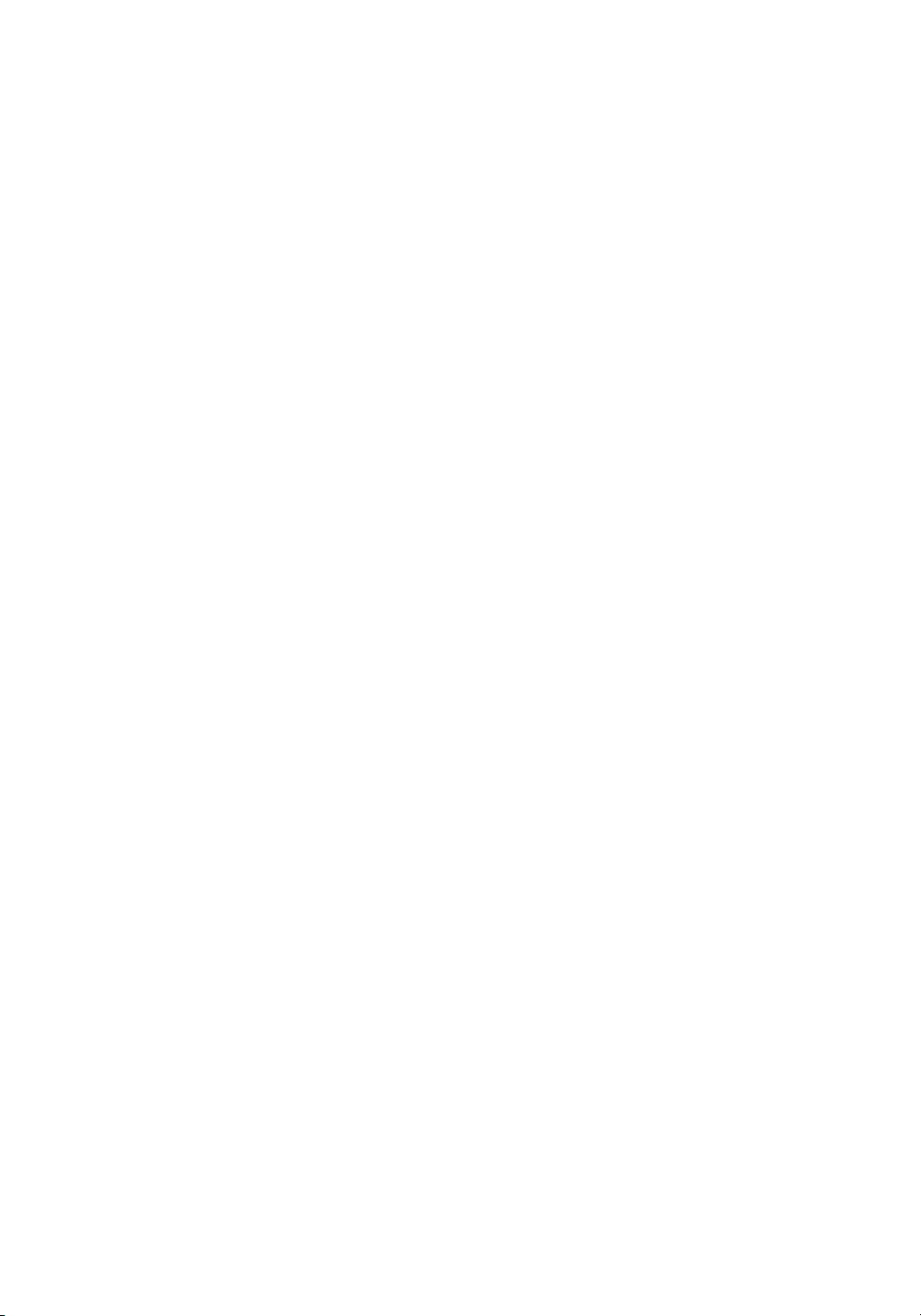
■ INTRODUCTION
xviii
Target Audience
The target audience for this book is software developers including:
• Software developers who are creating programs for Armv8-A platforms and want
to learn how to code performance-enhancing algorithms and functions using the
Armv8-A 32-bit and 64-bit instruction sets
• Software developers who need to learn how to write SIMD functions or accelerate
the performance of existing code using the Armv8-A 32-bit and 64-bit SIMD
instruction sets
• Computer science/engineering students and hobbyists who want or need to gain a
better understanding of the Armv8-A platform and its SIMD architecture
Readers of this book should have previous high-level language programming experience and a basic
understanding of C++. Should you desire to run or modify any of the source code examples published in
this book, a smidgen of familiarity with Linux and the GNU toolchain will be helpful. However, previous
experience with Linux and the GNU toolchain is not necessary to benefit from this book.
Content Overview
The primary objective of this book is to help you learn Armv8-A 32-bit and 64-bit assembly language
programming. The book’s chapters and content are structured to achieve this goal. Here is a brief overview
of what you can expect to learn.
Armv8-32 Architecture Chapter 1 introduces Armv8-A and the AArch32 execution state. It includes
a discussion of fundamental data types, internal architecture, register sets, instruction operands, and
memory addressing modes. Chapters 2, 3, and 4 explain the fundamentals of Armv8-32 assembly language
programming using the A32 instruction set and common programming constructs including arrays and
structures. The source code examples presented in these (and subsequent) chapters are packaged as
working programs, which means that you can run, modify, or otherwise experiment with the code to
enhance your learning experience.
Armv8-32 Floating-Point Programming Chapter 5 examines the floating-point resources of the
AArch32 execution state. It also covers important floating-point programming concepts. Chapter 6 explains
how to perform scalar floating-point arithmetic using single-precision and double-precision values.
Armv8-32 SIMD Programming Chapter 7 describes Armv8-32 SIMD resources including register
sets, data types, and the instruction set. It also includes a short primer on SIMD programming techniques.
Chapters 8 and 9 cover Armv8-32 SIMD programming using packed integer and packed floating-point
operands.
Armv8-64 Architecture Chapter 10 introduces the AArch64 execution state. This chapter expounds
relevant data types, architectural features, general-purpose and SIMD register sets, and memory addressing
modes. Chapters 11 and 12 describe the fundamentals of Armv8-64 assembly language programming using
the A64 instruction set.
Armv8-64 Floating-Point Programming Chapter 13 demonstrates how to perform scalar
floating-point arithmetic using the A64 instruction set.
Armv8-64 SIMD Programming Chapters 14 and 15 delve into the details of the Armv8-64 SIMD
architecture. These chapters contain a variety of programming examples that illustrate how to use the A64
instruction set to carry out calculations using packed integer and packed floating-point operands.
Armv8-64 Advanced Programming Chapter 16 includes source code examples that illustrate how
to perform sophisticated arithmetic calculations using the A64 SIMD instruction set. Chapter 17 outlines
specific coding strategies and techniques that you can use to boost the performance of your Armv8 assembly
language code.