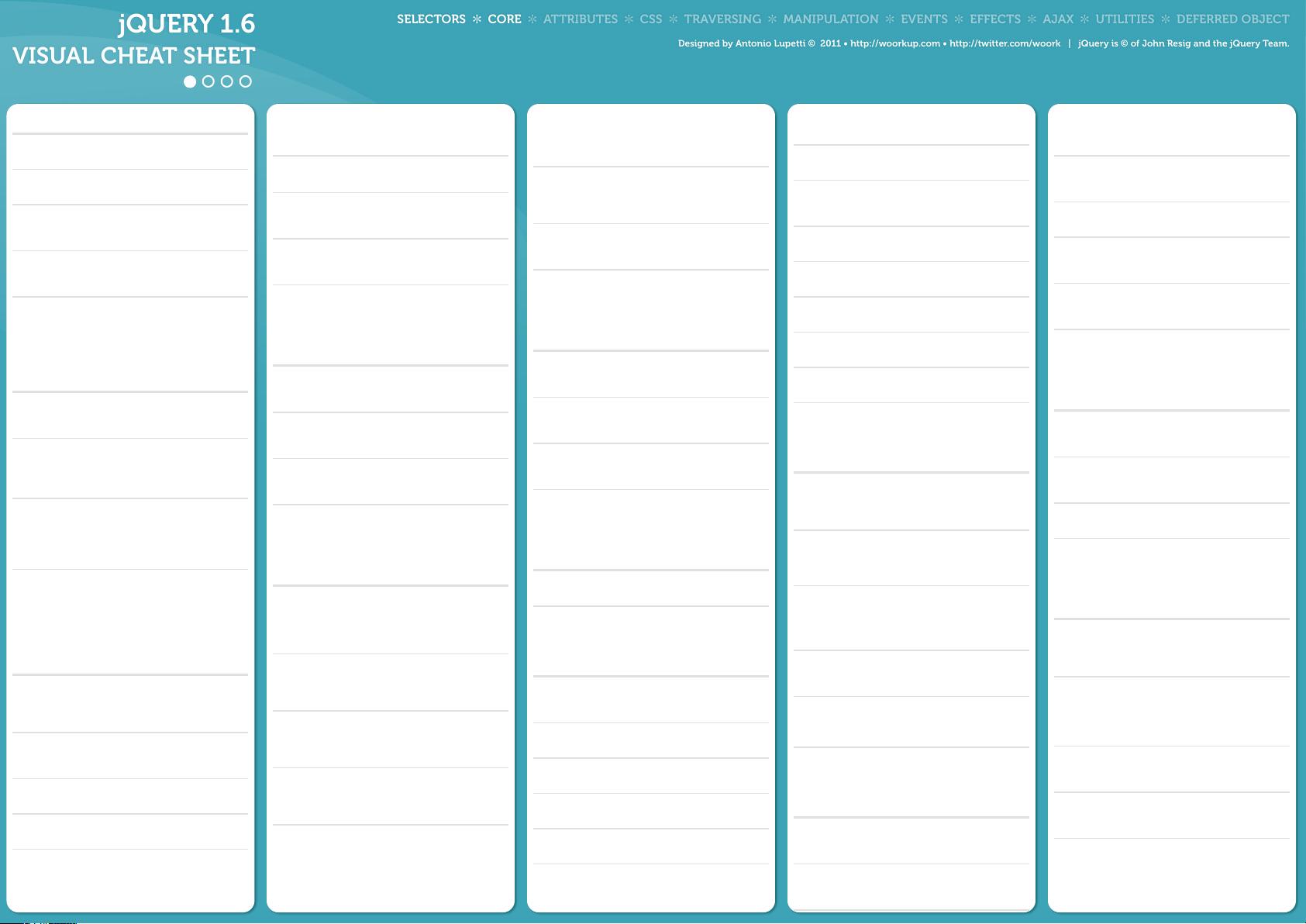
jQUERY 1.6
VISUAL CHEAT SHEET
All Selector (“*”)
Selects all elements.
Class Selector (“.class”)
Matches all elements with the given name.
Element Selector (“element”)
Selects all elements with the given tag
name.
ID Selector (“#id”)
Selects a single element with the given id
attribute.
Multiple Selector (“selector1,
selector2, selectorN”)
Selects the combined results of all the
specified selectors.
❉ SELECTORS / 2. HIERARCHY
❉ SELECTORS / 2. HIERARCHY
Child Selector (“parent > child”)
Selects all direct child elements specified by
"child" of elements specified by "parent".
Descendant Selector (“ancestor
descendant”)
Selects all elements that are descendants of
a given ancestor.
Next Adjacent Selector (“prev +
next”)
Selects all next elements matching "next"
that are immediately preceded by a sibling
"prev".
Next Siblings Selector (“prev ~
siblings”)
Selects all sibling elements that follow after
the "prev" element, have the same parent,
and match the filtering "siblings" selector.
❉ SELECTORS / 3. BASIC FILTER
❉ SELECTORS / 3. BASIC FILTER
:animated Selector
Select all elements that are in the progress
of an animation at the time the selector is
run.
:eq() Selector
Select the element at index n within the
matched set.
:even Selector
Selects even elements, zero-indexed
:first Selector
Selects the first matched element.
:gt() Selector
Select all elements at an index greater than
index within the matched set.
:header Selector
Selects all elements that are headers, like
h1, h2, h3 and so on.
:last Selector
Selects the last matched element.
:lt() Selector
Select all elements at an index less than
index within the matched set.
:not() Selector
Selects all elements that do not match the
given selector.
:odd Selector
Selects odd elements, zero-indexed. See
also even.
❉ SELECTORS / 4. CONTENT FILTER
❉ SELECTORS / 4. CONTENT FILTER
:contains() Selector
Select all elements that contain the
specified text.
:empty Selector
Select all elements that have no children
(including text nodes).
:has() Selector
Selects elements which contain at least one
element that matches the specified selector.
:parent Selector
Select all elements that are the parent of
another element, including text nodes.
❉ SELECTORS / 5. ATTRIBUTE
❉ SELECTORS / 5. ATTRIBUTE
[name|=value]
Selects elements that have the specified
attribute with a value either equal to a
given string or starting with that string
followed by a hyphen (-).
[name*=value]
Selects elements that have the specified
attribute with a value containing the a
given substring.
[name~=value]
Selects elements that have the specified
attribute with a value containing a given
word, delimited by spaces.
[name$=value]
Selects elements that have the specified
attribute with a value ending exactly with
a given string.
[name=value]
Selects elements that have the specified
attribute with a value exactly equal to a
certain value.
[name!=value]
Select elements that either don't have the
specified attribute, or do have the specified
attribute but not with a certain value.
[name^=value]
Selects elements that have the specified
attribute with a value beginning exactly
with a given string.
[name]
Selects elements that have the specified
attribute, with any value.
[name=value][name2=value2]
Matches elements that match all of the
specified attribute filters.
❉ SELECTORS / 6. CHILD FILTER
❉ SELECTORS / 6. CHILD FILTER
:first-child Selector
Selects all elements that are the first child
of their parent.
:last-child Selector
Selects all elements that are the last child of
their parent.
:nth-child Selector
Selects all elements that are the nth-child of
their parent.
:only-child Selector
Selects all elements that are the only child
of their parent.
❉ SELECTORS / 7. VISIBILITY FILTER
❉ SELECTORS / 7. VISIBILITY FILTER
:hidden Selector
Selects all elements that are hidden.
:visible Selector
Selects all elements that are visible.
:button Selector
Selects all button elements and elements of
type button.
:checkbox Selector
Selects all elements of type checkbox.
:checked Selector
Matches all elements that are checked.
:disabled Selector
Selects all elements that are disabled.
:enabled Selector
Selects all elements that are enabled.
:focus selector ★
Selects element if it is currently focused.
:file Selector
Selects all elements of type file.
:image Selector
Selects all elements of type image.
:input Selector
Selects all input, textarea, select and button
elements.
:password Selector
Selects all elements of type password.
:radio Selector
Selects all elements of type radio.
:reset Selector
Selects all elements of type reset.
:selected Selector
Selects all elements that are selected.
:submit Selector
Selects all elements of type submit.
:text Selector
Selects all elements of type text.
❉ CORE / 1. THE jQUERY FUNCTION
❉ CORE / 1. THE jQUERY FUNCTION
jQuery()
Accepts a string containing a CSS selector
which is then used to match a set of
elements.
jQuery.sub()
Creates a new copy of jQ whose properties
and methods can be modified without
affecting the original jQuery object.
jQuery.when()
Provides a way to execute callback functions
based on one or more objects, usually
Deferred objects that represent
asynchronous events.
jQuery.noConflict( )
Relinquish jQuery's control of the $
variable.
jQuery.holdReady() ★
Holds or releases the execution of jQuery's
ready event.
jQuery.extend( object )
Extends the jQuery object itself.
❉ CORE / 2. OBJECT ACCESSORS
❉ CORE / 2. OBJECT ACCESSORS
.context
The DOM node context originally passed to
jQuery().
.each( function(index, Element) )
Iterate over a jQ object, executing a function
for each matched element.
.get( [ index ] )
Retrieve the DOM elements matched by the
jQuery object.
.index()
Search for a given element from among the
matched elements.
.length
The number of elements in the jQuery object.
.selector
A selector representing selector originally
passed to jQuery().
.size()
Return the number of DOM elements matched
by the jQuery object.
.toArray()
Retrieve all the DOM elements contained in the
jQuery set, as an array.
.queue( [ queueName ], newQueue)
Show the queue of functions to be executed on
the matched elements.
.data( obj )
Store arbitrary data associated with the
matched elements.
.removeData( [ name ] )
Remove a previously-stored piece of data.
.dequeue( [ queueName ] )
Execute the next function on the queue for the
matched elements.
❉ CORE / 4. INTEROPERABILITY
❉ CORE / 4. INTEROPERABILITY
jQuery.fn.extend( object )
Extends the jQuery element set to provide new
methods (used to make a typical jQuery
plugin).
jQuery.extend( object )
Extends the jQuery object itself.
.attr( attributeName )
Get the value of an attribute for the first
element in the set of matched elements.
.attr( attributeName, value )
Set one or more attributes for the set of
matched elements.
.removeAttr()
Remove an attribute from each element in the
set of matched elements.
SELECTORS ✼ CORE ✼ ATTRIBUTES ✼ CSS ✼ TRAVERSING ✼ MANIPULATION ✼ EVENTS ✼ EFFECTS ✼ AJAX ✼ UTILITIES ✼ DEFERRED OBJECT
Designed by Antonio Lupetti © 2011 • http://woorkup.com • http://twitter.com/woork | jQuery is © of John Resig and the jQuery Team.
★ = NEW IN jQUERY 1.6 / f(x) = FUNCTION / a = ARRAY / jQ = jQUERY / El = ELEMENT / 0-1 = BOOLEAN / Obj = OBJECT / NUM = NUMBER / Str = STRING