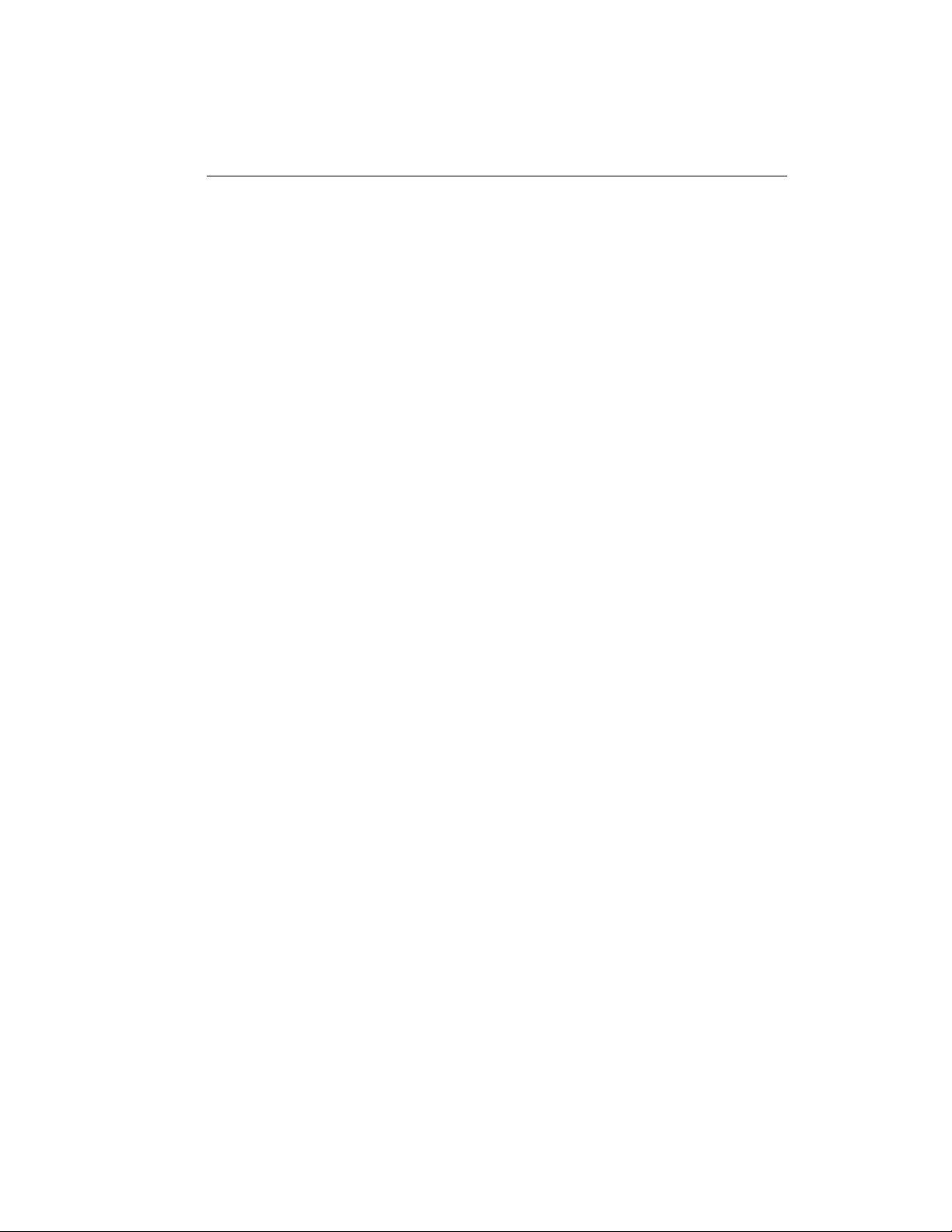
Table of Contents xvii
Mutex Module . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 604
Waiting and Synchronization . . . . . . . . . . . . . . . . . . . . . . . . . 608
Condition Module . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 609
Synchronous Communication . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 612
Synchronization using Communication Events . . . . . . . . . . . . . . . 612
Transmitted Values . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 612
Module Event . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 613
Example: Post Office . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 614
The Components . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 615
Clients and Clerks . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 617
The System . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 618
Exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 619
The Philosophers Disentangled . . . . . . . . . . . . . . . . . . . . . . . . 619
More of the Post Office . . . . . . . . . . . . . . . . . . . . . . . . . . . . 619
Object Producers and Consumers . . . . . . . . . . . . . . . . . . . . . . 619
Summary . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 620
To Learn More . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 621
20 : Distributed Programming 623
The Internet . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 624
The Unix Module and IP Addressing . . . . . . . . . . . . . . . . . . . . 625
Sockets . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 627
Description and Creation . . . . . . . . . . . . . . . . . . . . . . . . . . . 627
Addresses and Connections . . . . . . . . . . . . . . . . . . . . . . . . . . 629
Client-server . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 630
Client-server Action Model . . . . . . . . . . . . . . . . . . . . . . . . . . 630
Client-server Programming . . . . . . . . . . . . . . . . . . . . . . . . . . 631
Code for the Server . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 632
Testing with telnet . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 634
The Client Code . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 635
Client-server Programming with Lightweight Processes . . . . . . . . . . 639
Multi-tier Client-server Programming . . . . . . . . . . . . . . . . . . . . 642
Some Remarks on the Client-server Programs . . . . . . . . . . . . . . . 642
Communication Protocols . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 643
Text Protocol . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 644
Protocols with Acknowledgement and Time Limits . . . . . . . . . . . . 646
Transmitting Values in their Internal Representation . . . . . . . . . . . 646
Interoperating with Different Languages . . . . . . . . . . . . . . . . . . 647
Exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 647
Service: Clock . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 648
A Network Coffee Machine . . . . . . . . . . . . . . . . . . . . . . . . . . 648
Summary . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 649
To Learn More . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 649
21 : Applications 651